1 year ago
#357528
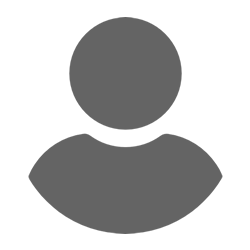
user18630166
What are the performance drawbacks of using global symbols in a getter and setter methods?
The JavaScript code below has a getter and setter for name
using global symbols, and age_
using a dangling underscore.
JavaScript Code
function Human(name, age) {
this.name = name;
this.age_ = age;
}
Human.prototype.greet = function() {
console.log(`Hello ${this.name}! You are ${this.age} years old.`);
};
Human.prototype[Symbol.iterator] = function* () {
yield* Object.getOwnPropertyNames(this);
yield* Object.getOwnPropertySymbols(this);
};
Object.defineProperties(
Human.prototype,
{
name: {
get() {
return this[Symbol.for('name')];
},
set(value) {
this[Symbol.for('name')] = value;
},
},
age: {
get() {
return this.age_;
},
set(value) {
this.age_ = value;
},
},
},
);
let mary = new Human('Mary Smith', 18);
mary.greet();
console.dir(mary);
let john = new Human('John Doe', 25);
john.greet();
console.dir(john);
Console Output
I want to know is there any performance drawbacks to using global symbols for a getter and setter methods because I find it much cleaner code.
javascript
getter-setter
0 Answers
Your Answer