1 year ago
#382400
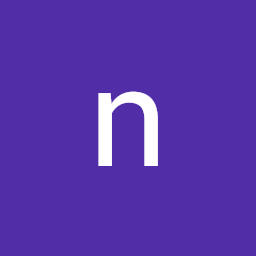
nicolas osorio bustos
How to make this cube a Sphere in P5.js?
I've been developing a procedural cube, which is built in three steps.
- Setting up the vectors
- Drawing the triangles needed
- Normalizing to convert to sphere
My problem regards to the last step, when I normalize the vectors it only shows a quarter of a sphere. Could anyone help me out with this?
let world;
function setup() {
createCanvas(500, 500, WEBGL);
world = new cube(9, 80); // Testing values
}
function draw() {
background(200);
world.createVertices();
//world.drawPoints();
world.createTriangles();
world.drawTriangles();
}
class cube {
constructor(resolution,scale,vertices, triangles, roundness, normals) {
this.resolution = resolution;
this.vertices = vertices;
this.scale = scale;
this.triangles = triangles;
this.normals = normals;
}
createVertices() {
let cornerVertices = 8;
let edgeVertices = ((this.resolution * 3) - 3) * 4;
let faceVertices = ((this.resolution - 1) * (this.resolution - 1) +(this.resolution - 1) * (this.resolution - 1) +(this.resolution- 1) * (this.resolution - 1)) * 2;
let totalVertices = cornerVertices + edgeVertices + faceVertices // For checking purposes only
this.vertices = []
this.normals = []
let v = 0;
for (let y = 0; y <= this.resolution; y++) {
for (let x = 0; x <= this.resolution; x++) {
this.SetVertex(v++, x, y, 0);
}
for (let z = 1; z <= this.resolution; z++) {
this.SetVertex(v++, this.resolution, y, z);
}
for (let x = this.resolution - 1; x >= 0; x--) {
this.SetVertex(v++, x, y, this.resolution)
}
for (let z = this.resolution - 1; z > 0; z--) {
this.SetVertex(v++, 0, y, z);
}
}
for (let z = 1; z < this.resolution; z++) {
for (let x = 1; x < this.resolution; x++) {
this.SetVertex(v++, x, this.resolution, z);
}
}
for (let z = 1; z < this.resolution; z++) {
for (let x = 1; x < this.resolution; x++) {
this.SetVertex(v++, x, 0, z);
}
}
console.log(this.vertices)
console.log(totalVertices)
}
createTriangles() {
let quads = (this.resolution * this.resolution + this.resolution * this.resolution + this.resolution * this.resolution) * 2; // For checking purposes only
this.triangles = [];
let ring = (this.resolution * 2) * 2;
let t = 0
let v = 0;
for (let y = 0; y < this.resolution; y++, v++) {
for (let q = 0; q < ring - 1; q++, v++) {
t = this.SetQuad(this.triangles, t, v, v + 1, v + ring, v + ring + 1);
}
t = this.SetQuad(this.triangles, t, v, v - ring + 1, v + ring, v + 1);
}
t = this.CreateTopFace(this.triangles, t, ring);
t = this.CreateBottomFace(t, ring);
console.log(this.triangles)
}
drawPoints() {
strokeWeight(1);
let angleX = 200
let angleY = 500
let angleZ = 100
beginShape(POINTS);
for(let i = 0; i < this.vertices.length; i++) {
vertex(this.vertices[i].x * this.scale,this.vertices[i].y * -this.scale,this.vertices[i].z * this.scale)
rotateX(angleX)
rotateY(angleY)
rotateZ(angleZ)
}
endShape();
}
drawTriangles(){
let coordinates = [];
for(let i = 0; i < this.triangles.length; i++){
coordinates.push(this.vertices[this.triangles[i]])
}
console.log(coordinates)
for(let i = 0; i < coordinates.length; i = i + 3) {
beginShape(TRIANGLES);
vertex(coordinates[i].x * this.scale,coordinates[i].y * -this.scale,coordinates[i].z * this.scale)
vertex(coordinates[i + 1].x * this.scale,coordinates[i + 1].y * -this.scale,coordinates[i + 1].z * this.scale)
vertex(coordinates[i + 2].x * this.scale,coordinates[i + 2].y * -this.scale,coordinates[i + 2].z * this.scale)
endShape();
}
}
// Define vertex order.
