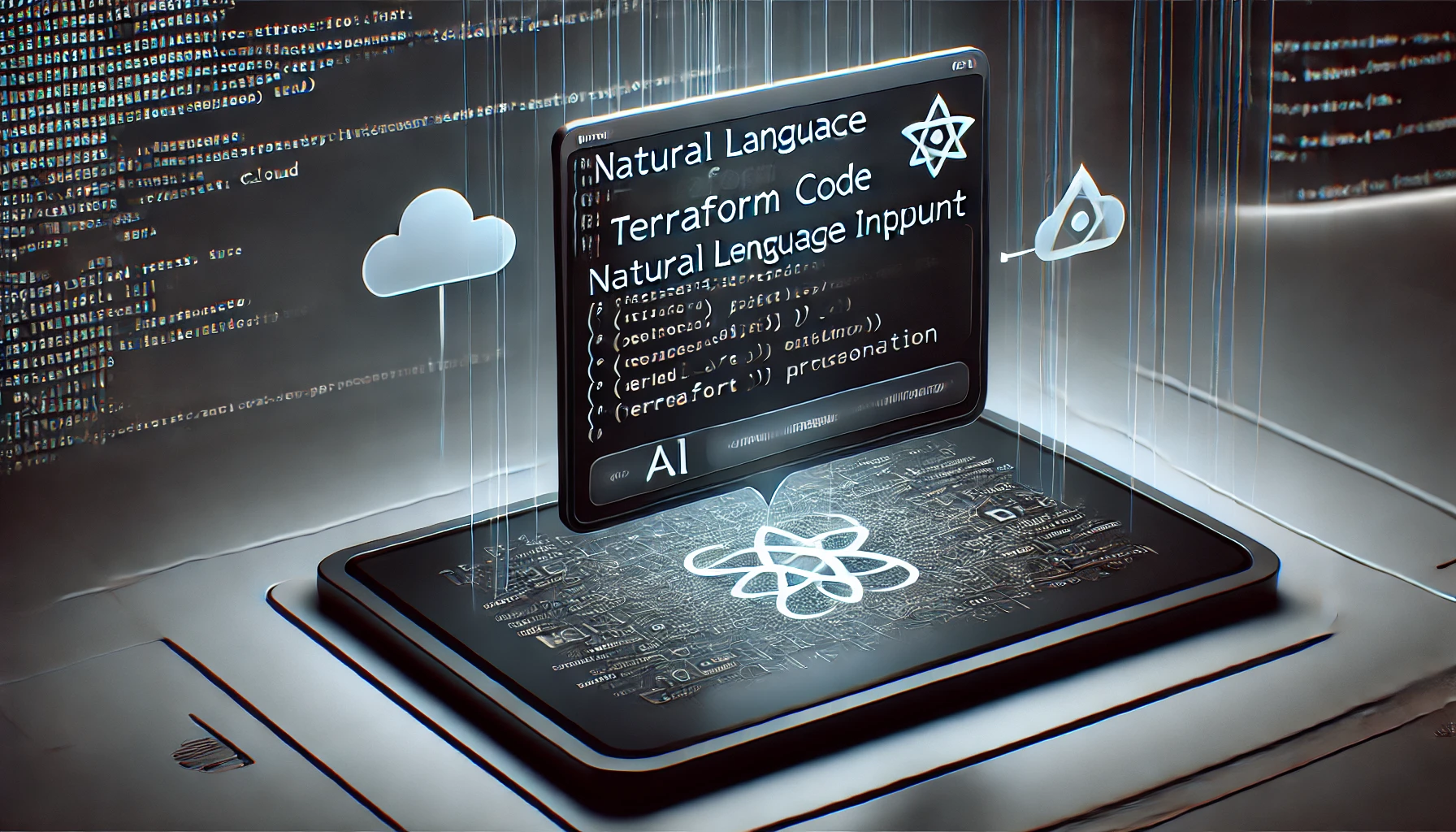
April 24, 2025
Creating a Natural Language to Terraform Converter with DeepSeek
Writing Infrastructure-as-Code (IaC) for advanced cloud installations is hard. I spend hours creating Terraform configurations, double-checking syntax, and debugging issues as a developer. What if we described the infrastructure in simple English and let AI handle the rest?
I attempted that using DeepSeek, an AI-powered code generation model that converts natural language descriptions into Terraform configurations. Instead of manually building Terraform scripts, DeepSeek can comprehend our needs and produce completely working infrastructure code in seconds.
This blog shows how to use DeepSeek to develop a Natural Language to Terraform Converter. In this article, I'll discuss how to take user input, making dynamic Terraform code, validating output, and using configurations. And you'll see a prototype for AI-automated infrastructure deployment in the end.
