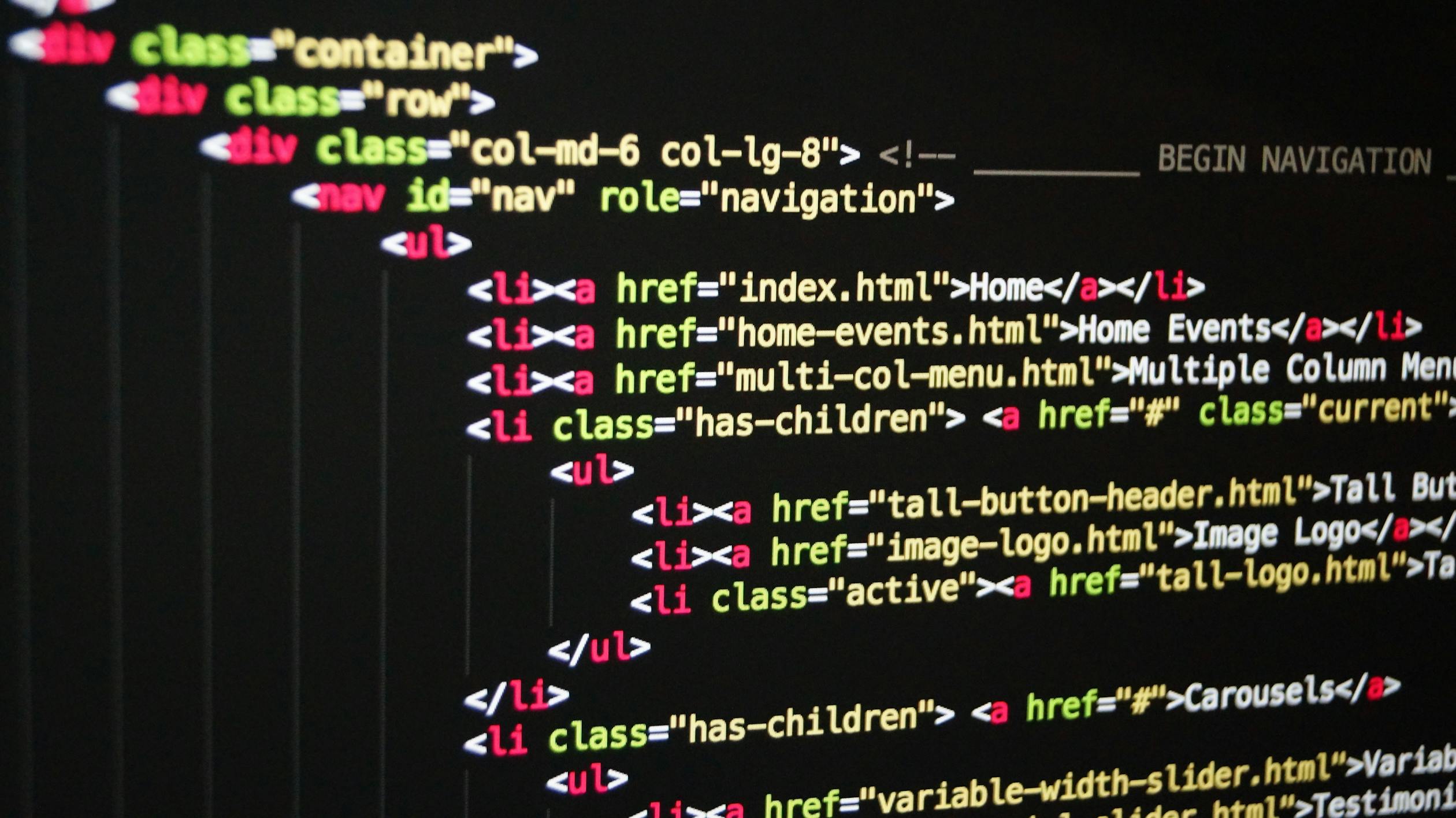
September 06, 2023
Laravel Precognition (VueJs/Inertia)
By utilizing the integration of React/VueJs with Laravel Precognition, you can seamlessly deliver real-time validation experiences to your users. With this combined approach, you eliminate the need to duplicate validation rules in your frontend application.
In the upcoming example, we will demonstrate the integration of Laravel Precognition with Vue.Js and Inertia for the frontend. If you are using React/Vue.Js with Inertia, please refer to the comprehensive documentation available at: https://laravel.com/docs/10.x/precognition.
To guide you through the process of integrating Laravel Precognition, I have outlined a step-by-step guide below. Additionally, I have embedded a helpful video from the official Laravel channel for those who prefer a visual tutorial.
In order to utilize Precognition for a specific route, you need to include the HandlePrecognitiveRequests middleware in the route's definition. Additionally, it is recommended to create a dedicated form request to encapsulate the validation rules for the route.
To enable Precognition, follow these steps:
Add the HandlePrecognitiveRequests middleware to the route definition:
use App\Http\Requests\StoreUserRequest;
use Illuminate\Foundation\Http\Middleware\HandlePrecognitiveRequests;
Route::post('/users', function (StoreUserRequest $request) {
// ...
})->middleware([HandlePrecognitiveRequests::class]);
By including the 'HandlePrecognitiveRequests
' middleware, you enable Precognition for this route.
Additionally, when working with Vue and Inertia, it is essential to install the Inertia-compatible Precognition library through NPM.
npm install laravel-precognition-vue-inertia
After installing Precognition, you can use the useForm function to get an Inertia form helper that includes the discussed validation features. The submit method of the form helper has been simplified by removing the requirement to specify the HTTP method or URL. Now, you can pass Inertia's visit options as the first and only argument. Instead, you can choose from any of Inertia's supported event callbacks and provide them in the visit options for the submit method.
<script setup>
import { useForm } from 'laravel-precognition-vue-inertia';
const form = useForm('post', '/users', {
name: '',
email: '',
});
const submit = () => form.submit({
preserveScroll: true,
onSuccess: () => form.reset(),
});
</script>
<template>
<form @submit.prevent="submit">
<label for="name">Name</label>
<input
id="name"
v-model="form.name"
@input="form.validate('name') && form.clearErrors()"
/>
<div v-if="form.invalid('name')">
{{ form.errors.name }}
</div>
<label for="email">Email</label>
<input
id="email"
type="email"
v-model="form.email"
@input="form.validate('email') && form.clearErrors()"
/>
<div v-if="form.invalid('email')">
{{ form.errors.email }}
</div>
<button :disabled="form.processing">
Create User
</button>
</form>
</template>
By following these steps, you can efficiently enable Precognition for a specific route and utilize a dedicated form request to handle its validation rules. Additionally you may configure the debounce timeout by calling the form's setValidationTimeout function:
form.setValidationTimeout(3000);
573 views