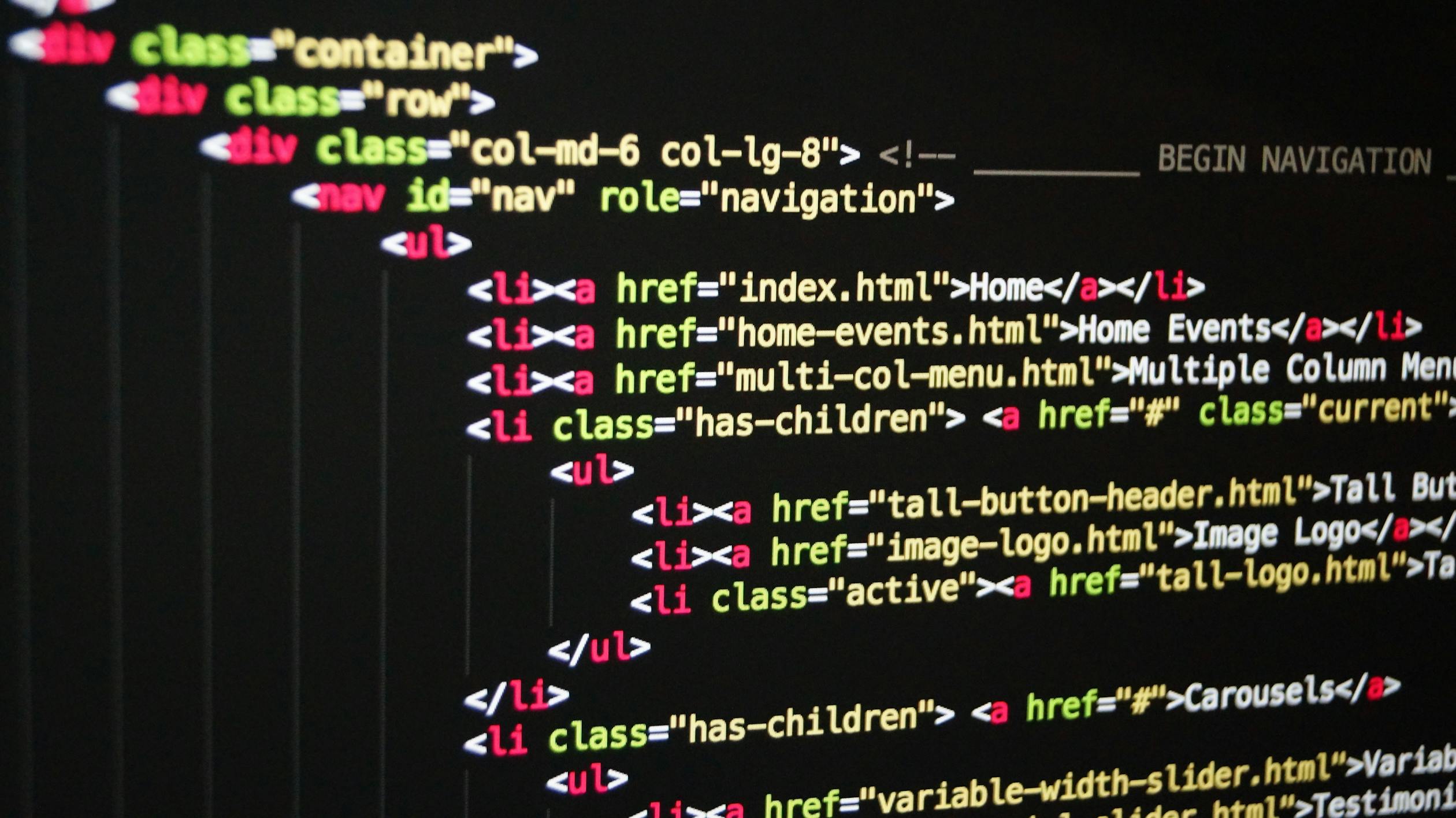
In the world of JavaScript development, debugging plays a crucial role in fine-tuning our code. It helps us identify and fix errors and provides us insights into the behavior of our applications. When it comes to debugging, developers typically rely on console.log() to print values directly onto the console for observation. However, what if we could present our data in a more structured and visually appealing manner? This is where console.table() comes into play.
Understanding console.table():
Introduced in JavaScript version ES6, console.table() is a powerful method that allows developers to present their data as a table directly on the browser's console. It provides an organized view of the data, making it easier to interpret and analyze. While console.log() simply outputs values as text, console.table() takes an array or an object as its parameter and converts it into a table structure.
console.table()
Benefits and Use Cases:
Clear Data Presentation: One of the significant advantages of console.table() is its ability to display complex data structures in a formatted way. Arrays, objects, and even JSON can be presented as tables, making it easier to comprehend their contents. This becomes especially helpful when dealing with large datasets that are difficult to analyze through traditional console.log() outputs.
Improved Object Visualization:
When using console.log() to display an object, the output may not provide a comprehensive view of its properties and their values. On the other hand, console.table() provides a dedicated column for each object property, eliminating ambiguity and providing a holistic representation of complex object structures.
Console.table VS Console.log - Object
const myObj = {
name: 'Giordano',
surname: 'Maserati',
newsletter: true,
}
console.log(myObj)
console.table(myObj)
Output
Efficient Data Comparison:
When working with arrays or objects that need to be compared for differences or similarities, console.table() proves to be a valuable tool. By displaying two or more data sets side by side, developers can easily spot variations between them. This makes debugging and verification more efficient, ensuring the accuracy of the code.
Implementation Example:
Let's consider a simple example to understand how console.table() can be implemented:
Console.table VS Console.log - Array
const colours = ["green", "red", "blue"]
console.log(colours)
console.table(colours)
Output
In this example, we have an array of colours with their respective properties. By passing the colours array to console.table(), we obtain a neatly organized, user-friendly table that showcases the colour data.
Intuitive Sorting and Filtering:
The console.table() method provides an interactive table on the console, allowing you to sort the data in ascending or descending order by simply clicking on the relevant table headers. It also offers the option to filter rows based on specific criteria, providing a dynamic and simplified debugging experience.
Conclusion:
console.table() is an indispensable debugging tool that enhances the interactive nature of JavaScript development. By utilizing its features to their full potential, developers can better understand complex data structures, compare and validate data sets, and improve overall debugging efficiency. Next time you find yourself in a debugging session, remember to leverage the power of console.table() to simplify and streamline your debugging process.
525 views