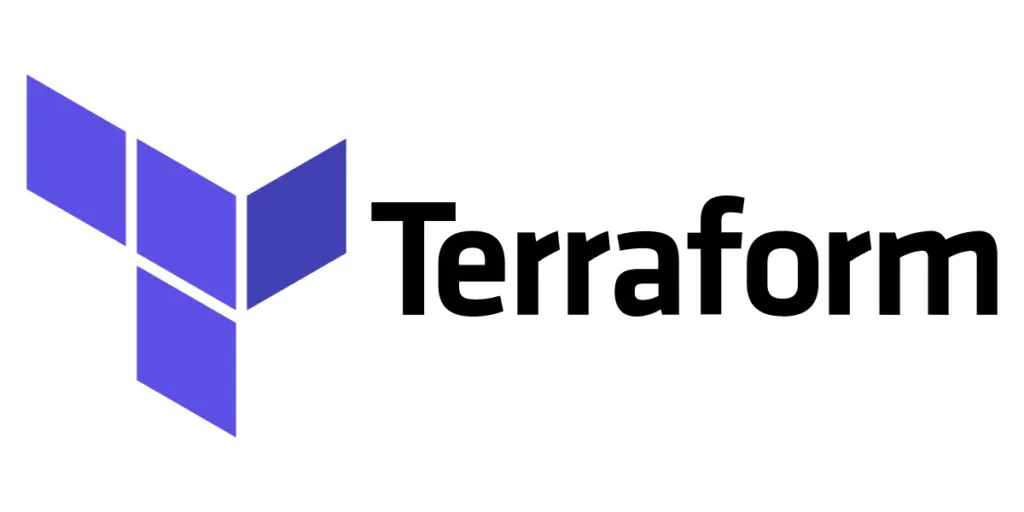
May 16, 2025
How to Use Go to Automate Infrastructure with Terraform Plugins
In cloud infrastructure, manual resource management is useless. Terraform can automate practically everything. What if Terraform suppliers do not support your use case? Custom plugins are a game changer for developers who desire greater infrastructure automation control. The appropriate language for strong, scalable Terraform plugins is Go. This post will teach you how to utilize Go to construct custom Terraform plugins and improve infrastructure automation.
What Are Terraform Plugins?
Understanding Terraform plugins is crucial before learning Go. Terraform supports Infrastructure as Code (IaC) resource definition and provisioning. Through 'providers', it communicates with the APIs of the services you manage. Terraform has pre-built providers for AWS, Azure, and Google Cloud, but sometimes you need something special.
Plugins help there. Terraform plugins provide functionality, such as accessing an API without a provider. Best part? Write these plugins in Go, the main language for doing so.
Why Go for Terraform Plugins?
Wondering why Go? There are various causes. Go is fast and efficient, perfect for performance-critical applications. Second, Go is suited for concurrent cloud service interactions due to concurrency. Finally, Go's simplicity and readability aid when designing a key infrastructure plugin.
Go works well with Terraform's plugin architecture, making it ideal for scaling Terraform's capabilities. A powerful programming language that works well with Terraform's IaC infrastructure.
Setting Up Your Development Environment
Start by setting up your development environment. Install Terraform and Go first. Go is available from the official site, and Terraform from HashiCorp.
Next, set up a Go-based Terraform plugin working directory. Name it terraform-provider-custom. To set up your Go module in this folder, run:
go mod init terraform-provider-custom
Install the required dependencies. The most essential is terraform-plugin-sdk, which offers Terraform provider building tools. Run to install:
go get github.com/hashicorp/terraform-plugin-sdk/v2
After setting up your environment, now its time to write your plugin.
Writing a Basic Terraform Plugin with Go
A Go Terraform service has this layout:
- Provider: Terraform's plugin entry point for initialization.
- Resources: Your plugin maintains infrastructure resources.
- Data sources: Provides plugins with resource information.
Simple Go custom service that interfaces with a fictional API to handle "foo" resources.
package main
import (
"github.com/hashicorp/terraform-plugin-sdk/v2/helper/schema"
)
func main() {
// Define the provider and resources
}
func provider() *schema.Provider {
return &schema.Provider{
ResourcesMap: map[string]*schema.Resource{
"custom_foo": resourceFoo(),
},
}
}
func resourceFoo() *schema.Resource {
return &schema.Resource{
Create: resourceFooCreate,
Read: resourceFooRead,
Delete: resourceFooDelete,
// Additional CRUD functions go here
}
}
This basic provider can create, read, and delete "foo" resources. Replace CRUD operations with API-interactive logic. Terraform-plugin-sdk abstracts plugin development complexity, letting you concentrate on business logic.
Testing Your Plugin Locally
You should test your plugin locally after writing it. From the command line, perform Terraform commands. Enter your plugin directory and run:
terraform init
terraform apply
Terraform initializes your plugin and applies configuration file modifications. You may check your provider's behavior here. Terraform gives precise error reports to help you troubleshoot.
Best Practices for Terraform Plugins in Go
Build maintainable custom Terraform plugins, not simply functional ones. Clean and modular code is essential when your provider interacts with several services or gets more complex. To simplify debugging, use comprehensive error handling and logging.
Another ideal practice is version control. Tag versions and follow semver as your plugin improves to prevent breaking changes for users.
Conclusion
Building custom Terraform plugins in Go is a great approach to customize Terraform for your infrastructure. Go's simplicity and efficiency make it ideal for plugin development, whether you are managing unique services or automating complicated procedures. By following these steps, you will learn how to create strong, reusable Terraform tools that will automate your infrastructure more effectively than ever.
239 views