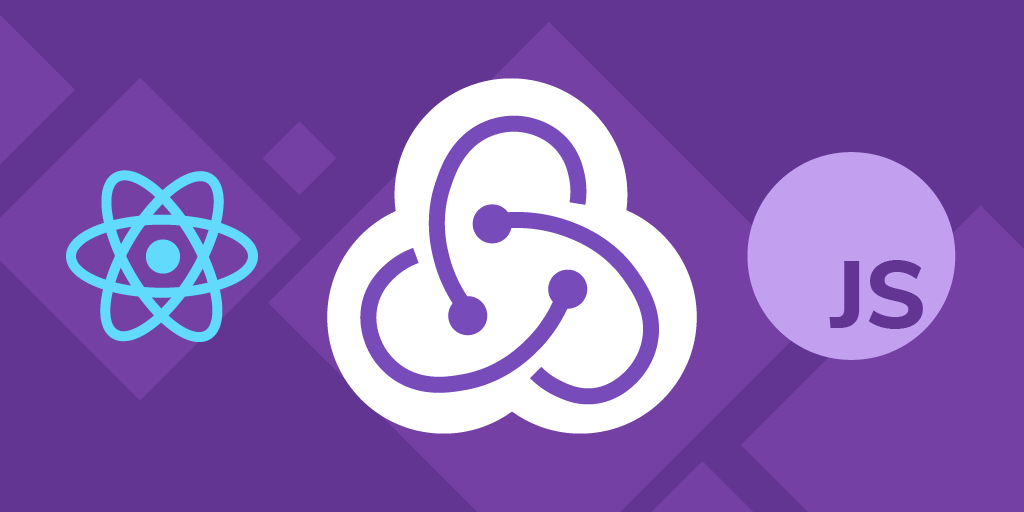
May 14, 2025
Mastering State Management: How to Use Redux with Modern JavaScript
I have worked on advanced JavaScript apps, and managing state can be difficult, particularly as the application scales. Maintaining consistency and tracking component status can be a hassle. Redux, one of the most powerful state management solutions, can rescue the day. Centralizing the state facilitates app management and debugging. I will explain how to install Redux in your JavaScript project, cover fundamental ideas, and integrate it with modern frameworks like React in this article. Jump in!
What is Redux?
Redux is a state management library that lets you predictably manage application state. It is built on three main principles:
- 1. Single source of truth: Application state is maintained in one place, simplifying management and debugging.
- 2. Read-only state: Only actions can change it.
- 3. Changes done using pure functions: Simple, side-effect-free actions and reducers.
Large apps with shared state benefit from Redux. Redux may be excessive for a minimal project, and local component state may be easier.
Setting Up Redux in a JavaScript Project
Before using Redux, we must install libraries. React users must install redux and react-redux first. This is possible using npm:
npm install redux react-redux
Building your Redux setup begins after installing the libraries. A typical Redux program has three parts:
- Actions: These are simple objects that describes app events.
- Reducers: Functions that manage state changes due to actions.
- Store: It saves state and allows for actions to be taken.
Understanding Redux Concepts
Actions:
First of all you need to know Redux depends on actions. A simple JavaScript action can explain application events. Users can increase a counter by clicking a button.
const increment = { type: 'INCREMENT' };
Reducers:
Here come reducers. Reducers define how actions modify the application state. A simple counter reducer:
const counter = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
};
Store:
The store is the main place where your application's information is kept. Redux's createStore method takes a reducer to create a store:
import { createStore } from 'redux';
const store = createStore(counter);
The store saves state and lets you dispatch actions that activate reducers to change it.
Using Redux with Modern JavaScript
Now that you know the basics, let's combine Redux with React (or any current JavaScript framework). React requires wrapping your root component in a react-redux Provider component to allow all components store access:
import { Provider } from 'react-redux';
import { store } from './store';
function App() {
return (
<Provider store={store}>
<Counter />
</Provider>
);
}
UseSelector and useDispatch hooks let components interface with Redux stores. UseDispatch lets you alter the state, whereas useSelector lets you read it. This Counter component uses both hooks:
import { useSelector, useDispatch } from 'react-redux';
function Counter() {
const count = useSelector((state) => state);
const dispatch = useDispatch();
return (
<div>
<h1>{count}</h1>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Increment</button>
</div>
);
}
UseSelector reads the current state and useDispatch clicks to increment the counter.
Redux Toolkit optimizes numerous Redux functionalities and minimizes boilerplate for a nicer experience. It offers createSlice, which integrates actions and reducers:
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: (state) => state + 1,
decrement: (state) => state - 1,
},
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
Best Practices for Using Redux
Redux actions must be concise and explicit. Redux manages the basic state of your application, so avoid storing derived data. Redux Toolkit reduces boilerplate and improves maintainability.
Separating issues helps organize your app. Place actions, reducers, and components in appropriate folders and separate reducers by feature to minimize oversizing. Use memoization tools like reselect to generate selectors that compute derived data without re-rendering to maximize speed.
Common Pitfalls and How to Avoid Them
Redux is a strong tool, but it can be misused easily. Overusing Redux for basic state management, such component local state, is a typical mistake. React's useState works better in these circumstances.
Massive, unmanageable reducers are another concern. Split the large reducer into smaller, more specialized reducers for individual state sections. Unsubscribe from listeners to minimize memory leaks, particularly when utilizing middleware or external APIs.
Conclusion
Redux Toolkit makes it simpler than ever to start using Redux for complex application state management. Redux lets you construct scalable apps with data control by centralizing state predictably. As you learn Redux, explore middleware (Redux Thunk) and async actions.
204 views