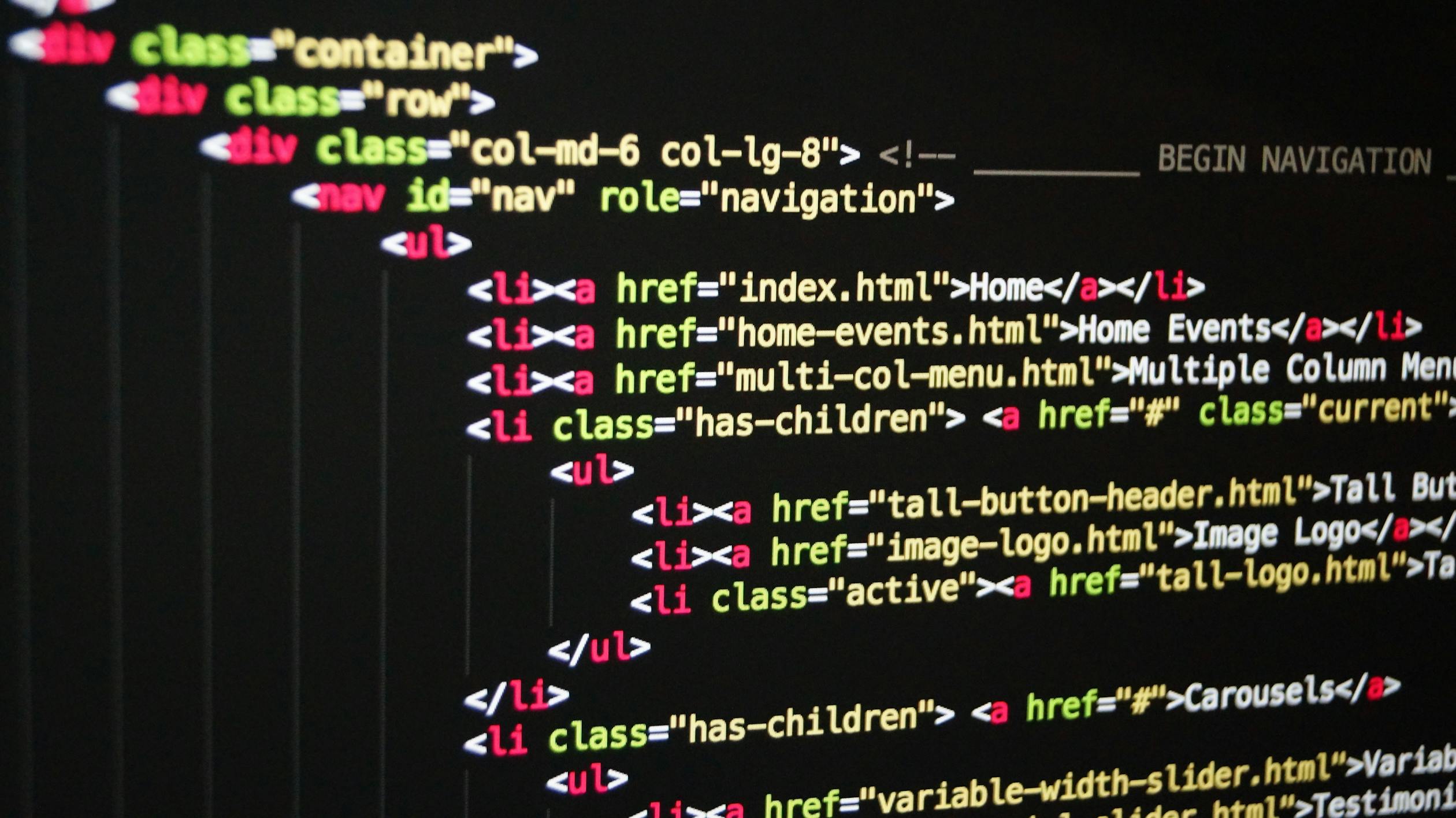
IntroductionMemory management is a crucial aspect of programming in C#, and understanding the concepts of the stack and heap is fundamental to efficiently managing memory in your applications. In this blog, we will explore these two memory areas and provide examples to help you grasp their significance.
The Stack
The stack is a region of memory used for storing value types and managing method calls and local variables. It operates in a Last-In-First-Out (LIFO) manner, which means the most recently added item is the first to be removed
Example 1: Storing Value Types on the Stack
int a = 10; // Allocating an integer variable 'a' on the stack
int b = 20; // Allocating another integer variable 'b' on the stack
int result = a + b; // Perform an operation on stack variables
In the example above, the integer variables a, b, and result are allocated on the stack. When they go out of scope, the memory is automatically released.
The stack is highly efficient for managing local variables but has limited capacity and doesn't support dynamic memory allocation. Therefore, objects with unknown or variable lifetimes should not be stored on the stack.
The Heap
The heap is another region of memory used for storing reference types, which include objects and class instances. Unlike the stack, memory allocated on the heap does not have a fixed lifetime and must be explicitly managed. The garbage collector takes care of this by automatically freeing memory when objects are no longer reachable.
class Student
{
public string Name;
public int Age;
}
Student student1 = new Student(); // Allocate a Student object on the heap
student1.Name = "Alice";
student1.Age = 20;
Student student2 = student1; // Create a reference to the same object
student1 = null; // Set the first reference to null
// The object is still accessible through 'student2'
Console.WriteLine(student2.Name); // Outputs "Alice"
In this example, a Student object is created on the heap using the new keyword. The variable student1 references this object. When student1 is set to null, the object is not immediately destroyed but becomes eligible for garbage collection. The memory will be freed by the garbage collector when no references point to the object.
The heap is suitable for objects with dynamic lifetimes and those that need to be shared between different parts of your program. However, improper memory management on the heap can lead to memory leaks if references are not properly cleaned up.
Conclusion
Understanding the stack and heap in C# memory management is essential for writing efficient and reliable code. Value types are typically stored on the stack with automatic memory management, while reference types are allocated on the heap, where the garbage collector takes care of freeing memory when it's no longer in use. Properly managing memory on the stack and heap ensures your C# applications run smoothly and efficiently while avoiding memory-related issues.
354 views