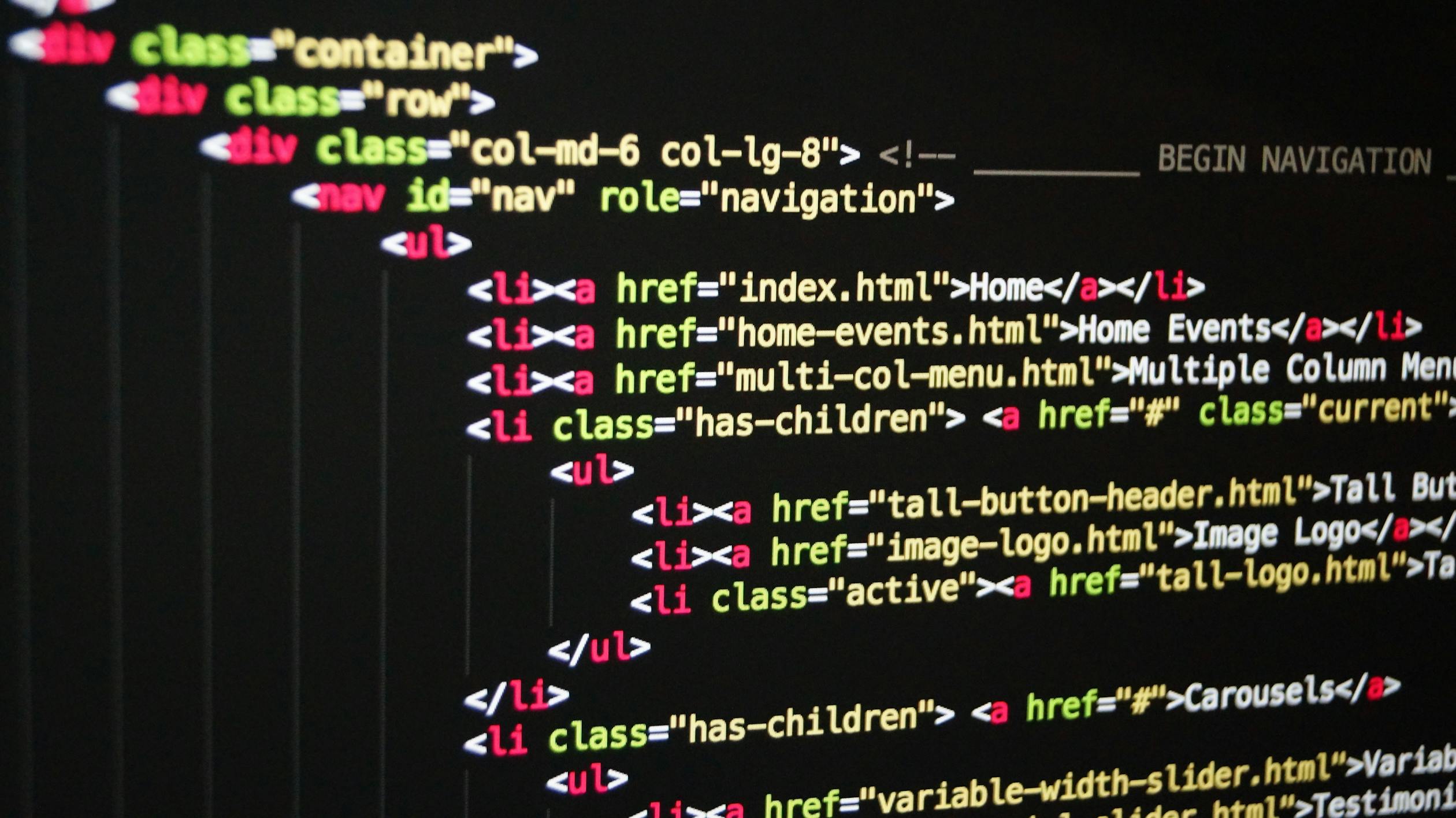
September 22, 2023
Mastering Laravel Migration: Building Rock-Solid Database Schemas
Introduction:
In modern web development, having an efficient and consistent database schema is crucial for creating scalable and maintainable applications. Laravel, one of the most popular PHP frameworks, offers a powerful tool called Migration to manage database changes effortlessly. This article explores the key features and benefits of Laravel Migration, providing a comprehensive guide for developers to build seamless database schemas.
