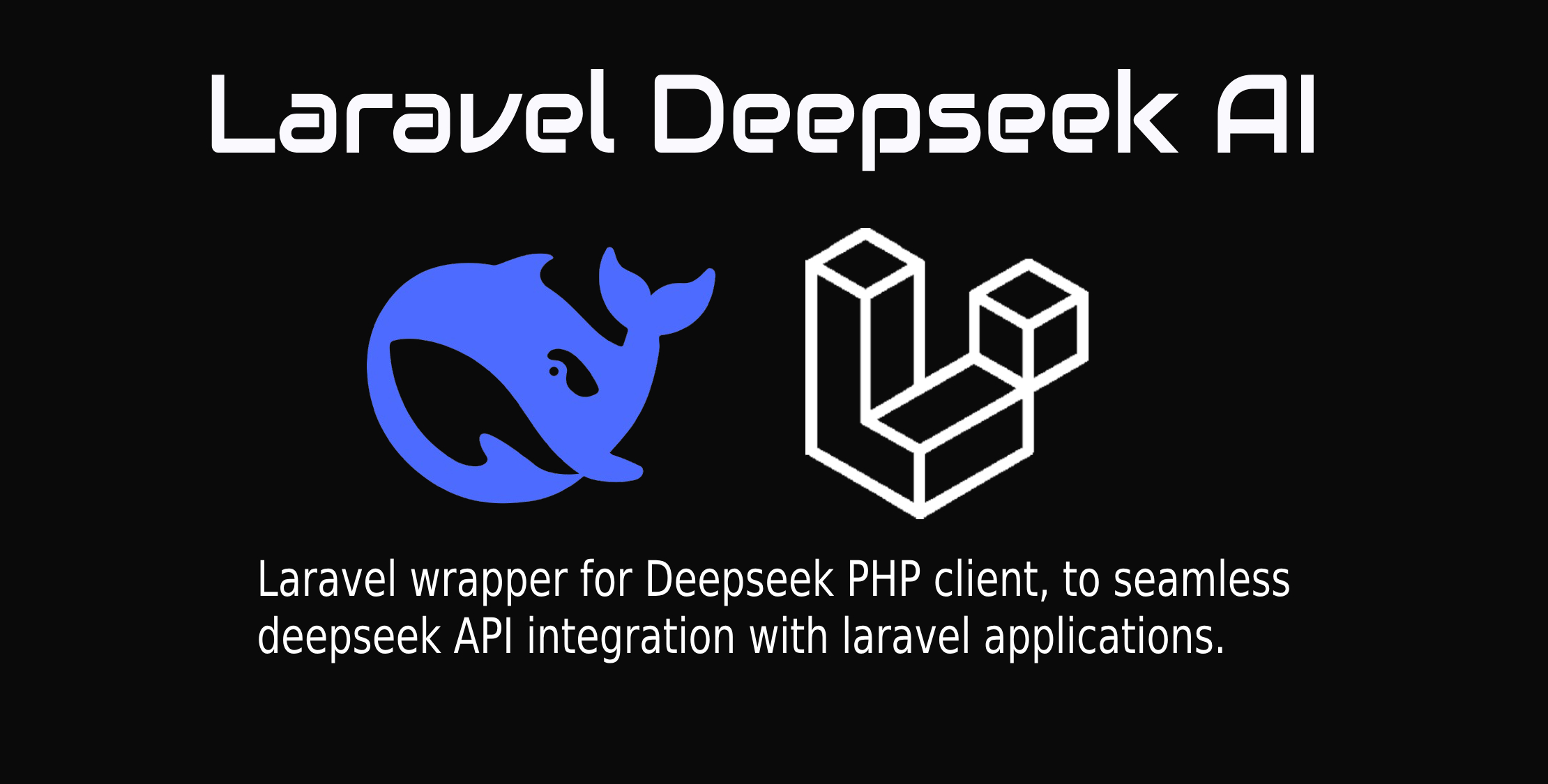
March 13, 2025
Building AI-Enhanced Laravel Apps with Deepseek Laravel
Ever considered adding an AI-powered assistant to your Laravel project? Deepseek Laravel makes it simple to employ powerful artificial intelligence to assess natural language searches, generate replies, and change interactions for certain use cases.
