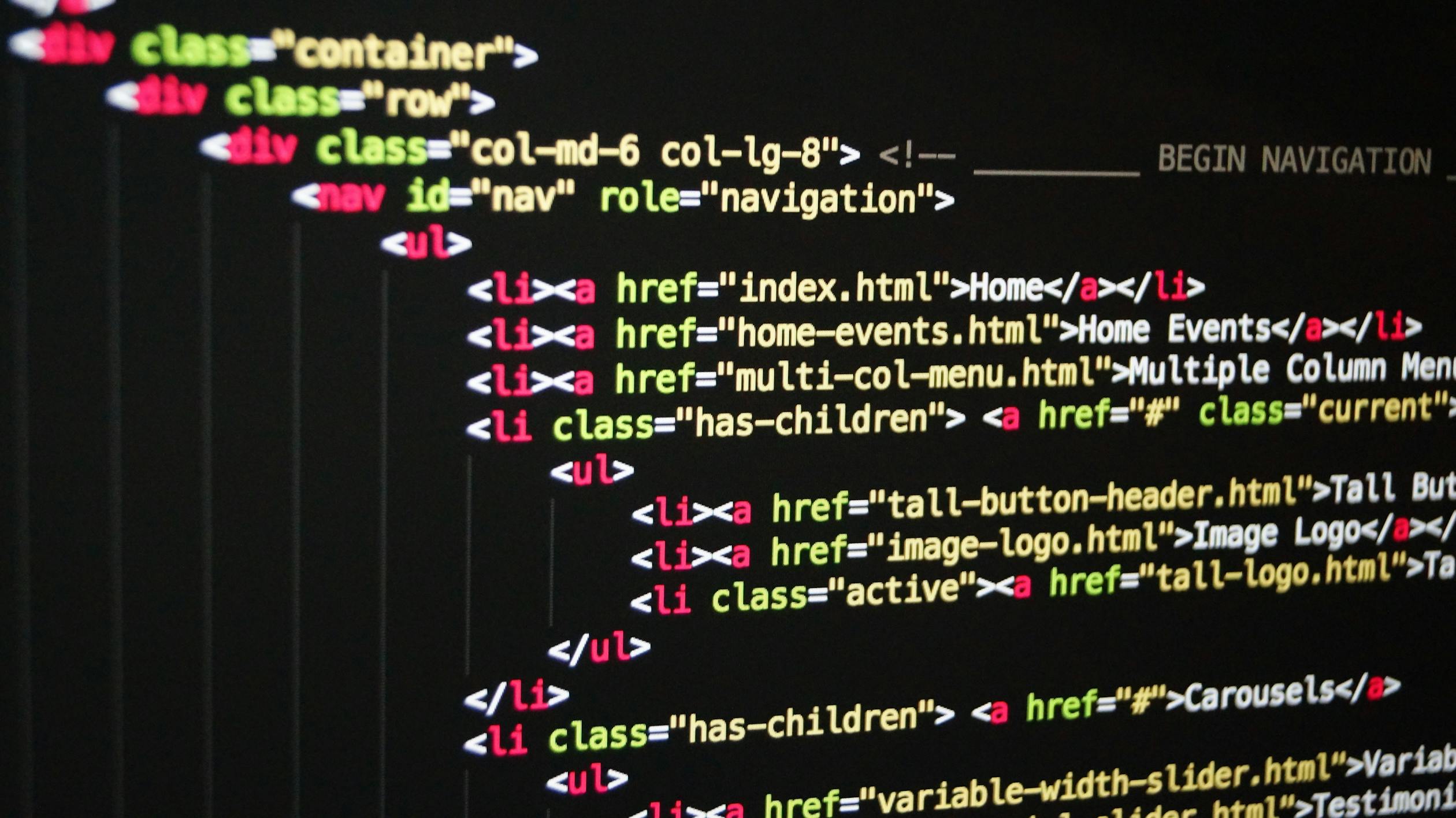
August 16, 2023
The Joy of Interacting with Databases: Laravel's Eloquent ORM
In the world of web development, managing databases smoothly and efficiently is crucial. Laravel, a popular PHP framework, offers an incredible tool called Eloquent, which simplifies database interactions and amplifies the joy of working with databases. In this blog post, we'll explore how Eloquent, an object-relational mapper (ORM), enhances the overall experience of managing databases in Laravel.
1. What is Eloquent?
Eloquent is an ORM bundled with Laravel that provides an intuitive and expressive way to interact with databases. It serves as a bridge between your application and the database, making database operations a breeze. Eloquent abstracts database tables into objects called Models, enabling developers to interact with databases using familiar object-oriented syntax.
2. Models: Your Gateway to Database Operations
In Laravel, each database table has a corresponding Model that acts as an intermediary for interacting with that table. Models encapsulate all the logic required to perform database operations, eliminating the need to write raw SQL queries. With Eloquent models, developers can create, retrieve, update, and delete records effortlessly.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Flight extends Model
{
/**
* The table associated with the model.
*
* @var string
*/
protected $table = 'my_flights';
}
3. Retrieving Records: The Eloquent Way
Eloquent makes retrieving records from the database a breeze. Using fluent and chainable methods, you can build complex queries in a concise and readable manner. Whether it's retrieving a single record, filtering results based on specific conditions, or sorting data, Eloquent provides an expressive syntax that simplifies the process.
use App\Models\Flight;
foreach (Flight::all() as $flight) {
echo $flight->name;
}
4. Creating and Updating Records: Simplicity Redefined
Eloquent takes the complexity out of creating and updating records in your database. With just a few lines of code, you can leverage Eloquent's methods to insert new records or update existing ones. Eloquent's syntax is not only concise but also offers advanced features like mass assignment protection, making it a secure choice for working with databases.
use App\Models\Flight;
$flight = Flight::find(1);
$flight->name = 'Paris to London';
$flight->save();
5. Deleting Records: Easy and Hassle-Free
Eloquent's approach to deleting records is equally straightforward. By invoking a single method on the corresponding Model, you can seamlessly remove records from your database. Whether you need to delete a single record or multiple ones, Eloquent provides the necessary tools to simplify the process.
use App\Models\Flight;
$flight = Flight::find(1);
$flight->delete();
6. Relationships: Uniting Data Effortlessly
One of Eloquent's standout features is its support for database relationships. Whether it's one-to-one, one-to-many, or many-to-many relationships, Eloquent makes handling related data a breeze. These relationships allow you to access associated records effortlessly, saving you from the hassle of writing complex SQL joins.
$user->posts()->where('active', 1)->get();
Follow me to learn more about Laravel ORM Relations
Conclusion:
Laravel's Eloquent ORM transforms the way developers interact with databases. With its intuitive syntax and powerful features, Eloquent makes working with databases an enjoyable experience. From retrieving records to creating, updating, and deleting them, Eloquent simplifies every aspect of database management. Embrace Eloquent's elegance, and you'll find yourself appreciating the sheer joy it brings to your Laravel projects.
208 views