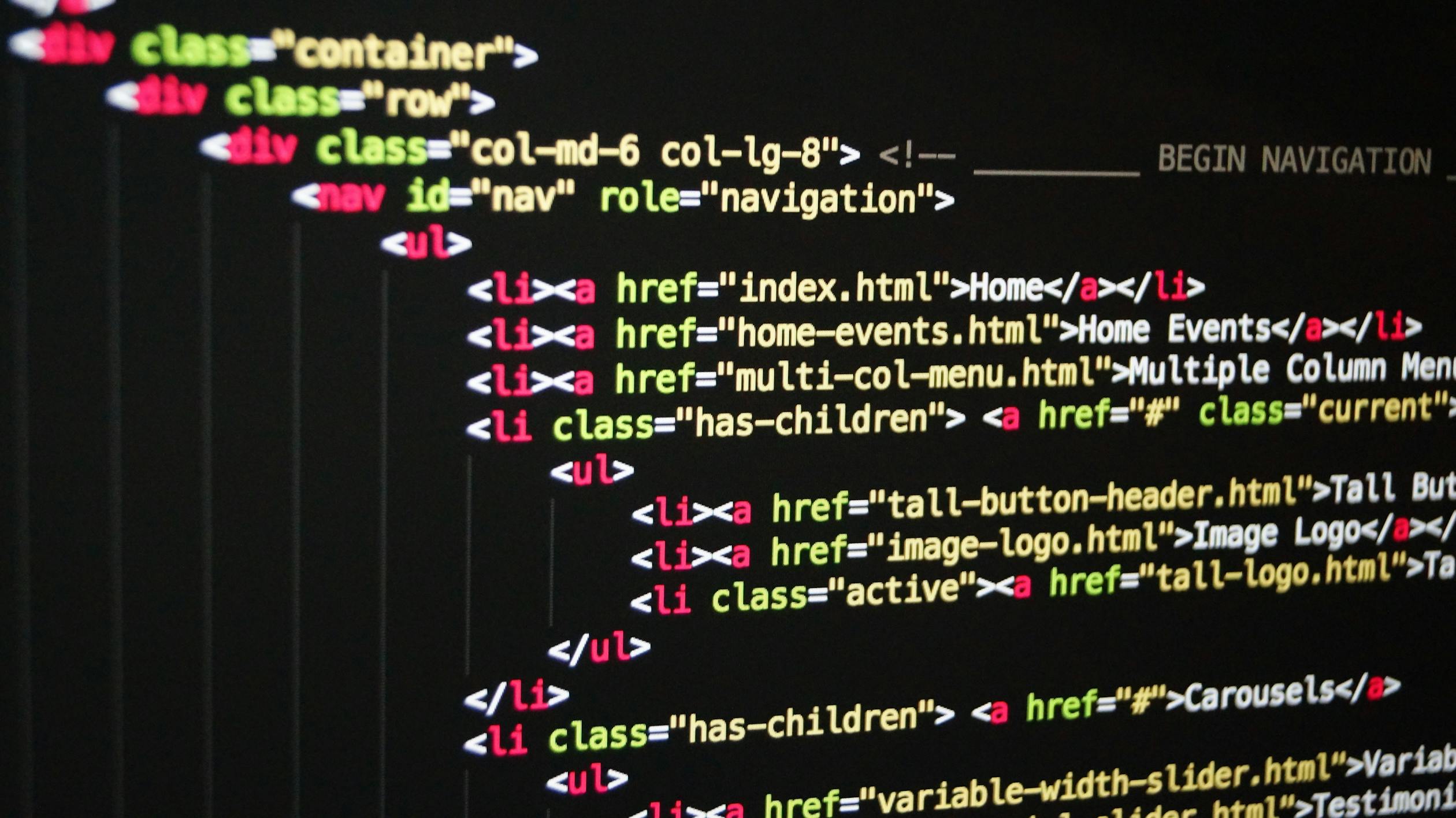
September 28, 2023
Hello, Python! A beginner's tutorial to the famous programming language
Purpose
This document will introduce beginners to the Python programming language via a hands-on tutorial.
Audience
From newbies to code-hardened veterans, this document will appeal to anyone looking to learn this amazing coding language.
Prerequisites
Before embarking on this journey, you will need to install Python on your device.
