Answer
Approach
You can define a single function signature using TypeScript's union types for both e
(event) and value
parameters. TypeScript will infer the specific types based on how you call the function.
type MyFunc = (
e: Event | React.SyntheticEvent<Element, Event>,
value: number | number[]
) => void;
Example Usages
Here are some examples of how you can call this MyFunc
:
const handler1: MyFunc = (e: Event, value: number) => {
you may handle event and number value
};
const handler2: MyFunc = (e: Event, value: number[]) => {
you may handle event and number array value
};
const handler3: MyFunc = (e: React.SyntheticEvent<Element, Event>, value: number) => {
// Handle synthetic event and number value
};
const handler4: MyFunc = (e: React.SyntheticEvent<Element, Event>, value: number[]) => {
// Handle synthetic event and number array value
};
for exemple I used it in my material UI code .
import Slider from '@mui/material/Slider';
const MyComponent = () => {
const handleChange: MyFunc = (e, value) => {
// Handle slider change here
};
return (
<Slider
value={50}
onChange={handleChange}
// other props
/>
);
};
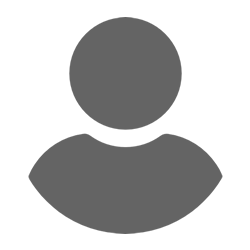
@felix-domingos
How could I have optional/flexible TypeScript typed in a function signature?
I have the following example function signature:
(e: Event | React.SyntheticEvent<Element, Event>, value: number | number[]) => void
But what I really want is to have the ability to accept functions that have any combination of:
(e: Event, value: number) => void
(e: Event, value: number[]) => void
(e: React.SyntheticEvent<Element, Event>, value: number) => void
(e: React.SyntheticEvent<Element, Event>, value: number[]) => void
But TypeScript is really annoying and would only accept the exact same signature... What is the correct way to make the TypeScript function signature a little bit more "acceptable", "flexible" or "smarter"?
I kinda don't want to have the following though:
type MyFuncSig =
(e: Event, value: number) => void |
(e: Event, value: number[]) => void |
(e: React.SyntheticEvent<Element, Event>, value: number) => void |
(e: React.SyntheticEvent<Element, Event>, value: number[]) => void;
Edit:
The original function signature in question, is actually from Material UI's Slider (see: onChange
and onChangeCommitted
)
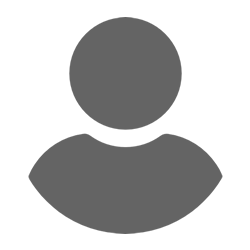
@