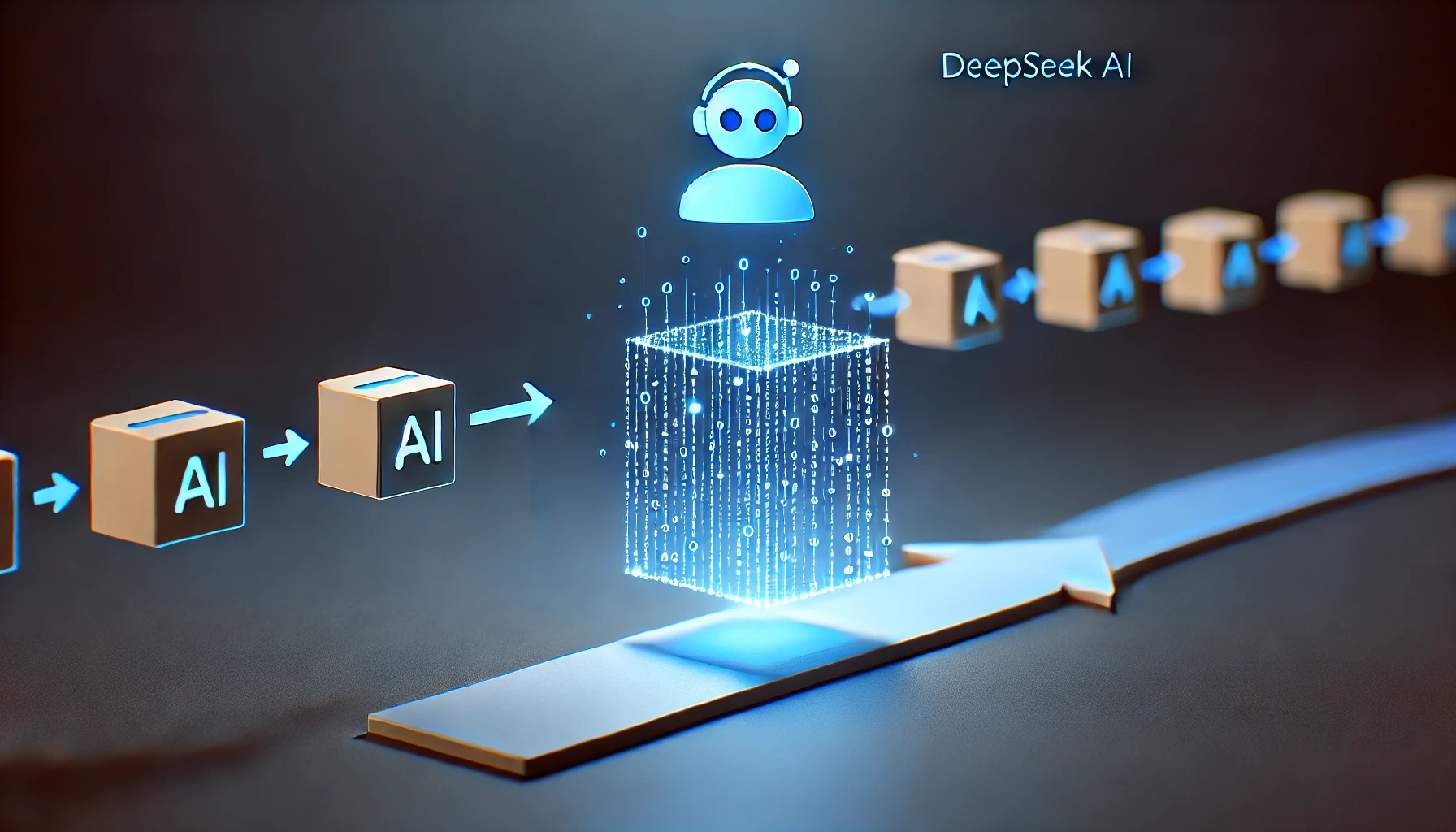
April 09, 2025
Developing an AI-Powered Code Migration Tool with DeepSeek
Moving code across programming languages has always been laborious and error-prone. I have stared at hundreds of lines of old Python code that required Rust rewrites for improved performance. Do you do it manually? That would take hours or days. Let's not even mention the danger of adding bugs.
