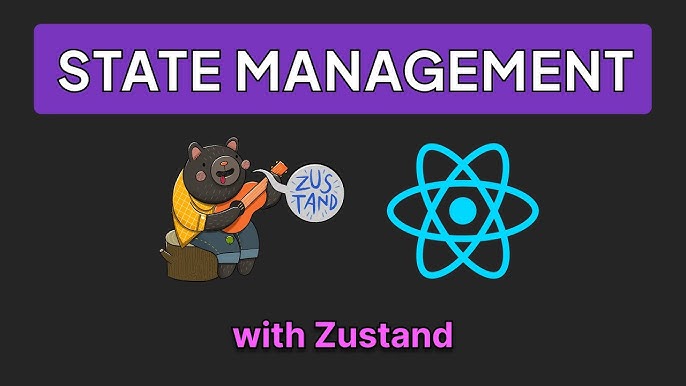
May 15, 2025
State Management Simplified: Using Zustand for Scalable React Apps
State management may become difficult as React apps scale. Prop-drilling, complex state management, and performance difficulties may be overwhelming. Zustand is a simple yet robust state management library that simplifies life. Zustand's reactive nature and small size make state management and scaling React projects easier than Redux. Zustand will simplify state management in React projects, this article will show you how to integrate it.
Why Zustand?
Zustand is a lighter alternative to Redux for developers. Zustand has a simple API and no reducers or actions, unlike Redux, which involves a lot of boilerplate code and setup. To simplify component sharing, it provides a single repository for your state. Zustand is ideal for smaller projects or those that wish to bypass the overhead and code quicker due to its simplicity.
Zustand is more user-friendly, reactive, and scalable than competing state management frameworks. If you are familiar with React's local state but need something more robust, Zustand makes app-wide state management easy.
Setting Up Zustand in Your React Project
Zustand starts quickly and easily. Simply install the library using npm or yarn:
npm install zustand
Install and start building your store. Zustand stores are simple JavaScript objects that retain your application's state. Zustand's build function lets you establish state variables and update functions for a store.
Simple store for counter management:
import create from 'zustand';
const useStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
This store has a count state and two actions (increment and decrement) to change it. State management is complete with a few lines of code.
Core Concepts of Zustand
After setting up the store, let's explore Zustand's capabilities and how it works.
Zustand's create function accepts an object to build a store. The object includes initial state and modification methods. Returning a new state object from the set function in each method updates the state.
Component state subscription is one of Zustand's most notable features. Any component that uses the useStore hook immediately subscribes to store changes. Because components always render with the latest state, state management is significantly easier than tough prop-drilling.
Zustand provides built-in middleware for page reload state persistence. The persist middleware lets you save the store's state to local storage so your app remembers it after refreshing the page.
Here's one way to implement persistence:
import create from 'zustand';
import { persist } from 'zustand/middleware';
const useStore = create(
persist(
(set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}),
{ name: 'counter-storage' }
)
);
Zustand syncs the store with local storage and maintains state across sessions using this arrangement.
Using Zustand in React Components
Zustand is easy to use in React components after creating your store. Call the useStore hook to access state and execute actions. Zustand's reactive nature means that only components utilizing the state will re-render when the state changes, which is the best part.
Let's see how to integrate the store into a counter component:
import React from 'react';
import { useStore } from './store';
const Counter = () => {
const { count, increment, decrement } = useStore();
return (
<div>
<h1>{count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
The component subscribes to the count state and refreshes the display to reflect changes. Clicking the buttons executes increment and decrement. Zustand is great because it manages reactivity without boilerplate code.
Advanced Features of Zustand
Zustand also supports middleware for logging, saving state, and connecting with debugging tools like Redux DevTools.
A devtools middleware can monitor app state changes, making debugging easier:
import create from 'zustand';
import { devtools } from 'zustand/middleware';
const useStore = create(
devtools((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
}))
);
For better debugging, the devtools middleware lets you view your state in the browser's developer tools, monitor state changes, and undo/redo operations.
Best Practices for Using Zustand
Zustand's API is basic, yet there are recommended practices. Small to medium-sized applications should concentrate their stores on their key states. Zustand is unsuitable for maintaining local, component-level state. React's useState is superior.
Zustand with the Context API can manage complex state or transfer state down the component tree as your project evolves.
Conclusion
Now, you've come to know that Zustand is a light and scalable state management solution for React projects. Its simple API, responsiveness, and scalability make it ideal for small and medium-sized projects. Zustand lets you create your app instead of maintaining its state by removing boilerplate code and delivering strong capabilities like middleware. Try Zustand in your next project to see that state management does not have to be hard.
41 views