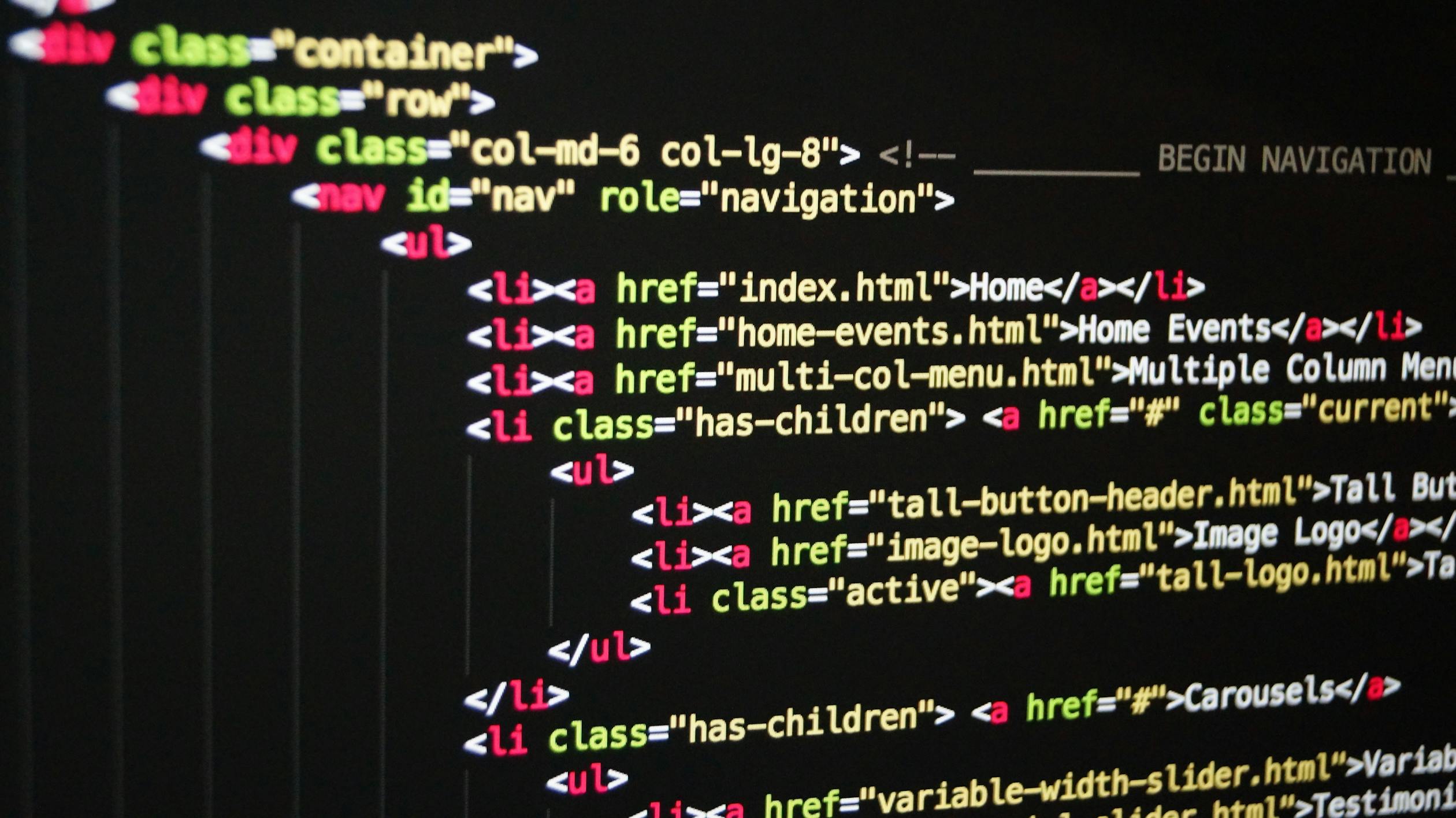
October 05, 2023
How old am I? Calculate the difference between two dates in Python
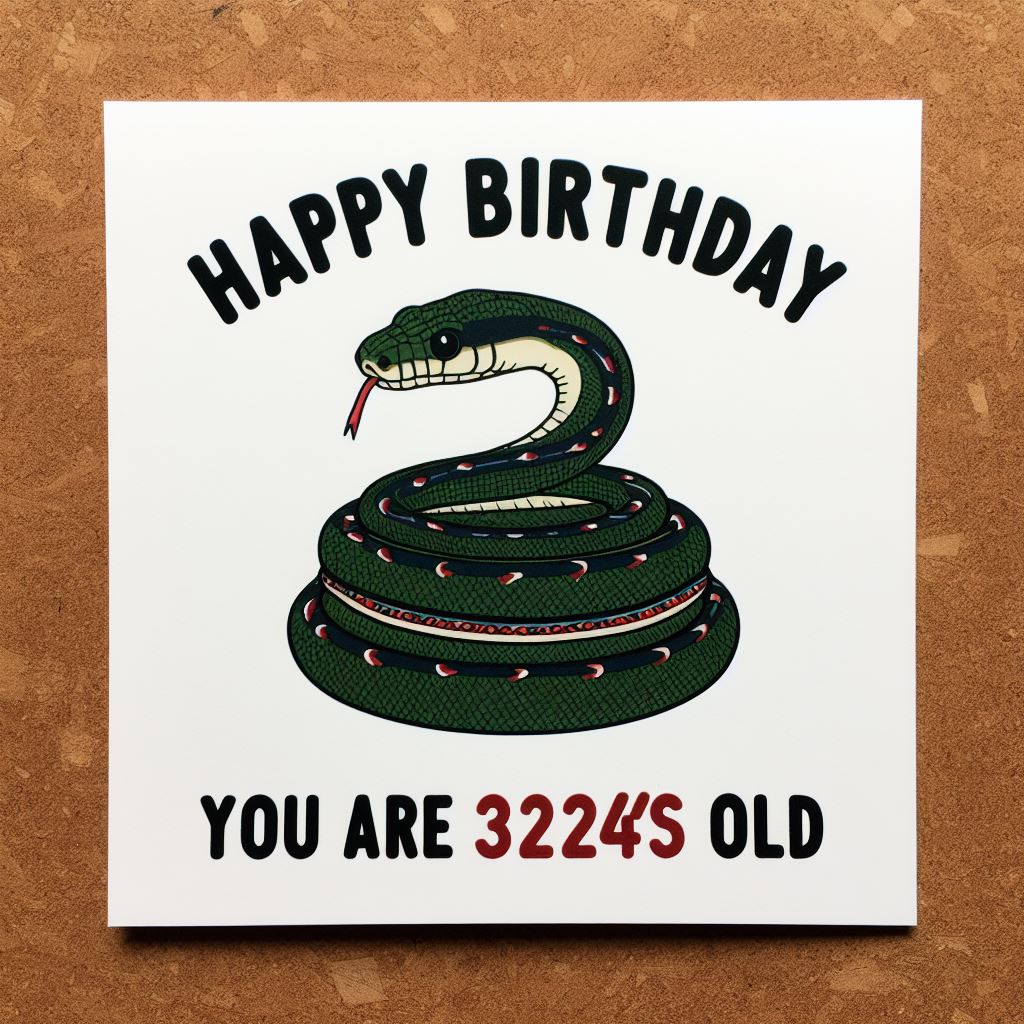
Purpose
This document will introduce the concepts of Python dates and command-line arguments.
Audience
This document will be useful for developers and learners new to the Python programming language.
Prerequisites
- Python interpreter installed on your computer - you can learn more about installing Python on your machine by checking out the pre-requisites in my article https://onlycoders.net/article/29/hello-python-a-beginners-tutorial-to-the-famous-programming-language-29
- text editor or integrated development environment (IDE)
Introduction
I have a lot of nieces and nephews, so many that birthdays can be tricky - especially when I forget their ages. Nothing screams 'bad uncle' like a birthday card with the wrong number on it! It happens to us all, maybe me more than others.
But with every challenge, comes opportunity. When learning a programming language, or programming in general, I find it is useful to write lots of small programs, each with individual purposes across a variety of domains. Mental maths is great, but the stakes are too high here. I decided to write a utility that will take a date - for example for nephew's date of birth - and tell me the number of years, months, and days since that date.
In this tutorial, we will create a program that does this, and I invite you to follow along!
Step-by-step
Step 1 - Create a Python program and import modules
- With your favourite editor or IDE at the ready, create a new file and call it diff_dates.py.
- add this as the first two lines:
from datetime import date
import sys
Python's 'datetime' module provides a set of classes for working with dates and times. The above lines import the datetime and sys module into our program, ready to be pressed into service.
We need to tell Python what date we are interested in. There are many ways of doing this, but we will use a command line input. The 'sys' module includes several standard interpreter functions, including the ability to access whatever the user types on the command line.
Step 2 - Add a 'main' function
Many programming languages have a specifically-named function that the interpreter executes automatically when the program starts. Not so for Python. Python simply executes each line one-by-one.