Understanding Laravel Migration:
Laravel Migration is an essential component that allows developers to define and manage database schema using PHP code. It provides a structured approach to create tables, columns, and relationships, making it easier to version control and share changes across development teams. Migration ensures that the database schema stays synchronized with the application's codebase, helping in maintaining data integrity.
Benefits of Laravel Migration:
1. Version Control: With Migration, developers can maintain a history of database changes in a version-controlled manner. This approach allows easy rollbacks and collaboration among team members, reducing the risk of conflicts while developing enterprise-level applications.
2. Database Portability: Migration facilitates the portability of database schemas across different environments. Developers can effortlessly migrate the database structure between development, staging, and production environments without the need for manual schema modifications.
3. Data Preservation: Laravel Migration allows developers to transform database structures while preserving the existing data. It eliminates the need for manual data manipulation during schema updates, ensuring data integrity and reducing the chance of data loss.
4. Rapid Deployment: By utilizing Migration, developers can deploy database schema changes alongside application code changes seamlessly. This makes the deployment process faster and more efficient, minimizing downtime and improving the overall development workflow.
Getting Started with Laravel Migration:
1. Installation: Laravel Migration comes bundled with Laravel framework by default. Ensure that you have the latest version of Laravel installed before proceeding.
To create Laravel project example-app
. Read more at https://laravel.com/docs/10.x/installation to install laravel in different operation system
composer create-project laravel/laravel example-app
2. Migration Files: Migration files are created using the Artisan command-line interface. These files are located in the "database/migrations" directory and contain two primary methods: "up" and "down." The "up" method defines the actions to create or modify database structures, while the "down" method undoes those actions.
Create Migration file
php artisan make:migration create_flights_table
Sample Migration file
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('flights', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('airline');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::drop('flights');
}
};
3. Schema Builder: Laravel provides a Schema Builder to define database structure fluently. It supports various column types, relationships, indexes, and constraints. Developers can utilize functions like "create," "table," "addColumn," and "dropColumn" to modify the schema effortlessly.
Schema builder Renaming Columns
Schema::table('users', function (Blueprint $table) {
$table->renameColumn('from', 'to');
});
Schema builder Dropping Columns
Schema::table('users', function (Blueprint $table) {
$table->dropColumn('votes');
});
4. Running Migrations: Laravel's Artisan CLI allows running migrations using the "migrate" command. This command executes all pending migrations and updates the database schema accordingly. Developers can also perform rollbacks using the "migrate:rollback" command.
php artisan migrate
5. Migration Seeds: Besides schema migrations, Laravel's Migration feature also supports data seeding. Developers can populate the database with initial or test data by creating seed classes and executing them using Artisan CLI. I attached a sample below but I am going to create a dedicate totorial for it.
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Str;
class DatabaseSeeder extends Seeder
{
/**
* Run the database seeders.
*/
public function run(): void
{
DB::table('users')->insert([
'name' => Str::random(10),
'email' => Str::random(10).'@gmail.com',
'password' => Hash::make('password'),
]);
}
}
Best Practices for Laravel Migration:
1. Naming Conventions: Follow a consistent naming convention for migration files and classes. Use informative and descriptive names that reflect the changes being made, making it easier to identify and understand migrations.
2. Test Your Migrations: Before deploying migrations to production, thoroughly test them in development and staging environments. This minimizes the chances of errors and conflicts, ensuring a smooth transition.
3. Documentation: Document migrations properly, including information on the purpose, expected outcomes, and any specific considerations. This helps in maintaining an organized and well-documented codebase, making it easier for developers to understand and work collaboratively.
Conclusion:
Laravel Migration serves as a powerful tool for developers to build and maintain efficient, consistent, and scalable database schemas. By enabling straightforward versioning, improved portability, data preservation, and rapid deployment, Laravel Migration simplifies the database management process. Following best practices and leveraging Laravel's Schema Builder, developers can effortlessly create and modify database structures, resulting in seamless and robust applications.
424 views