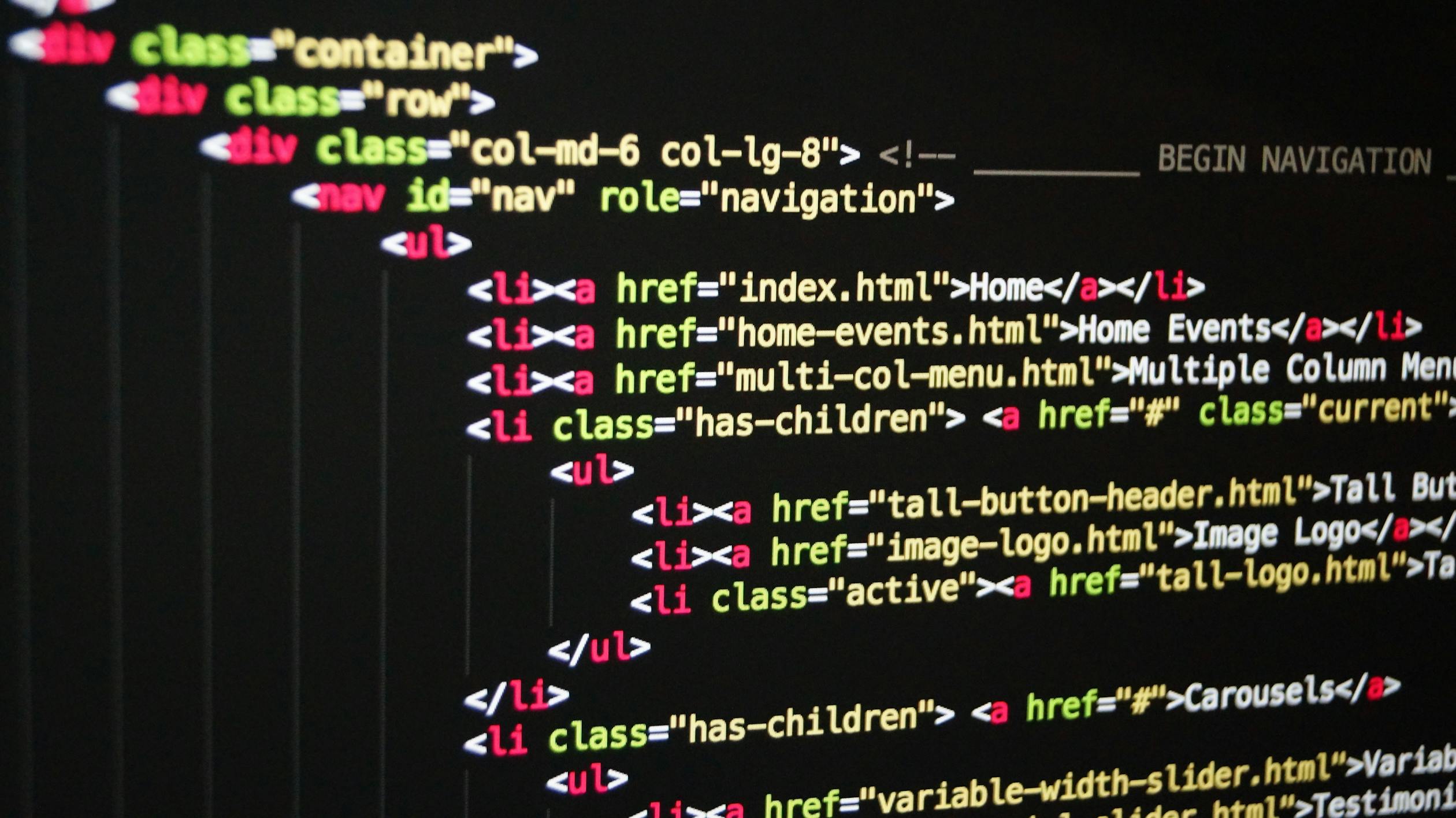
November 08, 2023
A Beginner's Guide to Classes in C# - Building a Banking Application
IntroductionClasses are one of the fundamental building blocks of object-oriented programming in C#. They allow you to define the blueprint for objects, encapsulating data and behavior. In this beginner's guide, we will create a simple C# project that defines and tests a BankAccount class, demonstrating the concept of classes, properties, and methods. By the end of this blog, you will have a better understanding of how to create and use classes in C#.
Creating a New C# Project
Before we dive into the code, let's set up a new C# project. You can use Visual Studio, Visual Studio Code, or any other C# development environment of your choice. Follow these steps to create a new project:
- Open your development environment and create a new C# project.
- Name your project (e.g., "BankAccountDemo").
- Once your project is set up, you should have a default Program.cs file. This is where we'll write our code.
Defining the BankAccount Class
Now, let's define the BankAccount class, which will represent a simple bank account with a balance and an account holder's name. Create a new class in the Program.cs file:
class BankAccount
{
public string Name { get; set; }
public decimal Balance { get; private set; }
public BankAccount(string name, decimal initialBalance)
{
Name = name;
Balance = initialBalance;
}
}
The method BankAccount is known as the constructor. The constructor is automatically called by Common Runtime Language (CLR) when an object of a class is created to initialize all the class data members.
Let's expand our class to add two essential methods that will carry out activities that every bank account does; Withdrawal and Deposit.
class BankAccount
{
public string Name { get; set; }
public decimal Balance { get; private set; }
public BankAccount(string name, decimal initialBalance)
{
Name = name;
Balance = initialBalance;
}
public void Deposit(decimal amount)
{
if (amount > 0)
{
Balance += amount;
Console.WriteLine($"Deposited {amount:C} into {Name}'s account. New balance: {Balance:C}");
}
}
public void Withdraw(decimal amount)
{
if (amount > 0 && amount <= Balance)
{
Balance -= amount;
Console.WriteLine($"Withdrawn {amount:C} from {Name}'s account. New balance: {Balance:C}");
}
else
{
Console.WriteLine("Invalid withdrawal amount or insufficient balance.");
}
}
}
Now, let's create an instance of the BankAccount class and test its methods in the Main method
class Program
{
static void Main()
{
BankAccount account = new BankAccount("John Doe", 1000);
Console.WriteLine($"{account.Name}'s initial balance: {account.Balance:C}");
account.Deposit(500);
account.Withdraw(200);
account.Close();
Console.ReadKey(); // Keep the console window open
}
}
In this code, we create an instance of the BankAccount class, perform some transactions, and then close the account. We also display the initial balance and any changes made.
Conclusion
Congratulations! You've now learned the basics of creating and using classes in C#. The BankAccount class serves as a simple example, but in real-world applications, classes can become much more complex, encapsulating data and behaviors to create powerful and organized code. Classes are the foundation of object-oriented programming and are used extensively in C# for building various types of applications.
As you continue your journey in C# programming, you'll encounter more complex classes and objects, enabling you to build sophisticated software. Stay curious, explore new concepts, and keep practicing to become a proficient C# developer. Happy coding
389 views