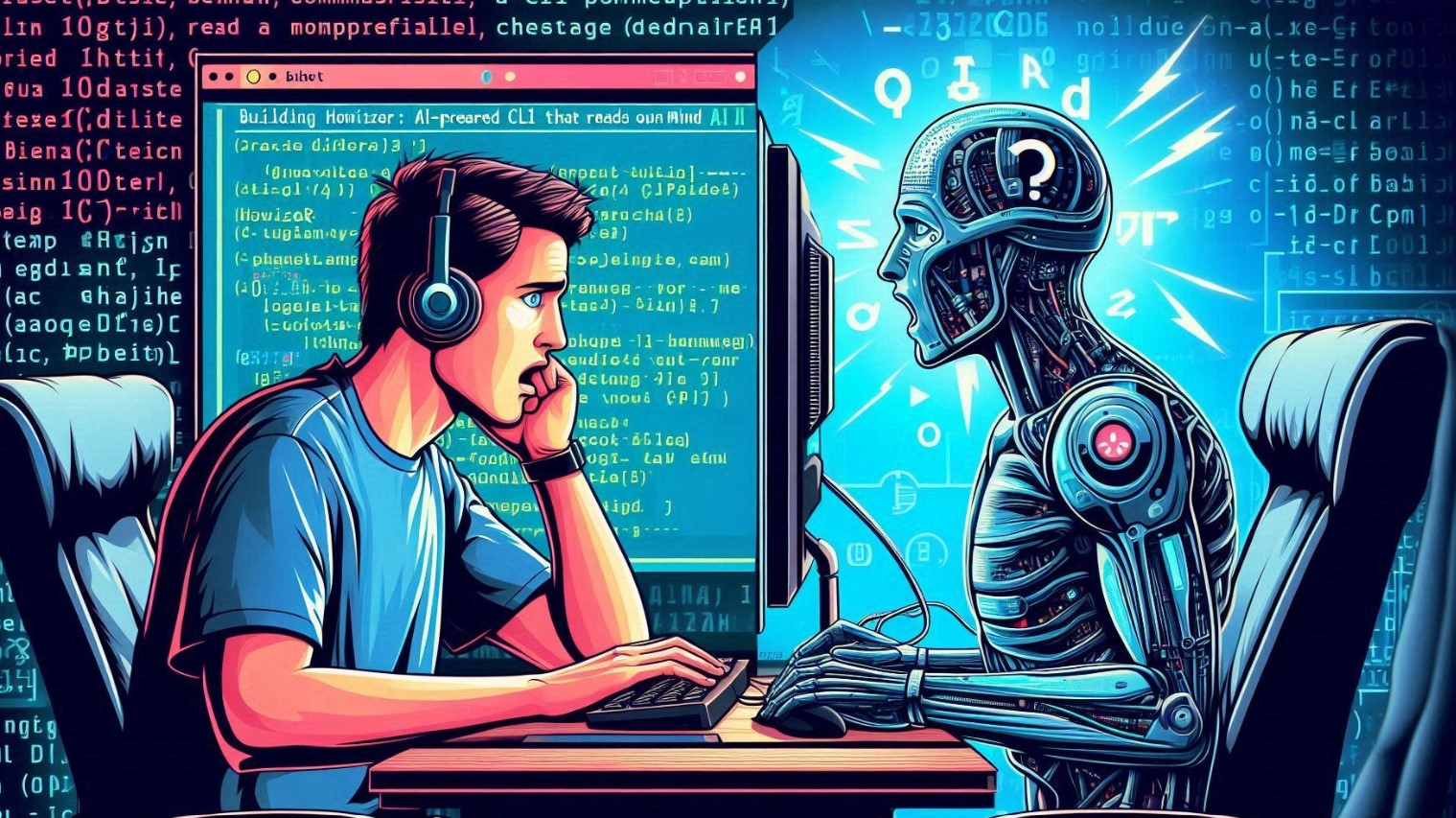
April 14, 2025
Building a Custom AI-Powered CLI Tool with DeepSeek
Have you ever had a hard time with code, looked for answers on Stack Overflow, or spent hours fixing a bug? Yes, I have. Think about having an AI-powered helper in your computer that could write, fix, and explain code in real time. Today, we will construct a DeepSeek-powered AI-driven CLI utility.
DeepSeek works great with this tool because it can generate and understand complex code. This command line tool will change the way you write and fix code, making your work faster whether you are a beginner or an experienced coder.
Why Use DeepSeek for a CLI Assistant?
DeepSeek is for developers. DeepSeek gives correct code suggestions, fixing help, and explanations in the computer language you are using, unlike most AI models that only answer general questions.
