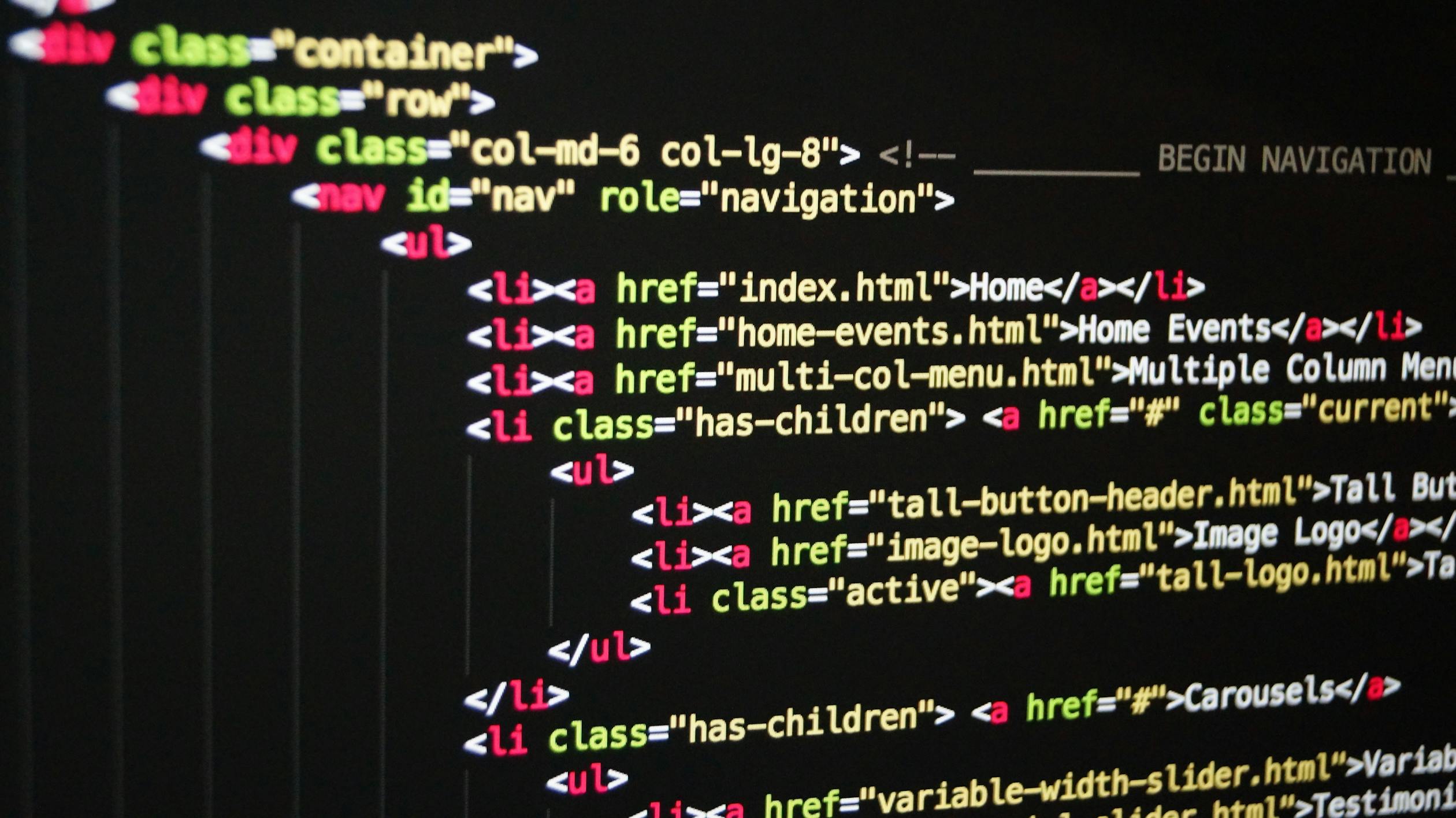
November 03, 2023
Deploying an Angular/React Website to AWS S3 Using GitLab CI/CD
In today's fast-paced development environment, automating the deployment of web applications is essential. This blog post will guide you through deploying an Angular or React website to Amazon S3 using GitLab CI/CD. We'll focus on configuring GitLab variables securely and streamlining the deployment process to ensure a smooth and efficient workflow.