SetQuad (triangles, i, v00, v10, v01, v11) {
triangles[i] = v00;
triangles[i + 1] = triangles[i + 4] = v01;
triangles[i + 2] = triangles[i + 3] = v10;
triangles[i + 5] = v11;
return i + 6;
}
CreateTopFace(triangles,t, ring) {
let v = ring * this.resolution;
for (let x = 0; x < this.resolution - 1; x++, v++) {
t = this.SetQuad(triangles, t, v, v + 1, v + ring - 1, v + ring);
}
t = this.SetQuad(triangles, t, v, v + 1, v + ring - 1, v + 2);
let vMin = ring * (this.resolution + 1) - 1;
let vMid = vMin + 1;
let vMax = v + 2;
for (let z = 1; z < this.resolution - 1; z++, vMin--, vMid++, vMax++) {
t = this.SetQuad(triangles, t, vMin, vMid, vMin - 1, vMid + this.resolution- 1);
for (let x = 1; x < this.resolution - 1; x++, vMid++) {
t = this.SetQuad(triangles, t, vMid, vMid + 1, vMid + this.resolution - 1, vMid + this.resolution);
}
t = this.SetQuad(triangles, t, vMid, vMax, vMid + this.resolution - 1, vMax + 1);
}
let vTop = vMin - 2;
t = this.SetQuad(triangles, t, vMin, vMid, vTop + 1, vTop);
for (let x = 1; x < this.resolution - 1; x++, vTop--, vMid++) {
t = this.SetQuad(triangles, t, vMid, vMid + 1, vTop, vTop - 1);
}
t = this.SetQuad(triangles, t, vMid, vTop - 2, vTop, vTop - 1);
return t;
}
CreateBottomFace (triangles, t, ring) {
let v = 1;
let vMid = this.vertices.length - (this.resolution - 1) * (this.resolution - 1);
t = this.SetQuad(triangles, t, ring - 1, vMid, 0, 1);
for (let x = 1; x < this.resolution - 1; x++, v++, vMid++) {
t = this.SetQuad(triangles, t, vMid, vMid + 1, v, v + 1);
}
t = this.SetQuad(triangles, t, vMid, v + 2, v, v + 1);
let vMin = ring - 2;
vMid -= this.resolution - 2;
let vMax = v + 2;
for (let z = 1; z < this.resolution - 1; z++, vMin--, vMid++, vMax++) {
t = this.SetQuad(triangles, t, vMin, vMid + this.resolution - 1, vMin + 1, vMid);
for (let x = 1; x < this.resolution - 1; x++, vMid++) {
t = this.SetQuad(triangles, t,vMid + this.resolution - 1, vMid + this.resolution, vMid, vMid + 1);
}
t = this.SetQuad(triangles, t, vMid + this.resolution - 1, vMax + 1, vMid, vMax);
}
let vTop = vMin - 1;
t = this.SetQuad(triangles, t, vTop + 1, vTop, vTop + 2, vMid);
for (let x = 1; x < this.resolution - 1; x++, vTop--, vMid++) {
t = this.SetQuad(triangles, t, vTop, vTop - 1, vMid, vMid + 1);
}
t = this.SetQuad(triangles, t, vTop, vTop - 1, vMid, vTop - 2);
return t;
}
SetVertex (i, x, y, z) {
let v = createVector(x, y, z)
v = v.mult(2)
v = v.div(this.resolution - 1)
this.normals[i] = v.normalize();
this.vertices[i] = this.normals[i];
}
}
javascript
3d
p5.js
0 Answers
Your Answer