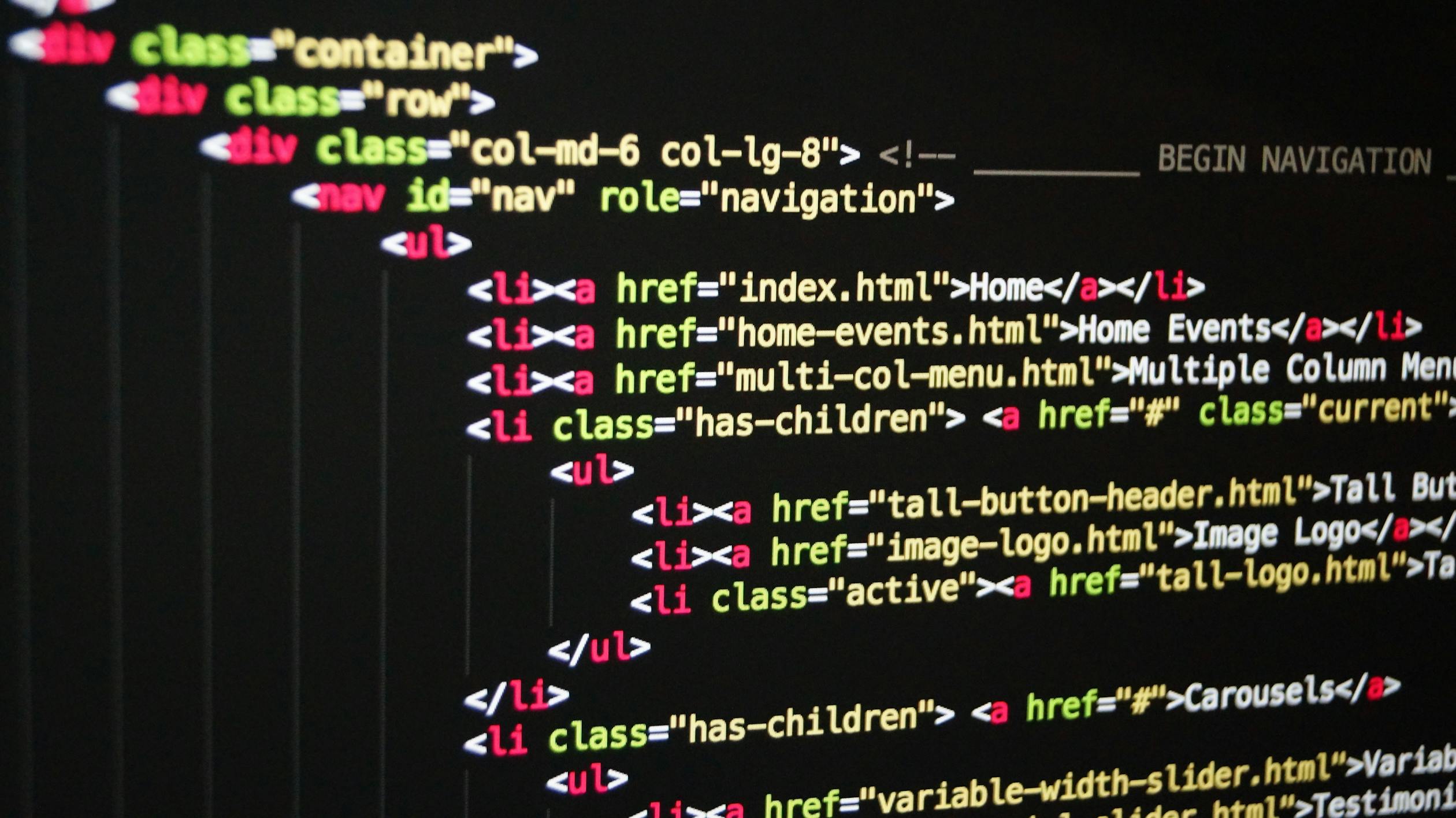
In most useful programs, we must instruct the processor to do something under a certain condition, and perhaps something else under different circumstances. This is called "conditional execution" and most programming languages express this with "if...else" statements. Let's meet Python's if, elif, and else statements.
Audience
I have written this article with beginners and new learners in mind.
Prerequisites
- a Python interpreter and IDE or text editor - check out my getting started tutorial
Introduction
"if" statements are part of the family of commands known as "control structures". They help control what a program does, in what order, and under which conditions. "if" statements compare constants or variables and return either "True" or "False" depending on the comparison's outcome. e.g., 2 > 4 will result in False. 4 > 2 will result in True.
Step-by-step
Simple comparisons
- create a file called condition.py and add the code below
print("4")
Under all circumstances, Python will print "4". Let's add a condition.
"if" statements test whether or not something is true - e.g., does 2 plus 2 equal 4? In most universes it does, so Python will always say "True" when asked to test that condition.
- change your code as below and run condition.py
if (2 + 2) == 4:
print("True")
else:
print("False")
True
NOTE: Python's comparison operators are:
Symbol | Operator |
---|---|
== | equals |
> | greater than |
< | less than |
>= | greater than or equal |
<= | less than or equal |
!= | not equal |
- let's try a false outcome, change your code as follows and run your program
if (2 + 2) == 5:
print("True")
else:
print("False")
False
- let's play with more comparisons
if (2 + 2) == 5:
print("True")
else:
print("False")
if 3 > 2:
print("Three is bigger")
else:
print("Something is wrong with the universe")
if (2 < 4):
print("Two is smaller")
else:
print("Something is wrong with the universe")
if (4>=4):
print("Four is equal to four")
if (50<=50):
print("Fifty is equal to fifty")
False
Three is bigger
Two is smaller
Four is equal to four
Fifty is equal to fifty
Less simple comparisons
You can perform multiple comparisons with the 'elif' clause. 'else' is the catch-all.
x = 3
if (x == 4):
print('x is 4')
elif (x == 2):
print('x is 2')
elif (x == 3):
print('x is 3')
else:
print('x is none of the above')
Short-hand 'if' statements
- Python allows you to write a single condition on one line
x = 41
y = 27
if x > y: print("x is greater than y")
x is greater than y
- you can even squeeze an 'else' into one line:
x = 4
y = 27
print("x is greater than y") if x > y else print("x is not greater than y")
x is not greater than y
NOTE: the above example is known as a 'ternary' operator which means having three parts.
Conclusion
This has been a simple introduction to a powerful concept not only in Python but in programming. You should now have another trusty tool in your kit to accompany you on your coding journey.
284 views