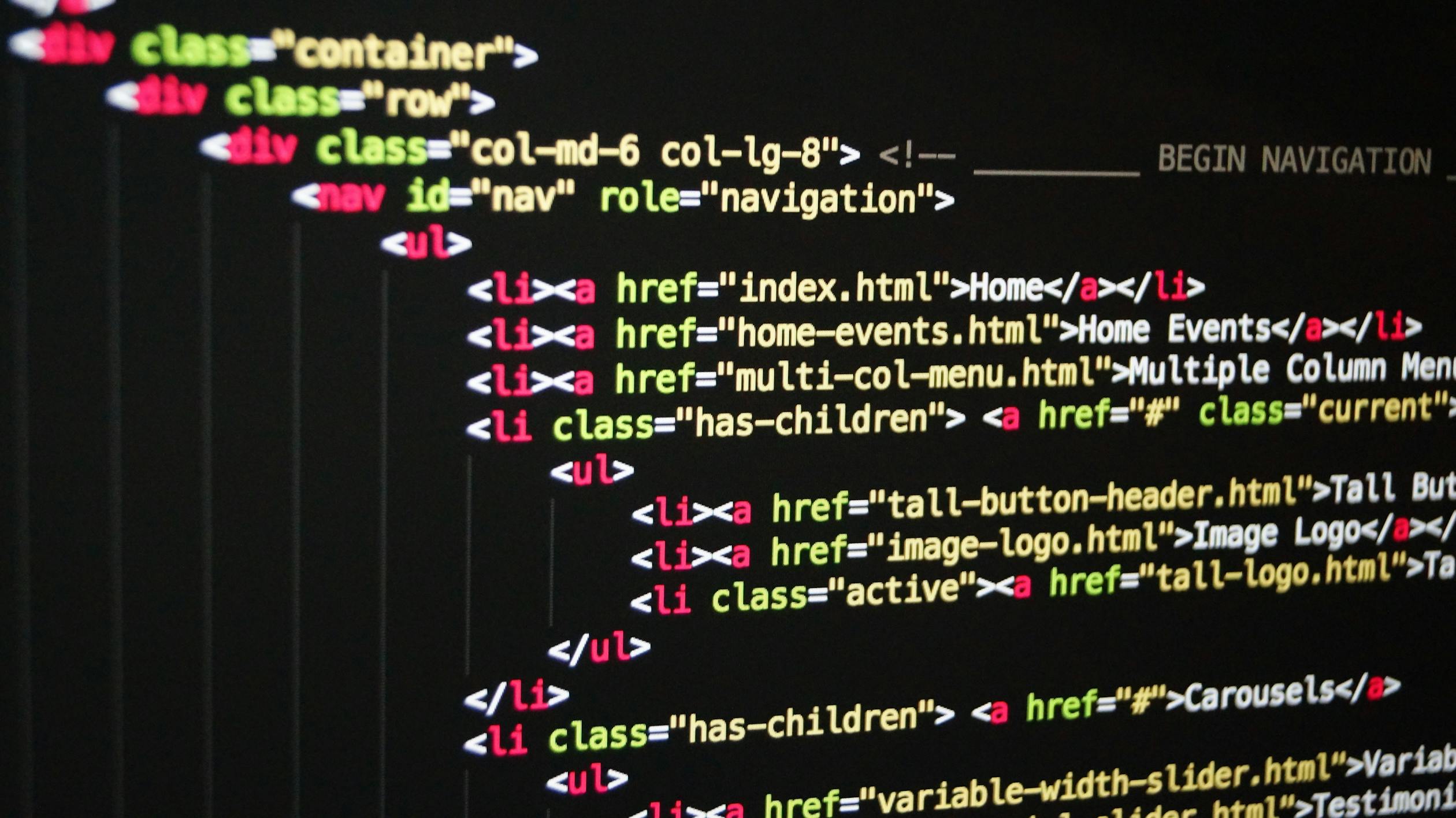
November 21, 2023
Poly-more-phism with classes and inheritance
My first post on the topic of classes introduced the classes and objects in Python. This article will expand on that by introducing inheritance and polymorphism.
Purpose
This article will explore class inheritance and polymorphism in Python via a simple example.
Audience
This article will appeal to newbies and learners.
Prerequisites
- Python interpreter
- some knowledge of classes in Python - here is a good place to start
Introduction
The word 'polymorph' comes from the Greek words 'poly' (many) and 'morph' (shape). In programming, it means our classes, operators (e.g., +) and functions can take different shapes - or do different things - depending on how they are used. For example, multiple classes can have the same method name, but execute different code. This becomes useful, especially with classes.
Classes are object-orientated programming's cornerstone. They allow us to neatly package attributes and behaviours into a named thing and to create as many copies of that thing as we like. But classes to a step further by allowing us to take any class we want and to define other classes based on the original class - this is called a 'subclass'.
Thus, we can define an entire taxonomy of classes that inherit member variables and functions from their parents, implement their own unique attributes and behaviours, and have features unique to them.
For example, all animals do an action called eat, but only birds can 'fly', and among birds, only hummingbirds can fly backwards.
Let's explore this with an example.
Step-by-step
Create a base class
- in a new file (e.g., called oop.py), create a class called 'Animal'. It should have one member function called 'eat' and a member variable called 'name'.
class Animal:
name = 'none'
def eat(self):
print('Nom nom')
def __init__(self, name):
self.name = name
Now, we want to create a class for birds. It would be handy if we did not have to create a 'Bird' class with its own 'name' variable and 'eat' function. Let's see how we can achieve this with subclasses. To define a subclass, we specify its parent class in parenthesis after the class name.
Create a subclass
- add a subclass to oop.py
class Animal:
name = 'none'
def eat(self):
print('Nom nom')
def __init__(self, name):
self.name = name
class Bird(Animal):
def fly(self):
print("Up and away!")
- Let's test our program - add these lines to the end of oop.py - now run your program
animal_1 = Animal("jeff")
animal_1.eat()
bird_1 = Bird("polly")
bird_1.eat()
bird_1.fly()
Nom nom
Nom nom
Up and away!
Notice that we did not need to define a function called 'eat' in our Bird subclass. The Bird subclass inherited it from Animal!
Define some more animals
- add more classes to oop.py
class Animal:
name = 'none'
def eat(self):
print('Nom nom')
def __init__(self, name):
self.name = name
class Bird(Animal):
def fly(self):
print("Up and away!")
class Hummingbird(Bird):
def eat(self):
print("Mmmm... bugs!")
def fly_backwards(self):
print("Look what I can do!")
class Bear(Animal):
pass
class Grizzly(Bear):
def eat(self):
print("Mmmm... honey!")
We have created a Hummingbird subclass that is a child class of Bird. We have also set up Bear - which is an animal - and Grizzly, which is a bear.
- add these lines and run your program
grizzly = Grizzly("Big")
hummy = Hummingbird("Tiny")
grizzly.eat()
hummy.eat()
Mmmm... honey!
Mmmm... bugs!
Amazing. Let's take this a step further.
- remove the last two lines from above and replace as follows:
for animal in (grizzly, hummy):
animal.eat()
In our 'for' loop, we create a variable called 'animal' and we invoke its 'eat' function. The interpreter executes the correct function, depending on the 'animal'. So our 'animal' can take many shapes - that's polymorphism!
646 views