Here come AI-powered solutions like DeepSeek. Automating code conversion using DeepSeek reduces human labor and ensures correct translations. To smoothly convert Python functions into Rust with a few lines of AI-generated recommendations seems miraculous. This post will explain how to make that magic happen.
Why Code Migration Matters
Businesses and developers move code for several reasons. Sometimes, it is about ditching old tech. Rust outperforms Python in memory safety and speed. Security is another priority. Migration is necessary to reduce danger since certain older languages lack newer safety measures.
Doing it manually is not fun. This long and error-prone process needs a lot of knowing both the source and target languages. AI-powered solutions are changing the game by letting programmers improve code instead of writing it from scratch.
Setting Up DeepSeek for Code Migration
DeepSeek is easy to use. If you have Python, importing DeepSeek and using it to produce translated code is simple.
First, install DeepSeek if you havent already:
pip install deepseek
You can translate Python code to different languages with a few lines of script after installation.
Converting Python Code to Rust Using DeepSeek
Let's adapt a basic Python code to Rust to show DeepSeek. The Python method below outputs "Hello, world!"
from deepseek import DeepSeekCode
python_code = """
def greet():
print("Hello, world!")
"""
response = DeepSeekCode.generate(f"Convert this Python code to Rust: {python_code}")
print(response)
DeepSeek analyzes the function and returns the Rust equivalent, which looks something like this:
fn greet() {
println!("Hello, world!");
}
Though simple, it illustrates how well DeepSeek handles direct translations.
Handling More Complex Code Migrations
Let's go one step further. What if we need to relocate a function that processes input and returns a result? Consider this Python method that sums a list:
python_code = """
def sum_numbers(numbers):
return sum(numbers)
"""
response = DeepSeekCode.generate(f"Convert this Python code to Rust: {python_code}")
print(response)
DeepSeek generates the Rust equivalent, which might look like this:
fn sum_numbers(numbers: &[i32]) -> i32 {
numbers.iter().sum()
}
It properly detects that the function processes a list and defines numbers as an array of integers for type safety. AI-powered migration excels in code intent understanding and exact conversions.
Automating Code Migration with DeepSeek
It is hard to manually convert each function for bigger applications. The best method is automation. Batch-process and migrate scripts automatically using DeepSeek in a CI/CD pipeline.
The following Python script converts numerous Python files to Rust:
import os
from deepseek import DeepSeekCode
directory = "python_scripts"
output_directory = "converted_rust"
os.makedirs(output_directory, exist_ok=True)
for filename in os.listdir(directory):
if filename.endswith(".py"):
with open(os.path.join(directory, filename), "r") as file:
python_code = file.read()
response = DeepSeekCode.generate(f"Convert this Python code to Rust: {python_code}")
with open(os.path.join(output_directory, filename.replace(".py", ".rs")), "w") as rust_file:
rust_file.write(response)
This script uses DeepSeek to convert Python files to Rust and stores them in a new folder. With this configuration, project migration is hands-free.
Limitations and Considerations
DeepSeek is strong yet imperfect. AI-generated code needs human inspection for optimization and efficiency. Complex language-specific features may need manual modifications. In languages like Rust, performance optimization needs memory management techniques that AI may not optimize.
Conclusion
Code migration no longer needs to be difficult and time-consuming. AI-powered solutions like DeepSeek help engineers optimize code instead of rewriting it. DeepSeek speeds up, simplifies, and reduces errors when switching languages like Python to Rust, JavaScript to TypeScript, and others.
Now is the moment to try DeepSeek and change how you move code. AI-powered development is now.
113 views