1. Setting Up Your Development Environment
Before we dive into the deployment process, it is crucial to set up your development environment correctly. Follow these steps:
Prerequisites
Ensure you have the following prerequisites for deploying an Angular or React website:
- Node.js and NPM installed.
- A GitLab repository for your project.
- An AWS account with the AWS CLI installed.
Generating the dist Folder
1. Angular
If you are working with Angular, follow these steps to generate the dist folder:
- Open your terminal and navigate to your Angular project's root directory.
- Run the following command to build your Angular application for production:
ng build --prod
This command compiles your Angular code and generates the production-ready files in the dist/ directory within your project.
2. React
For React projects, follow these steps to create the dist folder:
Open your terminal and navigate to your React project's root directory.
Run the following command to build your React application for production:
npm run build
This command compiles your React code and creates the optimized production build in the build/ directory within your project.
2. GitLab Pipeline Configuration
GitLab CI/CD pipelines are powerful tools for automating tasks like building and deploying applications. Let's explore how to set up your pipeline:
Understanding GitLab CI/CD
GitLab CI/CD is an integrated tool that allows you to automate the building, testing, and deployment of your code. At its core, a CI/CD pipeline consists of stages that run sequentially:
- Test: Run tests to ensure your code functions correctly.
- Build: Build your application for production.
- Deploy: Deploy your application to your desired hosting environment.
Sample .gitlab-ci.yml Configuration
Below is a sample .gitlab-ci.yml configuration for deploying your Angular or React website. It defines three stages: test, build, and deploy.
stages:
- test
- build
- deploy
variables:
# Define your variables here
test:
stage: test
script:
# Add your testing commands here
build:
stage: build
script:
# Add your build commands here
deploy:
stage: deploy
script:
# Add your deployment commands here
3. Defining Variables Securely
To keep sensitive information, such as API keys or AWS access credentials, secure in your GitLab CI/CD pipeline, you have two options:
Option 1: Define Variables in .gitlab-ci.yml
You can define variables directly within the .gitlab-ci.yml file. However, this is less secure because the variables are exposed in the repository.
variables:
S3_BUCKET_NAME: 'my-s3-bucket'
AWS_ACCESS_KEY_ID: 'aws_access_key_id'
AWS_SECRET_ACCESS_KEY: 'aws_secret_access_key'
Option 2: Define Variables in GitLab Project Settings (Recommended)
A more secure approach is to define variables in the GitLab project settings:
- Go to your GitLab project's main page.
- Navigate to Settings > CI/CD > Variables.
- Add your variables with their values.
To reference a variable in your .gitlab-ci.yml file, use the $VARIABLE_NAME syntax, where VARIABLE_NAME is the name of the variable you defined.
variables:
MY_VARIABLE: 'some_value'
stages:
- build
build:
stage: build
script:
- echo "My variable's value is: $MY_VARIABLE"
4. AWS Deployment to S3
With your GitLab CI/CD pipeline configured and your variables securely defined, it is time to deploy your website to AWS S3. Follow these steps:
Deploying to Amazon S3
- Create an S3 Bucket: Log in to your AWS Management Console, navigate to the S3 service, and create a new bucket with a unique name.
- Configure for Static Website Hosting: In the S3 bucket properties, go to the "Static website hosting" section. Enable static website hosting and specify your index document (e.g., index.html) and error document (optional).
- Set Public Access Permissions: To make your website publicly accessible, configure the bucket policy. Here is an example policy:
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicReadGetObject",
"Effect": "Allow",
"Principal": "*",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
}
]
}
- Replace <bucket-name> with your S3 bucket name.
- Upload Your Website: In your terminal, navigate to the directory containing your Angular or React project's dist folder. Use the AWS CLI to upload the files to your S3 bucket:
aws s3 sync dist/ s3://<bucket-name>/
Replace <bucket-name> with your S3 bucket name.
Conclusion
In this comprehensive guide, we've explored the process of deploying an Angular or React website to Amazon S3 using GitLab CI/CD. By following these steps, you can streamline your development workflow, automate deployments, and ensure the security of sensitive information.
Now that you have the knowledge and tools, take your web development projects to the next level with automated, secure, and efficient deployments.
455 views