NOTE: A function is a self-contained piece of code accomplishes a specific task. Think of a function as a machine with raw materials feeding in on a conveyer belt and completed products coming out.
Let's add this to our program:
def main():
print('Hello')
main()
What have we done here? On line 1, we define a function called 'main' - it could have been any name, but I used 'main'. All this function does for now is print the word 'Hello' on the terminal. It requires no inputs, so there is nothing in between the parentheses.
On line 3, we invoke the function - main(). This actually tells Python to execute that function.
- Test your program by:
- opening a terminal or command line prompt
- navigating to the directory where you created diff_dates.py
- typing: 'python diff_dates.py'
If all goes well, you should see the word 'Hello', printed on the terminal. Great, we have the basis for what we're doing, let's move on.
Step 3 - Create a function that takes command-line arguments
In our previous step we ran our Python program. 'diff_dates.py' is our program name. Whatever we type after the program name is called an argument. Arguments are the parameters, the options, the raw materials we pass into our program.
Our program will take three arguments representing a date: year (in YYYY) format, month (MM) and day (DD). So we would like our users to run our program as shown in this example:
python diff_dates.py 2009 10 01
Python offers a variable called 'sys.argv' that holds all arguments passed into our program. argv is a list, a concept not covered in this tutorial. For now, just know that we access a lists entries with a number in square brackets starting from one. So sys.argv[1] will be the first , sys.argv[2] will be the second argument and so on.
- Let's make function that takes the first three command line arguments and prints them out. Add these lines to your program above the main function.
def get_command_line_argument_date():
print(sys.argv[1], sys.argv[2], sys.argv[3])
- Now, let's replace the 'print('Hello')' statement in our main function, with a line that instead triggers our new 'get_command_line_argument_date' function.
def main():
get_command_line_argument_date()
main()
Our complete diff_dates.py file should now look like:
from datetime import date
import sys
def get_command_line_argument_date():
print(sys.argv[1], sys.argv[2], sys.argv[3])
def main():
get_command_line_argument_date()
main()
- Run your program as follows: 'python diff_dates.py 2010 11 12'
You should have this output:
2010 11 12
Step 4 - Convert the arguments to a date
Let's convert our three arguments to a Python date!
- In the get_command_line_argument_date function, replace the print line with this one:
print(date(int(sys.argv[1]), int(sys.argv[2]), int(sys.argv[3])))
We have created a new date object using our three arguments. We then print out that date object.
NOTE: we've wrapped each sys.argv argument in a call to the int() function. This is a useful little tool in Python that transforms (casts) the input argument into a number. Keep in mind that the input arguments are technically of type string - we need to convert them to integers before we can use them to make date objects.
- Run your program again - python diff_dates.py 2010 11 12
You should have this output - it looks more like a date now.
2010-11-12
- change the print statement to a return. Remember, functions are like machines that take raw materials and give back something new. We need our function to return what is has produced.
def get_command_line_argument_date():
return (date(int(sys.argv[1]), int(sys.argv[2]), int(sys.argv[3])))
Step 5 - Calculate the number of days
Now that we have a date object, we can do useful stuff with it like working our the number of days between it and today's date.
- Replace the contents of main() with the lines below.
def main():
date1 = get_command_line_argument_date()
date2 = date.today()
What we are doing here is creating two date objects. date1 is a date built on from the inputs that the user provides, and date2 is today's date.
Now that we have some date in the past, and today's date, we can work out the difference.
- Create a new function called 'diff_dates'
def diff_dates(date1, date2):
return abs(date2-date1).days
The above code does the magic for us. The function expects two dates and subtracts one from the other. It then returns the number of days that have elapsed between them!
- Edit main() function again to call our diff_dates function.
def main():
date1 = get_command_line_argument_date()
date2 = date.today()
number_of_days = diff_dates(date2, date1)
print(number_of_days)
The output is, depending on the date you entered and today's date, something like:
4701
Wow, we've calculated a number of days between two dates!
Step 6 - Calculate the age in years
I can't find birthday cards that measure age in days. But as impressive a milestone as 4701 is, we need to convert this number to years. That's an easier calculation, we simply divide the number of days by 365.
Here is the completed code:
from datetime import date
import sys
def diff_dates(date1, date2):
return abs(date2-date1).days
def get_command_line_argument_date():
return (date(int(sys.argv[1]), int(sys.argv[2]), int(sys.argv[3])))
def main():
date1 = get_command_line_argument_date()
date2 = date.today()
number_of_days = diff_dates(date2, date1)
print("You are : "+ str(number_of_days / 365) + " old!")
main()
396 views