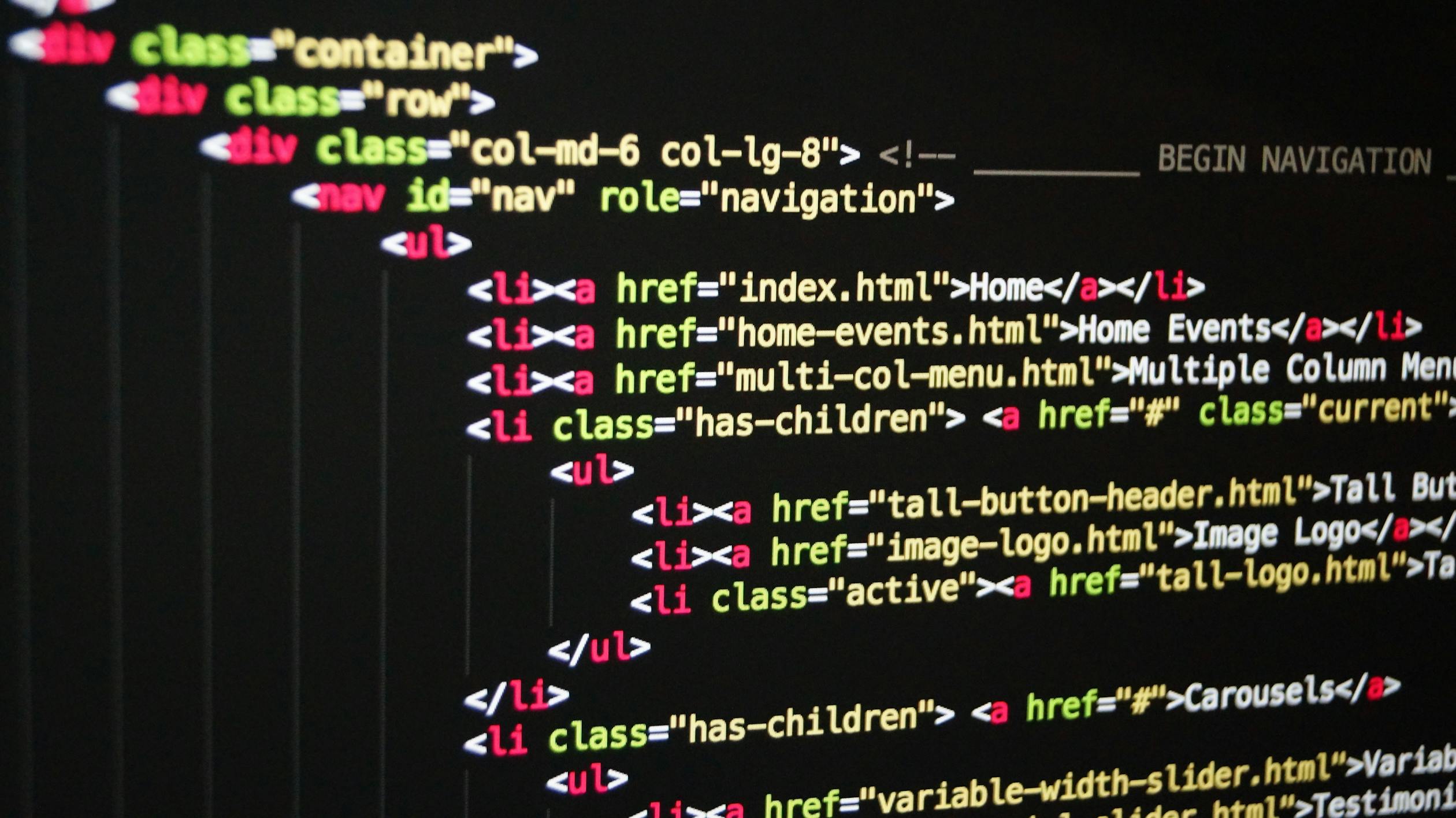
Feature tests in Laravel allow you to test your application's functionality from an end-user's perspective. Unlike unit tests that focus on testing individual units of code, feature tests mimic real user interactions and cover multiple parts of your application. This ensures that all components work seamlessly together and that your application behaves as expected. In Laravel 10, testing has been further improved to provide more efficient and robust ways to ensure the quality of your codebase.
In this tutorial, we'll cover the following topics:
- Setting up the Testing Environment
- Creating Feature Tests
- Writing Test Cases
- Running Tests
- Assertions and Assertions Helpers
Setting up the Testing Environment
Before you start writing feature tests, ensure that you have a Laravel 10 project set up. You can create a new project using Composer:
composer create-project laravel/laravel my-project
Once your project is ready, navigate to the project directory and configure the testing environment by modifying the .env.testing file to use a separate testing database. Make sure to run migrations and seeders to set up the testing database:
php artisan migrate --env=testing
php artisan db:seed --env=testing
Creating Feature Tests
Laravel 10 provides a convenient Artisan command to generate feature tests:
php artisan make:test Feature/MyFeatureTest
This command will create a new feature test file in the tests/Feature directory.
Writing Test Cases
Now, let's dive into writing actual test cases. In your MyFeatureTest.php file, you can define test methods using the test prefix. Here's an example of a basic test case that checks if the login page loads successfully:
use Illuminate\Foundation\Testing\RefreshDatabase;
use Tests\TestCase;
class MyFeatureTest extends TestCase
{
use RefreshDatabase;
public function testLoginPageLoads()
{
$response = $this->get('/login');
$response->assertStatus(200);
}
}
Running Tests
You can run your feature tests using the following command:
php artisan test
This command will discover and run all the tests in your application.
Assertions and Assertion Helpers
Laravel provides a wide range of assertion methods to simplify writing test cases. In the above example, we used the assertStatus method to check the HTTP response status. You can use other assertion methods like assertSee, assertDontSee, assertRedirect, and more.
Explore the official Laravel documentation for a comprehensive list of available assertion methods: Laravel Testing Documentation.
Conclusion
In this tutorial, we've introduced you to writing feature tests in Laravel 10. These tests allow you to simulate user interactions and ensure your application functions as expected. By following the steps outlined here, you can create robust and reliable tests for your Laravel application, helping you deliver high-quality software to your users.
363 views