I have tried a few different AI-powered writing tools, but DeepSeek is the fastest, most accurate, and best at understanding difficult code logic. This CLI assistant keeps everything in your terminal instead of browser tabs or auto-complete suggestions.
Setting Up Your Development Environment
Install Python 3+ before coding. Click is a Python library that facilitates CLI application development, and we will use DeepSeek API calls.
The following terminal command installs the necessary dependencies:
pip install click requests
Create a CLI tool Python file after installing dependencies.
touch ai_cli.py
Now, open this file in your chosen code editor, and we'll start developing the CLI utility from scratch.
Initializing the CLI Structure
The first step is to use Click to build the CLI structure and load the necessary libraries.
import click
import requests
After this, choose the endpoint and authentication key for the DeepSeek API. Replace "your_deepseek_api_key" with your API key.
API_KEY = "your_deepseek_api_key"
API_URL = https://api.deepseek.com/generate
We will develop a DeepSeek API request function to modularize our CLI utility. It takes a prompt, makes an API call, and returns the answer.
def call_deepseek(prompt):
"""Send a request to DeepSeek API and return the response."""
response = requests.post(
API_URL,
json={"prompt": prompt, "max_tokens": 200},
headers={"Authorization": f"Bearer {API_KEY}"}
)
if response.status_code == 200:
return response.json().get("text", "No response received.")
else:
return f"Error: {response.status_code}, {response.text}"
CLI commands will be defined using Click. Click organizes CLI functions elegantly; therefore, let us build a command group.
@click.group()
def cli():
"""AI-Powered CLI Tool using DeepSeek"""
pass
Implementing Code Generation
We will start with code creation. The CLI generates code from a text prompt (e.g. Python program for quicksort).
@click.command()
@click.argument("query")
def generate(query):
"""Generate code from a prompt."""
click.echo("Generating code...")
result = call_deepseek(f"Write a {query} function in Python.")
click.echo(result)
How this command works:
- The @click.command() decorator creates a CLI command.
- The @click.argument("query") decorator enables a text prompt during command execution.
- The call_deepseek method requests code creation from DeepSeek.
Now it's time to add this command to our CLI tool:
cli.add_command(generate)
To test this function, run:
python ai_cli.py generate "quick sort algorithm"
DeepSeek should yield a Python Quicksort implementation.
Implementing Debugging Feature
Implement code debugging next. This feature analyses a code fragment and suggests improvements using DeepSeek.
@click.command()
@click.argument("code")
def debug(code):
"""Debug a given code snippet."""
click.echo("Analyzing code for errors...")
result = call_deepseek(f"Debug this code and suggest fixes:\n{code}")
click.echo(result)
Although similar to create, this method changes the API prompt to emphasize debugging.
We also need to register this command:
cli.add_command(debug)
To test the debugging feature, run:
python ai_cli.py debug "def add_numbers(a, b): return a + c"
DeepSeek should see c is undefined and propose a solution.
Implementing Code Explanation
The last part is the code explanation. Users may enter a code fragment to get a plain English explanation.
@click.command()
@click.argument("code")
def explain(code):
"""Explain what a code snippet does."""
click.echo("Explaining code...")
result = call_deepseek(f"Explain this code in simple terms:\n{code}")
click.echo(result)
This method requests DeepSeek describe the code snippet in simple words.
We add this command to our CLI tool:
cli.add_command(explain)
To test it, run:
python ai_cli.py explain "def factorial(n): return 1 if n==0 else n * factorial(n-1)"
DeepSeek should explain recursive factorial.
Running and Testing the CLI Tool
A completely working AI-powered CLI tool now works. Our script currently includes:
- A CLI group (cli()) groups commands.
- Generate, debug, and explain instructions for AI-driven coding jobs.
- The call_deepseek() method connects to the DeepSeek API.
To try our CLI tool, run:
python ai_cli.py -- help
This should show all commands. You may now produce, debug, and explain code from your terminal.
Packaging the CLI Tool for Easy Installation
Create a setup.py file in the same directory to install the CLI tool easily:
from setuptools import setup, find_packages
setup(
name="deepseek_cli",
version="1.0",
py_modules=["ai_cli"],
install_requires=["click", "requests"],
entry_points={
"console_scripts": [
"deepseek-cli=ai_cli:cli",
],
},
)
Now, install it locally using:
pip install --editable .
You can now use the CLI tool globally by running:
deepseek-cli generate "fibonacci sequence"
Conclusion and Next Steps
Our AI-powered CLI tool generates, debugs, and explains code using DeepSeek. This tool brings AI-powered coding help to the terminal, eliminating the need to seek online.
In future stages, consider adding:
- Support for several programming languages
- Advanced debugging with potential fixes
- Automatic execution of created code snippets
AI-powered development tools are the future, and this CLI helper is a wonderful start.
85 views