What is Terraform and Its Role in IaC
Let's learn why Terraform is the IaC tool of choice before getting into DeepSeek. The open-source Terraform tool lets developers create infrastructure using declarative configuration files. Implementing resources as code allows version control, consistency, and repeatability.
I would write this to build an AWS EC2 instance using Terraform:
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "my_server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
You need to know about Terraform code, cloud tools, and setup choices in order to use this way effectively. What if I could tell an AI to "deploy a t2.micro EC2 machine in AWS" and it would do it? DeepSeek is here.
Introduction to DeepSeek
More advanced AI model DeepSeek turns natural language prompts into code. It is like ChatGPT, but it is better for writing code. It lets us turn simple English statements into well-organized Terraform setups.
Terraform's HCL (HashiCorp Configuration Language) is one of the programming languages DeepSeek knows. We can turn what users type into Terraform code with DeepSeek and a simple Python tool.
Building a Natural Language to Terraform Converter
1. Configure DeepSeek
Here we'll need DeepSeek's API key, but if you have it, then its a bonus we can dirently start by installing Python libraries:
pip install openai requests
Here's how you can connect with DeepSeek:
import openai
openai.api_key = "your_deepseek_api_key"
def generate_terraform_code(prompt):
response = openai.ChatCompletion.create(
model="deepseek-coder",
messages=[{"role": "user", "content": prompt}]
)
return response["choices"][0]["message"]["content"]
user_prompt = "Create an AWS EC2 instance with 2 vCPUs and 4GB RAM."
terraform_code = generate_terraform_code(user_prompt)
print(terraform_code)
DeepSeek receives natural language input from this script. The model returns the Terraform code after processing the request.
2. Designing the Input Processing System
We should evaluate and gather essential factors for better results before delivering user input to DeepSeek. SpaCy for Named Entity Recognition (NER) can deduce the characteristics of cloud services, instance types, regions, and more.
pip install spacy
python -m spacy download en_core_web_sm
import spacy
nlp = spacy.load("en_core_web_sm")
def extract_parameters(text):
doc = nlp(text)
entities = {ent.label_: ent.text for ent in doc.ents}
return entities
user_input = "Create an AWS S3 bucket named 'my-app-bucket' with public read access."
parameters = extract_parameters(user_input)
print(parameters)
3. Generating Terraform Code with DeepSeek
We may enhance Terraform configurations by enhancing our API request after obtaining key parameters.
def generate_terraform_code(resource_type, resource_name, attributes):
prompt = f"Generate Terraform code for a {resource_type} named '{resource_name}' with the following attributes: {attributes}"
return generate_terraform_code(prompt)
terraform_script = generate_terraform_code("AWS S3 Bucket", "my-app-bucket", {"acl": "public-read"})
print(terraform_script)
4. Validating and Applying Terraform Code
Now we can start applying Terraform scripts from DeepSeek after validating it. After that, run the Terraform commands below result saving into an a.tf file:
terraform init
terraform plan
terraform apply -auto-approve
Such as, suppose DeepSeek creates Terraform code like this:
resource "aws_s3_bucket" "my_app_bucket" {
bucket = "my-app-bucket"
acl = "public-read"
}
We can save it under s3_bucket.tf and run Terraform CLI.
terraform apply -auto-approve
Terraform will provision the S3 bucket as specified.
Example: Converting Natural Language to Terraform Code
Try our system using a real-world example. Should the user write "Deploy an AWS EC2 instance in us-West-2 with a t3.medium instance type."
DeepSeek should create the Terraform script shown here:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "my_instance" {
ami = "ami-0abcdef1234567890"
instance_type = "t3.medium"
}
Terraform can deploy the instance using this output.
Challenges and Optimization
This method works well but has some challenges too. Validate DeepSeek output as it may provide slightly inaccurate configurations. Uncertain user inputs may need a fallback mechanism or user confirmation.
A Terraform dataset may assist fine-tune DeepSeek for accuracy. One more option is a verification mechanism that runs produced codes against Terraform's schema.
Future Improvements and Use Cases
To further enhance this project, you can:
- Test DeepSeek's handling of different cloud providers, including Azure and Google Cloud.
- Built a real-time chatbot for dynamic Terraform settings.
- Building a web-based Terraform file download and request input interface.
Conclusion
Automating Terraform code creation using DeepSeek is interesting for infrastructure provisioning availability. Instead of spending hours developing and debugging Terraform scripts, we can now define our needs in normal language and let AI do the rest.
Through DeepSeek, NLP, and Terraform, we created a powerful cloud automation tool. AI-powered IaC tools will only grow smarter, speeding up and improving cloud administration.
Try DeepSeek for Terraform projects. Let AI do the hard work while you innovate!
259 views