1 year ago
#311252
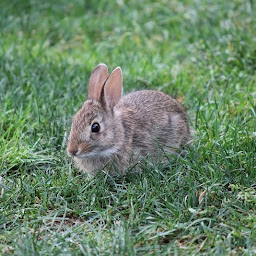
Bunny
How to use python asyncio to asynchronously dispatch tasks?
I am looking to implement a simple p2p file downloader in python. The downloader needs to work within the following limitations:
- When queried, the tracker responds with exactly 2 peers
- The tracker ignores requests made within 2 seconds of the previous request
The downloader should attempt to download a single file as fast as possible. From my reading about this I thought that I should be using asyncio. I thought I'd structure the code something like this pseudo code:
async def downloader(peer):
while file is not downloaded:
download a new block from the peer without blocking
response = synchronously query tracker for initial pair of peers
newPeers = exractPeers(response)
while file not downloaded:
for peer in newPeers:
dispatch new downloader(peer)
wait 2 seconds without blocking downloaders
response = query tracker for initial pair of peers without blocking downloaders
newPeers = exractPeers(response)
What asyncio methods should I be using to "dispatch" new downloaders and query the tracker? From my testing it seems that await
ing an async function blocks the event loop:
import asyncio
from random import randint
async def myfunc(i):
print("hello", i)
await asyncio.sleep(randint(1,3))
print("world", i)
async def main():
await myfunc(1)
await myfunc(2)
await myfunc(3)
await myfunc(4)
asyncio.run(main())
This code runs each myfunc
call as though it were defined without async
. I'd appreciate anything, including basic pointers.
python
python-asyncio
p2p
0 Answers
Your Answer