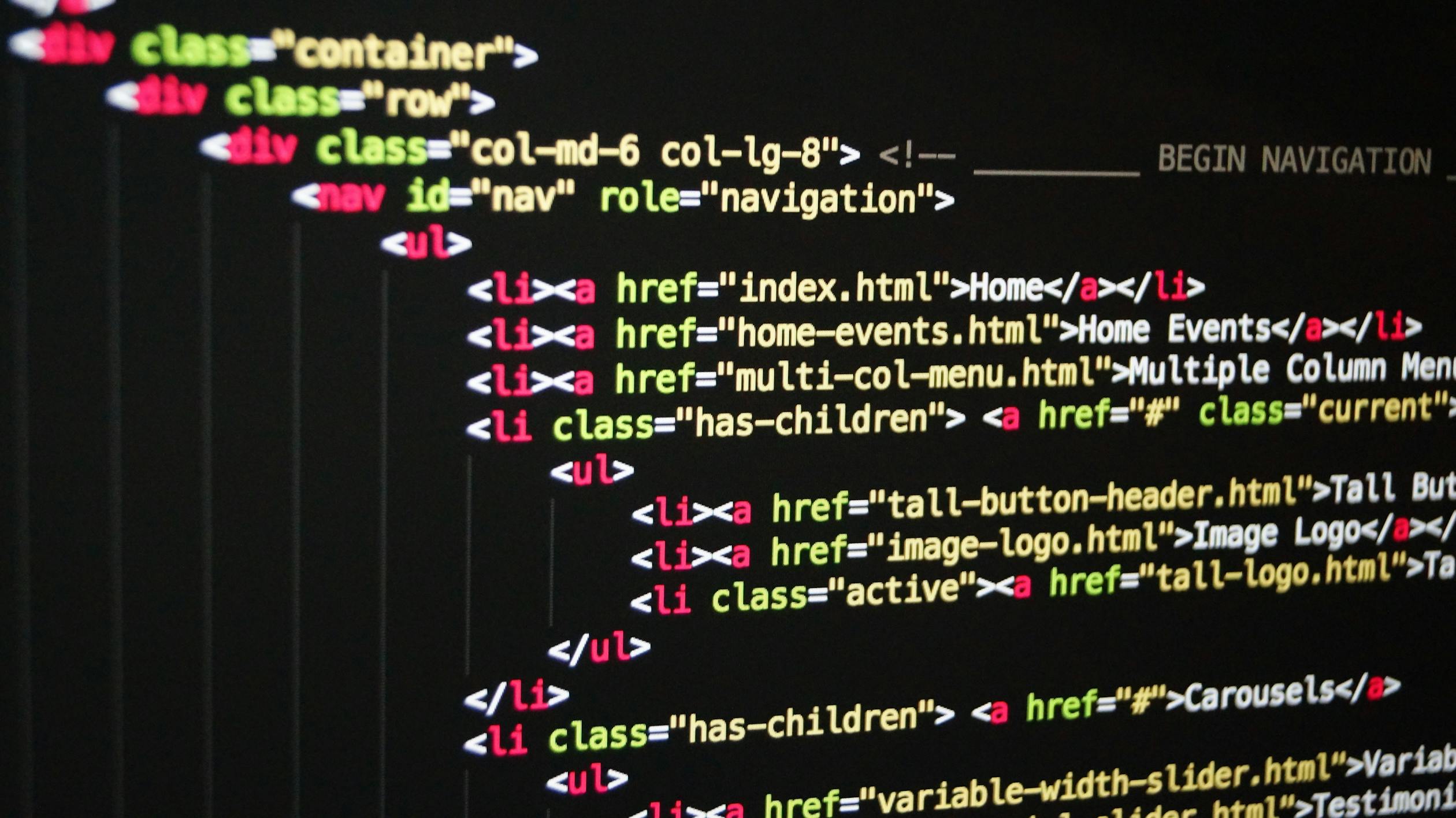
PHP classes are an essential feature of object-oriented programming (OOP) in PHP. They allow you to structure your code in a more organized and reusable way. In this tutorial, we'll cover the basics of creating and using classes in PHP.
Creating a Class
To define a class in PHP, use the class keyword followed by the class name. Here's a simple example:
<?php
class Car {
// Properties
public $brand;
public $model;
// Methods
public function displayInfo() {
echo "This is a {$this->brand} {$this->model}.";
}
}
?>
In this example, we've created a class named Car with two properties ($brand and $model) and one method (displayInfo()).
Creating an Object
Once you've defined a class, you can create an object (an instance of the class) using the new keyword:
<?php
// Create an object of the Car class
$myCar = new Car();
// Set object properties
$myCar->brand = "Toyota";
$myCar->model = "Camry";
// Call a method
$myCar->displayInfo();
?>
Now, $myCar is an instance of the Car class with the specified properties.
Constructors and Destructors
Constructors and destructors are special methods that are called when an object is created and destroyed, respectively. You can define them in your class like this:
<?php
class Car {
public $brand;
public $model;
// Constructor
public function __construct($brand, $model) {
$this->brand = $brand;
$this->model = $model;
}
// Method
public function displayInfo() {
echo "This is a {$this->brand} {$this->model}.";
}
// Destructor
public function __destruct() {
echo "The {$this->brand} {$this->model} has been destroyed.";
}
}
// Create an object with constructor parameters
$myCar = new Car("Ford", "Mustang");
// Call a method
$myCar->displayInfo();
?>
Inheritance
PHP supports class inheritance, allowing you to create a new class that is a modified version of an existing class. Here's a simple example:
<?php
class SportsCar extends Car {
public $topSpeed;
public function displayInfo() {
parent::displayInfo();
echo " It can reach a top speed of {$this->topSpeed} mph.";
}
}
// Create an object of the SportsCar class
$sportsCar = new SportsCar("Ferrari", "458 Italia");
$sportsCar->topSpeed = 210;
// Call the overridden method
$sportsCar->displayInfo();
?>
In this example, SportsCar inherits from the Car class and overrides the displayInfo() method.
Summary
This short tutorial covers the basics of PHP classes, including class creation, object instantiation, constructors/destructors, and inheritance. As you continue to explore object-oriented programming in PHP, you'll discover more advanced features and techniques to enhance your code organization and reusability.
Happy coding!
540 views