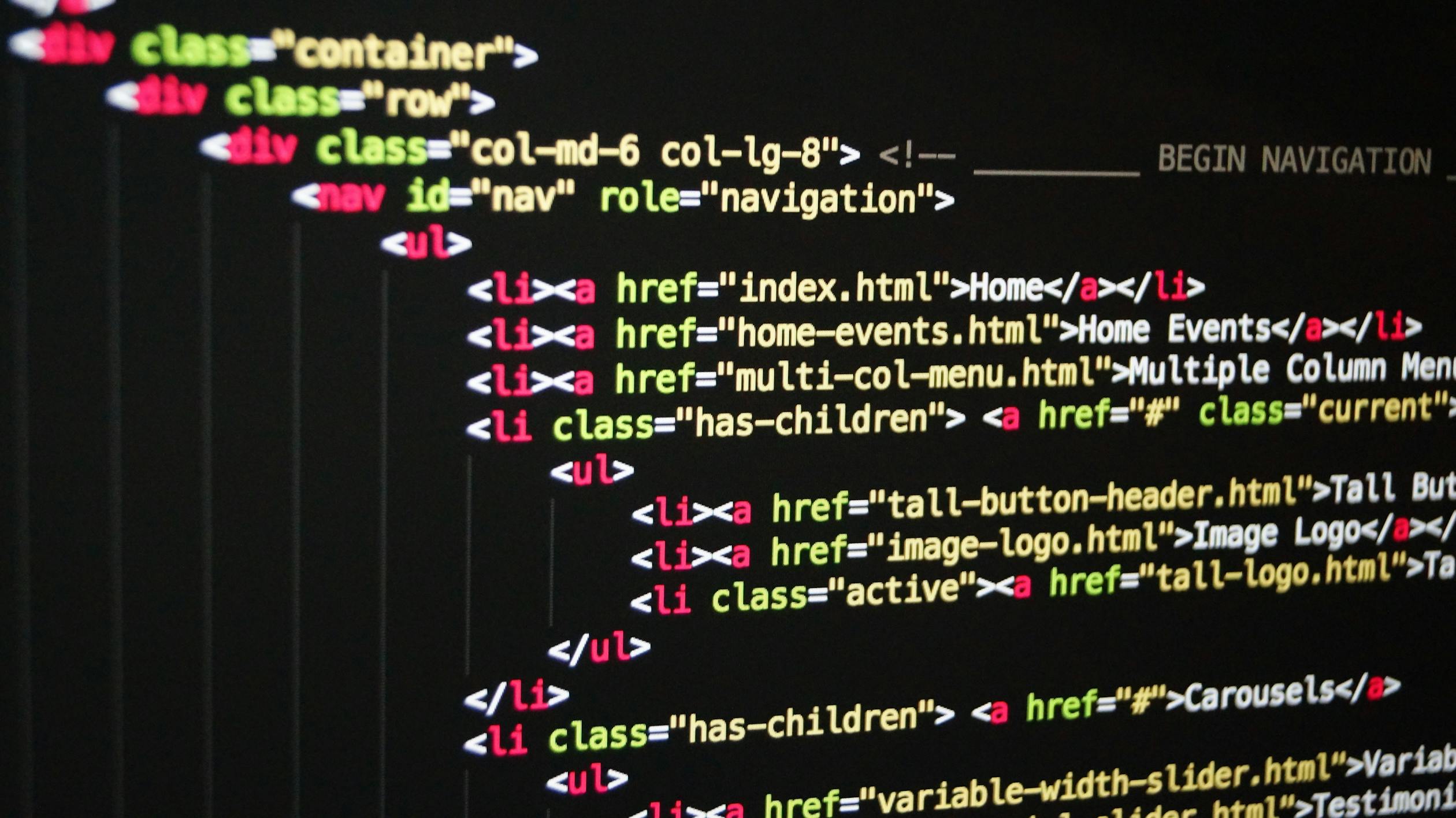
October 26, 2023
String theories (and practice) in Python
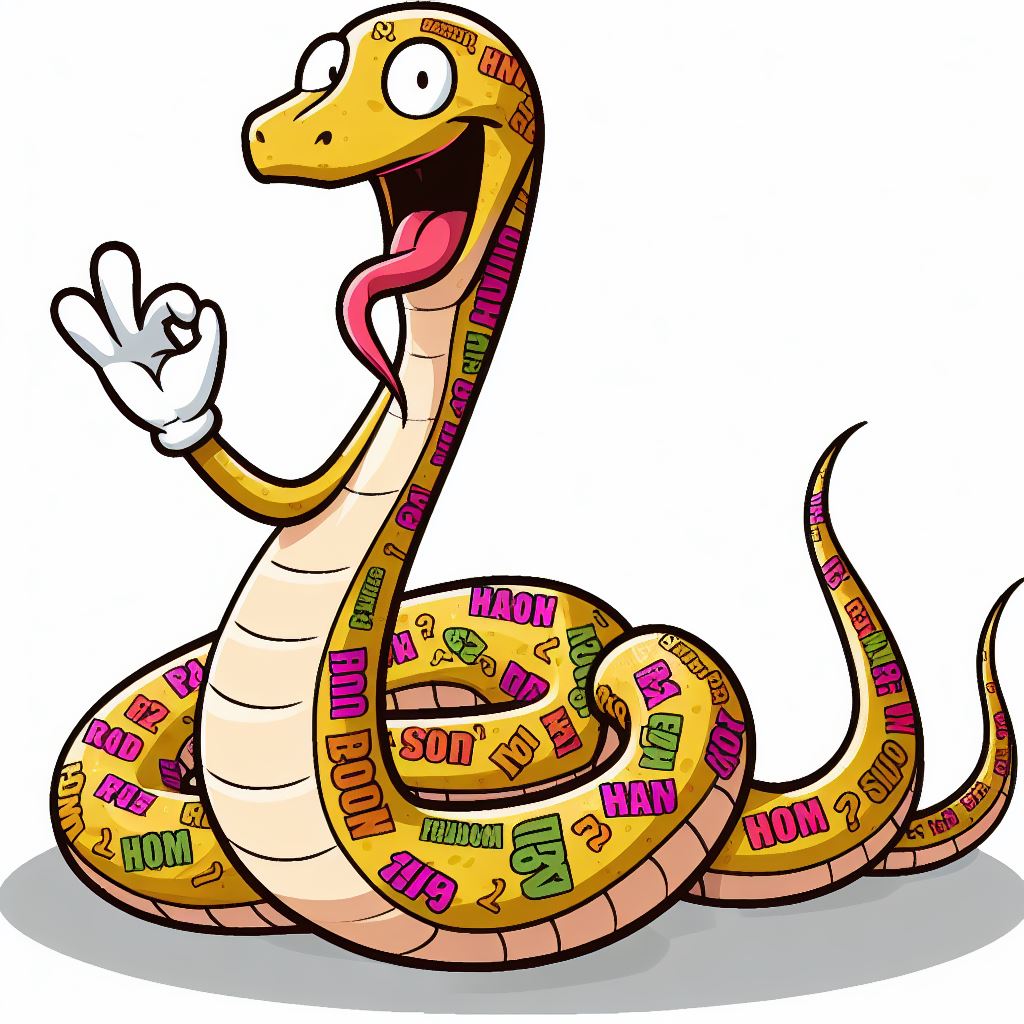
Purpose
This tutorial will introduce beginners and learners to working with strings in Python.
Audience
This document is suitable for Python beginners or those learning programming in general.
Prerequisites
- a Python interpreter installed on your machine - if you are new to Python, check out my introduction
- text editor or IDE
Introduction
A sequence of characters is a 'string'. For example, a long, complex password containing letters, numbers, and symbols is a string. "Feet or no feet, I can keep abreast of all thy four" is a string. "37.422131&-122.084801" is a string. You get the idea. Any programming language worth its salt has in-built capabilities for working with this essential data type. Let's look at Python.