This document works with Python 3, (Python 2 is SO 2007!). If you don't already have Python 3 installed, you can download it from the official Python website: https://www.python.org/downloads/
Python is ubiquitous, it runs on just about everything. Mac, Windows, Linux - even Raspberry Pi, cloud servers, there is a Python for everyone!
If you don't already have Python 3 installed, you can download it from the official Python website: https://www.python.org/downloads/
Introduction
Why do we love Python?
- it is as easy to learn as it is powerful to use
- everyone from beginners to experts use it
- it's one of the most popular programming languages in the world
- it's well-established with a beefy set of frameworks, libraries, and tools for just about any use case
Python is often described as a "beginner-friendly" language, and for good reason. It has a simple syntax that's easy to read and write. Plus, there are tons of resources available online and in libraries to help you learn Python.
While Python is simple, easy to read and understand, its applications are endless, including:
- Web applications
- Data science and machine learning applications
- Mobile applications
- Desktop applications
- Scripting and automation
Step-by-step
Okay, enough preamble. The best way to learn is to do, so let's do it!
Step 1- Using the command line
Python in an interpreted language. That's a fancy way of saying that Python reads the source code line by line and executes each line directly. This is great because it means we can type individual lines of code one by one and watch them execute in front of your eyes.
- Open a command prompt. If you are using Windows, open the command prompt program. If you are using Mac, open Terminal. If you are running any flavour of Linux, you probably don't need instructions.
- Next, type 'python'.
You should now be on a prompt with three arrows - ">>>".
- Let's say hello! Type 'print("Hello Python!")' and press enter
Something just happened. The interpreter read our command and executed - printing "Hello Python!" on the terminal. Great. But in reality you the programmer will generally not be present at the command line to input commands one at a time. Let's take it to the next level with a Python file.
- Type exit() and press enter. You can also close your command prompt or terminal now.
Step 2 - Create a Python file
Note on tooling: This tutorial will assume you do not have an IDE like JetBrains' PyCharm or Microsoft's Visual Studio Code installed and will employ simple text editors.
- Create a .py file.
Windows users may with to use the charming and much-loved Notepad. Mac users may prefer TextEdit. Hardcore hackers may use a terminal-based tool like emacs, nano, for the true craftspeople, vi. Open your weapon of choice and save (Windows users, CTRL+S), Mac (CMD+S). - Name your .py file
Python files have an extension of '.py'. Let's call our file 'hello.py'. I am saving my Python program files in a directory called 'scripts'. Windows Notepad example below:
- Click Save
Step 3 - Add some code
- type 'print("Hello Python")
Just as we did on the Python command line, type in the command to print our phrase.
- Save the file
Voila, we have created a (simple) Python program in file.
Step 4 - Execute!
- Keep your text editor open and open or return to your command line. Navigate to the folder where you saved your hello.py file.
NOTE: Navigation via the command line can be tricky if you aren't accustom to it. Here are some examples:
Windows
C:\Users\User1>cd Documents\scripts
Mac + Linux
user1@mydevice:~$ cd Documents/scripts
- Once you are in the correct directory, type: "python hello.py"
If all goes well, your program should print the greeting. e.g.,
Microsoft Windows [Version 10.0.22621.2283]
(c) Microsoft Corporation. All rights reserved.
C:\Users\User1>cd Documents\scripts
C:\Users\User1\Documents\scripts>python hello.py
Hello Python
C:\Users\User1\Documents\scripts>
Step 5 - Let's expand
Python programs can do a lot more than print a single line.
- To demonstrate this, return to your text editor and add more lines to hello.py
print('Hello Python')
print('Hello World!')
print('Hola Mundo')
print('Hallo Welt')
- Save your file, return to the command line and execute the command again.
C:\Users\User1\Documents\scripts>python hello.py
Hello Python
Hello World!
Hola Mundo
Hallo Welt
And there we have it. The Python interpreter has sequentially executed each command in our program file.
Next steps
This rather rudimentary example may seem galaxies away from analysing complex data, animating a robot, or training a machine learning algorithm. But this is a start, a mere surface scratch for Python's deep and broad capabilities.
I am super-excited to show you more! In the mean time, play around with your first program. Add more print statements, or change the text. Try creating other .py files with their own print statements. Practice makes permanent!
634 views