1 year ago
#338136
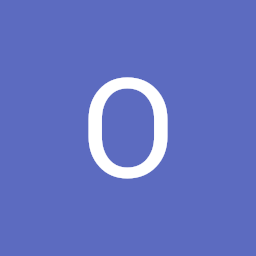
Voth
Sign In Problem Authorization and Authentication
Hi guys i have 2 question in this topic
Question 1:
I'm trying to sign in but user is coming null when i FindByNameAsync
Registered user's normalizedUserNames is always created null in sql too
im editing in sql normalizedusername then FindByNameAsync found my user but it is problem for me every single register edit.
Question 2: When i try to login after editing normalizingUserName this time claim is null reference issue.I dont understand where is my problem in codes. Pls help me about this issues and if you have good resources about role based user identity, Authorization and authentication share with me plz.
Create Controller :
[AllowAnonymous]
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> CreateUser(RegisterViewModel model)
{
if (ModelState.IsValid)
{
AppUser user = new AppUser();
user.UserName = model.Username;
user.NormalizedUserName = model.Username.ToLower();
user.Password = model.Password;
user.Email = model.Email;
var result = await userManager.CreateAsync(user, model.Password);
user.NormalizedUserName = user.UserName.ToUpper();
await userManager.UpdateNormalizedUserNameAsync(user);
if (result.Succeeded)
{
return RedirectToAction("Index", "Home", user.Id);
}
else
{
foreach (var item in result.Errors)
{
ModelState.AddModelError("", item.Description);
}
}
}
return View(model);
}
Login Controller:
[AllowAnonymous]
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Index(LoginViewModel model)
{
if (model == null)
{
return View();
}
if (ModelState.IsValid)
{
***Question 1***
AppUser user = await userManager.FindByNameAsync(model.Username);
if (user != null)
{
if(userManager.CheckPasswordAsync(user, model.Password).Result)
{
await signInManager.SignOutAsync();
***Question 2***
var result = await signInManager.PasswordSignInAsync(user.UserName, model.Password, false,false);
if (result.Succeeded)
{
return RedirectToAction("Index", "Admin", user.Id);
}
}
else
{
ModelState.AddModelError("PasswordWrong", "Password is wrong");
return View(model);
}
}
}
model.Password = "";
return RedirectToAction("Index", "Account", model);
}
Program cs:
builder.Services.AddDbContext<ApplicationDbContext>(options => options.UseSqlServer(
builder.Configuration.GetConnectionString("DefaultConnection")));
builder.Services.AddTransient<IPasswordValidator<AppUser>, PasswordValidator>();
builder.Services.AddTransient<IUserValidator<AppUser>, UsernameValidator>();
builder.Services.AddMvc();
builder.Services.AddRazorPages().AddRazorRuntimeCompilation();
builder.Services.ConfigureApplicationCookie(options =>
options.LoginPath = "/Account/Login");
builder.Services.AddIdentity<AppUser, IdentityRole>(options =>
{
// Password settings.
options.Password.RequireDigit = true;
options.Password.RequireLowercase = true;
options.Password.RequireNonAlphanumeric = true;
options.Password.RequireUppercase = true;
options.Password.RequiredLength = 6;
options.Password.RequiredUniqueChars = 1;
// Lockout settings.
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5);
options.Lockout.MaxFailedAccessAttempts = 5;
options.Lockout.AllowedForNewUsers = true;
// User settings.
options.User.AllowedUserNameCharacters =
"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789-._@+";
options.User.RequireUniqueEmail = false;
})
.AddEntityFrameworkStores<ApplicationDbContext>()
.AddDefaultTokenProviders();
builder.Services.ConfigureApplicationCookie(options =>
{
// Cookie settings
options.Cookie.HttpOnly = true;
options.ExpireTimeSpan = TimeSpan.FromMinutes(5);
options.LoginPath = "/Identity/Account/Login";
options.AccessDeniedPath = "/Identity/Account/AccessDenied";
options.SlidingExpiration = true;
});
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production
scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Account}/{action=Index}/{id?}");
app.Run();
asp.net-core
authentication
authorization
asp.net-identity
user-roles
0 Answers
Your Answer