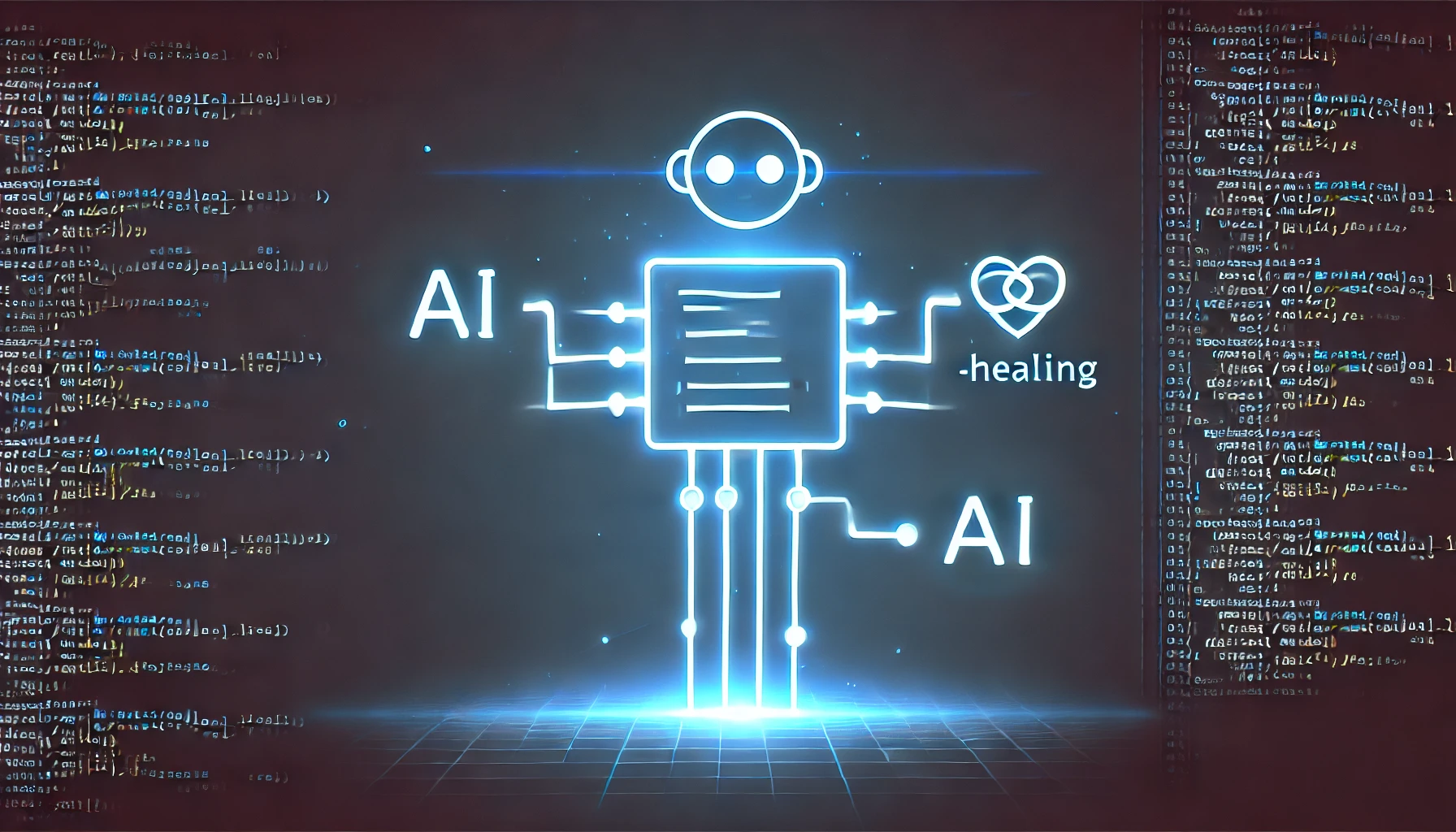
March 31, 2025
Building a Self-Healing Codebase with DeepSeek AI
Debugging takes time and frustration. I spent hours searching for issues only to find a simple error that ruined everything. What if an AI handled this? DeepSeek AI finds defects and proposes required fixes, helping us build a self-healing codebase. Imagine developing code with AI detecting logical mistakes, syntax difficulties, and edge cases to improve maintainability. Let's find out how DeepSeek helps improve code debugging and optimization.
Understanding DeepSeek AI for Code Debugging
Advanced code analysis and improvement tool DeepSeek AI. It finds problems, improves structure, and proposes speed improvements. DeepSeek AI finds trends and logic, which makes it a useful tool for developers. Reading code by hand is a traditional way to fix. AI takes care of fixing bugs, so I can focus on adding features instead of fixing bugs for hours on end.
Automating Bug Fixes with DeepSeek
DeepSeek can fix common bugs on its own, which is one of its best features. Like, I wrote a simple method once to divide two numbers:
def divide(a, b):
return a / b
It looked fine until I tried to divide by zero. Suddenly, my program crashed because of a mistake. I used DeepSeek AI to make a fix instead of fixing it manually:
buggy_code = """
def divide(a, b):
return a / b
"""
prompt = f"Fix any potential bugs in this code: {buggy_code}"
response = DeepSeekCode.generate(prompt)
print(response)
DeepSeek returned a much safer version:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
The AI detected the bug right away and fixed it, which saved me time and stopped future crashes.
Handling Logical Errors and Edge Cases
DeepSeek does not just fix grammar mistakes; it also finds mistakes in logic that could be hard to detect. I wrote a function once that would find square roots because I thought it would always get valid input:
import math
def sqrt(x):
return math.sqrt(x)
What is wrong? It cannot deal with negative numbers, which causes it to crash. When I executed this code through DeepSeek, I got a better version:
import math
def sqrt(x):
if x < 0:
raise ValueError("Cannot calculate square root of a negative number")
return math.sqrt(x)
By using AI to make this easy fix, the function became more stable and less likely to crash.
Refactoring for Maintainability
Fixing bugs is only one part of DeepSeek's job. It also improves and refactors code to make it easier to read and work better. I made a function that could handle a list of numbers once, but it was big and did not work well:
def process_data(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
return result
DeepSeek suggested a cleaner, more Pythonic approach:
def process_data(data):
return [item * 2 for item in data if item % 2 == 0]
Because the AI changed a long function with a lot of loops into a clean one-liner, my code works better and is easier to read.
Limitations and Challenges
DeepSeek is strong, but it is not perfect. It is still necessary for humans to check over fixes made by AI, especially in complicated situations where context is important. The AI might sometimes suggest wrong reasoning, and depending on it without question could lead to small bugs. That is why I added DeepSeek's ideas to my CI/CD pipeline. This way, I can be sure that every AI-assisted fix is tried and reviewed before it is released.
Conclusion
Debugging with AI is the way of the future. I can code more and spend less time looking for bugs now that I have DeepSeek AI. It solves bugs automatically, improves organization, and even finds logical errors, which helps me create a program that can fix itself. AI is a game-changer for software development, but it cannot replace human insight. To let AI do the hard work while you write great code now is the time to try it if you have not already.
30 views