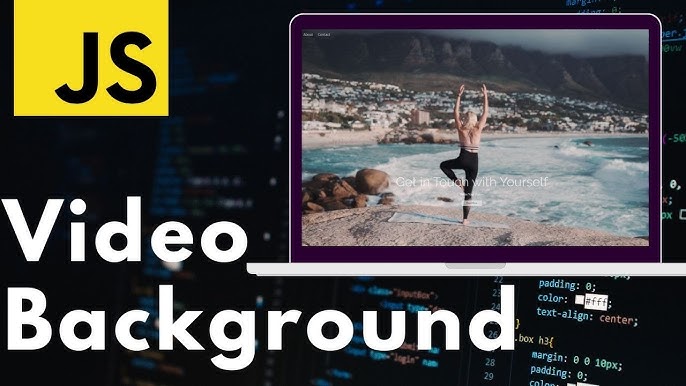
May 05, 2025
How to Add a Full-Screen Video Background with JavaScript
Web designs no longer uses static images. Websites must engage their visitors. Videos are very popular backgrounds. Imagine loading your website with a beautiful video background that enhances user experience. This tutorial will teach you how to use JavaScript, CSS, and HTML to add a full-screen video background to your website.
What You'll Need
Let's discuss your needs before diving into the coding. We will utilize three methods to create a full-screen video background:
- HTML5 for basic structure.
- CSS for video style and screen filling.
- JavaScript for enhanced functionality and responsiveness.
Use a high-quality web-optimized video for background. Compress file size to speed your site.
Setting Up the HTML Structure
First, let's setup our video background in HTML. We will use the HTML5 tag for this:
<video id="bg-video" autoplay loop muted playsinline>
<source src="your-video.mp4" type="video/mp4">
<source src="your-video.webm" type="video/webm">
Your browser does not support the video tag.
</video>
<div class="content">
<h1>Welcome to My Website</h1>
<p>This is a simple demo of a full-screen video background using HTML, CSS, and JavaScript.</p>
</div>
Autoplay starts the video when the website loaded, and loop restarts it when it stops. Autoplay requires muted, particularly on mobile devices. playsinline guarantees mobile video plays inline instead than fullscreen.
Styling the Video Background with CSS
After setting up the structure, let's make the video fill the screen. This is where CSS helps. The video should fill the entire screen without distorting its aspect ratio. This CSS does that:
#bg-video {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
object-fit: cover;
z-index: -1; /* Make sure the video stays behind content */
}
.content {
position: relative;
z-index: 1;
text-align: center;
color: white;
}
The object-fit: cover keeps the video's aspect ratio and fits the screen without stretching or distorting. Z-index positions the video beneath text and buttons on the page.
Adding JavaScript for Smooth Functionality
Simple HTML and CSS setting works, but adding JavaScript improves functionality and screen scaling. Video resizing for the browser window is key. This little JavaScript code handles resizing:
window.addEventListener('resize', function() {
var video = document.getElementById('bg-video');
var windowHeight = window.innerHeight;
var windowWidth = window.innerWidth;
video.style.height = windowHeight + 'px';
video.style.width = windowWidth + 'px';
});
This code changes video dimensions as the window resizes. It guarantees that the video covers the whole screen regardless of window size.
Add interactivity like a play/pause button or video loading detection. JavaScript may make your video background more dynamic and user-friendly.
Conclusion
A full-screen video background may quickly improve your website's look and usability. Few lines of HTML, CSS, and JavaScript may make your visitors' experience entertaining and appealing. To guarantee a pleasant experience, optimize your video and test its layout across devices.
253 views