1 year ago
#375132
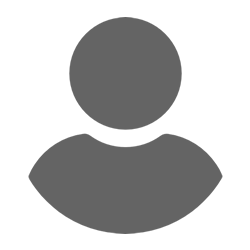
user17697729
How do I initialize my DLL (made in C#) and call its methods from VBA?
I'm trying to create a DLL file that will help me to access and read PDF files to Excel. As can be seen in the attached photo I've added the .tlb file to the reference library in Excel but I'm not able to run it without an error (Error 438).
I've created a Main Method in VisualStudio to test PdfExtractor class with good results. So my question is how do I initialize an object from my DLL file and pass arguments to it?
My VBA code:
Option Explicit
Sub PdfToExcel()
Dim vbObj As New PdfParser.PdfExtractor
Dim words As String
With vbObj
'Error 438
.PdfExtractor ("C:\Users\user\Downloads\54.pdf")
words = .readPDF
End With
End Sub
I have selected "Make assembly COM-Visible" and "Register for COM interop". My C# code (VB - Class Library (.NET Framework):
using iTextSharp.text.pdf;
using iTextSharp.text.pdf.parser;
using System;
using System.Runtime.InteropServices;
using System.Text;
namespace PdfParser
{
[ComVisible(true)]
public class PdfExtractor
{
private string url = string.Empty;
private string path = string.Empty;
private string getPageFromPdf = string.Empty;
private PdfReader pdfReader;
public PdfExtractor(string address)
{
try
{
if (address.Contains("http")){
this.url = address;
this.pdfReader = new PdfReader(new Uri(url));
}
else
{
this.path = address;
this.pdfReader = new PdfReader(path);
}
}
catch
{
}
}
public PdfExtractor(){}
[ComVisible(true)]
public string Url { get { return url; } set { url = value; } }
[ComVisible(true)]
public string Path { get { return path; } set { path = value; } }
[ComVisible(true)]
public void setPdfReader()
{
if (path != string.Empty) this.pdfReader = new PdfReader(path); else this.pdfReader = new PdfReader(new Uri(url));
}
[ComVisible(true)]
public string readPDF()
{
return parsePage();
}
private string parsePage()
{
try
{
for (int i = 1; i <= pdfReader.NumberOfPages; i++)
{
ITextExtractionStrategy its = new iTextSharp.text.pdf.parser.LocationTextExtractionStrategy();
string stringToConvert = iTextSharp.text.pdf.parser.PdfTextExtractor.GetTextFromPage(pdfReader, i, its);
stringToConvert = Encoding.UTF8.GetString(ASCIIEncoding.Convert(Encoding.Default, Encoding.UTF8, Encoding.Default.GetBytes(stringToConvert)));
getPageFromPdf = getPageFromPdf + stringToConvert;
}
pdfReader.Close();
return getPageFromPdf;
}
catch (Exception e)
{
return e.Message;
}
}
}
}
c#
vba
dllimport
0 Answers
Your Answer