Step-by-Step
Step 1 - Make and join strings
- create a new file "string.py"
- create a string by quoting it
x = 'Py'
print(x)
% python strings.py
Py
- create a string by copying an existing one
x = 'Py'
y = x
print(x)
print(y)
% python strings.py
Py
Py
The variable 'y' is a copy of 'x'. Changing the contents of 'x' will not affect 'y'.
Step 2 - Joining strings together
In computer science, the process of joining strings together is called 'concatenation'. To concatenate strings, Python provides the "+" operators.
NOTE: "concatenate" is derived from Latin words meaning "chain together". There, now you know where this strange word came from!
string1 = "Py"
string2 = "thon"
print(string1 + string2)
% python strings.py
Python
- Join more than two strings:
s1= "Feet "
s2 = "or "
s3 = "no "
s4 = "feet"
print(s1 + s2 + s3 + s4)
% python strings.py
Feet or no feet
Step 3 - Some escapism
Let's escape into a book. Consider this quote from "The Jungle Book".
"Feet or no feet, I can keep abreast of all thy four," said Kaa shortly.
The text contains quotes. But Python syntax also requires quotes around our strings.
- Try making a string with this text:
% python
>>> string = ""Feet or no feet, I can keep abreast of all thy four," said Kaa shortly."
File "<stdin>", line 1
string = ""Feet or no feet, I can keep abreast of all thy four," said Kaa shortly."
SyntaxError: invalid syntax
How would we represent this piece of text, including the quotes, in a string? The answer is escaping. Escaping means telling the interpreter to interpret the subsequent character or characters differently. To tell the Python interpreter to handle quotes or any special characters in a different way, we use the \ character.
- Let's 'escape' our quotes.
string = "\"Feet or no feet, I can keep abreast of all thy four,\" said Kaa shortly."
print(string)
"Feet or no feet, I can keep abreast of all thy four," said Kaa shortly.
...the quote from Kipling's famous python is intact!
Step 4 - How long is a string?
Python provides a useful function that tells us a string's length - "len()".
string = "\"Feet or no feet, I can keep abreast of all thy four,\" said Kaa shortly."
print(len(string))
72
Step 5 - Check if text exists in a string
Python's "in" operator (among other things) tells us if a character or string exists in another string. 'in' returns a value of True if it finds a match, and False if not.
NOTE: True and False are Boolean data types. A Boolean can only be true (1) or false (0).
- Search our text for various famous serpents.
string = "\"Feet or no feet, I can keep abreast of all thy four,\" said Kaa shortly."
snake1 = "Kaa"
snake2 = "Nagini"
print(snake1 in string)
print(snake2 in string)
True
False
Step 6 - Upper and lower cases
The last two in-built functions we will consider are .upper() and .lower() - these return a string containing the original string text in upper or lowercase.
NOTE: Strings are immutable - they cannot be changed once created. But we can created modified copies of them.
original_string = "\"Feet or no feet, I can keep abreast of all thy four,\" said Kaa shortly."
all_caps = original_string.upper()
all_lower = original_string.lower()
print(all_caps)
print(all_lower)
print(original_string)
% python strings.py
"FEET OR NO FEET, I CAN KEEP ABREAST OF ALL THY FOUR," SAID KAA SHORTLY.
"feet or no feet, i can keep abreast of all thy four," said kaa shortly.
"Feet or no feet, I can keep abreast of all thy four," said Kaa shortly.
Notice that even after we ran the upper() and lower() functions against the original_string variable, the value of that variable did not change. Python stored the modified value in the new string variables we created.
Next Steps
This is not a comprehensive guide to Python's string capabilities, in fact we have only scratched the surface. But, you should be comfortable with playing around with strings in Python! If you would like to learn more about strings, I recommend Google's Education page on the topic or W3 Schools.
print("Happy stringing!")
235 views