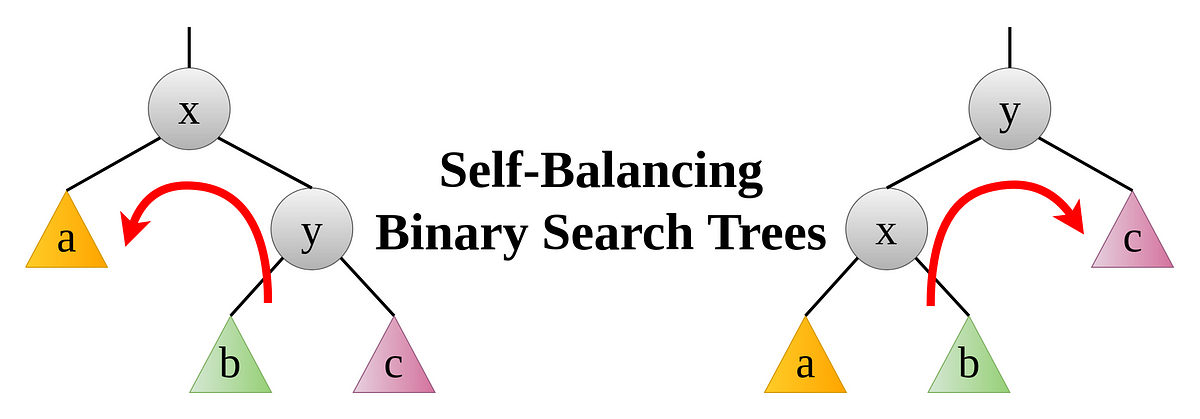
May 19, 2025
How to Implement a Self-Balancing Binary Search Tree in C++
If you have worked with enormous datasets, you know how crucial efficient search, insert, and remove is. A frequent option is the Binary Search Tree (BST), however it has a catch. Unbalanced trees can reduce operations from O(log n) to O(n), making them inefficient. Here come self-balancing Binary Search Trees (BSTs). This tutorial will show you how to create a self-balancing BST in C++ using the AVL tree, a common self-balancing tree structure.
What is a Self-Balancing Binary Search Tree?
Nodes in a Binary Search Tree (BST) have at most two children, with the left child smaller than the parent and the right child bigger. This structure streamlines element search, insertion, and deletion. A standard BST may perform poorly if the tree is imbalanced, resulting in lengthy pathways and wasteful operations.
Self-balancing trees like AVL trees automatically modify their structure to reduce this issue. These trees maintain a logarithmic height relative to the amount of elements for best efficiency.
Why Use a Self-Balancing Tree?
A self-balancing BST's key benefit is performance. A well-balanced tree makes insertion, deletion, and searching O(log n) time. Due to sorted elements, an imbalanced BST can degrade to O(n), resembling a linked list.
A self-balancing tree is essential for databases, caching systems, and other applications that need rapid access to elements and massive volumes of data or frequent dynamic modifications.
The AVL Tree: A Self-Balancing BST
AVL trees are famous self-balancing trees. Adelson-Velsky and Landis invented the first self-balancing BST. A balancing factor, the height difference between a node's left and right subtrees, balances an AVL tree. A balance factor of 0 means the node is balanced, 1 means the left subtree is higher, and -1 means the right subtree is higher.
Rebalancing the tree using rotations is necessary to maintain O(log n) operations when any node's balance factor exceeds this range.
Implementing an AVL Tree in C++
Here's how to implement an AVL tree in C++. A typical AVL tree contains nodes with values, left and right children, and heights. A node's height is its longest route to a leaf.
Here's an easy way to structure the tree:
struct Node {
int value;
Node* left;
Node* right;
int height;
};
We require insertion, balance factor, and rotation functions to maintain a state of equilibrium Start with insert function:
Node* insert(Node* root, int value) {
if (root == nullptr)
return new Node{value, nullptr, nullptr, 1};
if (value < root->value)
root->left = insert(root->left, value);
else
root->right = insert(root->right, value);
root->height = 1 + max(getHeight(root->left), getHeight(root->right));
int balance = getBalanceFactor(root);
// If the tree becomes unbalanced, perform rotations
if (balance > 1 && value < root->left->value) // Left Left Case
return rotateRight(root);
if (balance < -1 && value > root->right->value) // Right Right Case
return rotateLeft(root);
if (balance > 1 && value > root->left->value) { // Left Right Case
root->left = rotateLeft(root->left);
return rotateRight(root);
}
if (balance < -1 && value < root->right->value) { // Right Left Case
root->right = rotateRight(root->right);
return rotateLeft(root);
}
return root;
}
We put the value into the tree, update the height, and check any balancing factor-based rotations in this insert function.
Balancing Operations in Detail
Rotations are key to AVL tree balancing. Four rotations correct imbalances:
- Right Rotation: Use right rotation when left subtree is too tall.
- Left Rotation: When the right subtree is excessively tall.
- Left-Right Rotation: A mixture of left and right rotations employed in the left-right scenario.
- Right-Left Rotation: A right-left rotation combination for the right-left situation.
For example, a right rotation works like this:
Node* rotateRight(Node* y) {
Node* x = y->left;
Node* T2 = x->right;
x->right = y;
y->left = T2;
y->height = max(getHeight(y->left), getHeight(y->right)) + 1;
x->height = max(getHeight(x->left), getHeight(x->right)) + 1;
return x;
}
Conclusion
A self-balancing binary search tree like the C++ AVL tree guarantees fast tree operations as the dataset grows. With AVL trees, you may securely insert, delete, and search in logarithmic time. Understanding and executing rotations helps you balance and optimize your programs. There is more to learn about balanced trees, so experiment and apply the ideas to your projects.
199 views