This package was easy to set up when I tried it. Deepseek Laravel makes installing an AI easy, whether you require complete customization or a quick start. This article will cover installation, basic use, local running, and response tweaking. You will be ready to use AI in Laravel apps by the end.
Installation
Getting Deepseek Laravel requires little effort. Use Composer to install the package:
composer require deepseek-php/deepseek-laravel
Next, publish the configuration file so you can tweak settings if needed:
php artisan vendor:publish --tag=deepseek
You can change default parameters in this configuration file. Add your API key to the .env file before running Deepseek:
DEEPSEEK_API_KEY="your_api_key"
And that's it! Deepseek Laravel is now installed and ready to go.
Basic Usage
Deepseek Laravel lets you quickly submit queries and get responses created by artificial intelligence. Here's a basic usage example:
use DeepSeekClient;
$deepseek = app(DeepSeekClient::class);
$response = $deepseek->query('Hello Deepseek, I am Laravel Framework, how are you Today ^_^ ?')->run();
print_r("Deepseek API response: " . $response);
This queries Deepseek and gets an answer. Deepseek automatically optimizes settings in simple mode, making AI integrations simple.
For default settings, see the published configuration file.
Advanced Usage
Customizing Deepseek searches using roles, models, and parameters gives you greater control. Here is an example of a more advanced way to do it:
use DeepSeekClient;
$deepseek = app(DeepSeekClient::class);
$response = $deepseek
->query('Hello Deepseek, how are you?', 'system')
->query('Hello Deepseek, my name is PHP', 'user')
->withModel("deepseek-chat")
->setTemperature(1.5)
->run();
print_r("Deepseek API response: " . $response);
Use user roles to build a multi-turn conversation. WithModel("deepseek-chat") creates a model, while SetTemperature(1.5) alters response randomness. Higher temperatures foster creativity, whereas lower ones preserve predictability.
Developers designing chatbots, content generators, or AI-driven Laravel apps that need dynamic responses would love this degree of control.
Running Deepseek Locally
Follow these instructions to test Deepseek Laravel locally.
Check your Laravel setup first. Start your Laravel Sail development container:
./vendor/bin/sail up -d
If you're not using Sail, just run your Laravel server as usual:
php artisan serve
Now, make sure your .env file includes your Deepseek API key:
DEEPSEEK_API_KEY="your_api_key"
To test everything, open Laravel Tinker:
php artisan tinker
Then, run a quick query:
use DeepSeekClient;
$deepseek = app(DeepSeekClient::class);
$response = $deepseek->query('Testing Deepseek locally!')->run();
print_r("Deepseek API response: " . $response);
If you see a response from Deepseek, congratulations! Your local setup is working perfectly.
Fine-Tuning Deepseek Laravel
Deepseek Laravel is strong by default, but customizing it may improve AI integration. Here are performance-boosting tips.
Choosing the Right AI Model
Deepseek utilizes a conventional model by default, but you may select a more complicated one:
$response = $deepseek->withModel("deepseek-pro")->run();
Controlling Response Creativity
AI responses are random or inventive, depending on temperature. Higher numbers add diversity, whereas lower values keep replies factual:
$response = $deepseek->setTemperature(0.8)->run();
When using Deepseek for chatbots or content development, this might help you be more creative.
Providing System Instructions
System commands may help Deepseek respond more systematically:
$response = $deepseek
->query('You are a helpful Laravel assistant.', 'system')
->query('How do I optimize Laravel performance?', 'user')
->run();
This ensures the AI understands its role and generates responses accordingly.
Caching API Responses
To optimize performance and reduce API calls, cache frequently used responses:
use Illuminate\Support\Facades\Cache;
Cache::remember('deepseek_response', 3600, function () use ($deepseek) {
return $deepseek->query('What is Laravel?')->run();
});
This stores the response for an hour, decreasing Deepseek API queries.
Conclusion
AI integration in Laravel projects is simple with Deepseek Laravel. This package gives versatility for basic query processing or comprehensive customisation with advanced parameters.
I can state from experience that Deepseek is a game-changer for developers who wish to use AI without complicated settings. After following this guide, you can:
- Install and setup Deepseek Laravel
- Send simple and complex AI inquiries.
- Run it locally for testing
- Optimize performance with fine adjustments.
Try Deepseek to improve your Laravel projects.
91 views