1 year ago
#334221
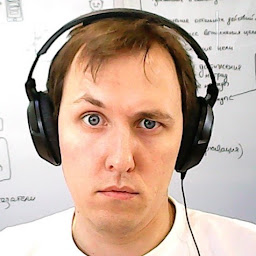
Grigory Zhadko
Boxing vs ToString for int
I have a .net 6 project and write some logs. The usual log looks:
int profileId = 100; //Any number
_logger.LogInformation("profileId = {ProfileId}", profileId);
Here, the boxing happens (int -> object). So, I decided to replace the code with:
int profileId = 100; //Any number
string profileIdString = profileId.ToString();
_logger.LogInformation("profileId = {ProfileId}", profileIdString);
However, I decided to run the benchmark and compare both options. Surprisingly, the results are not the ones I expected. Here is the code.
[MemoryDiagnoser]
public class Benchmark
{
private const int N = 1000;
[Benchmark]
public void Boxing()
{
for (var i = 0; i < N; i++)
{
var s = string.Format("Test: {0}", i);
}
}
[Benchmark]
public void CastToString()
{
for (var i = 0; i < N; i++)
{
var s = string.Format("Test: {0}", i.ToString());
}
}
}
public class Program
{
public static void Main(string[] args)
{
var summary = BenchmarkRunner.Run(typeof(Program).Assembly);
}
}
Output:
| Method | Mean | Error | StdDev | Gen 0 | Allocated |
|------------- |---------:|---------:|---------:|-------:|----------:|
| Boxing | 26.41 us | 0.236 us | 0.221 us | 4.8828 | 62 KB |
| CastToString | 32.91 us | 0.297 us | 0.278 us | 5.4626 | 70 KB |
It looks like the boxing approach works better: It runs faster and allocates less memory. My question is why does it happen? Does it mean that it is better to use the boxing approach in cases like this? Or did I write the performance test incorrectly?
c#
performance
benchmarking
.net-6.0
benchmarkdotnet
0 Answers
Your Answer