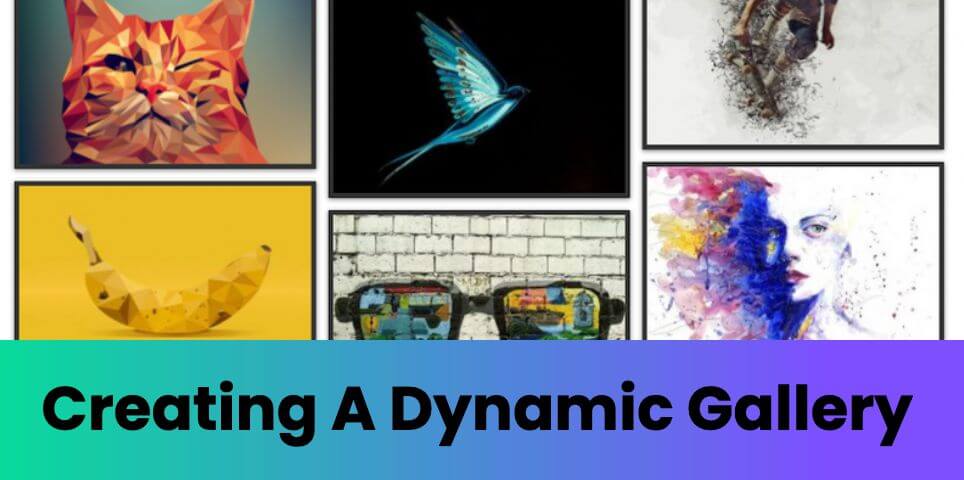
April 25, 2025
Creating Dynamic Photo Galleries Using XML
Have you ever wanted to develop an excellent photo gallery for your website but felt intimidated by coding? Lucky you! Building a dynamic photo gallery using XML is today's tutorial. It is easier than you think, and the benefits are worth it! Photo data storage and management using XML is a secret. Let's make your static galleries active wonders.
Why Use XML for Photo Galleries?
Ask yourself, Why XML? Let me clarify. XML is the ideal data organizer. It is lightweight, human-readable, and compatible with JavaScript and PHP. Best part? XML separates stuff and display. This lets you manage photographs in one file and style them elsewhere. Like having the best gallery project organizing tool!
Setting Up Your Environment
Prepare your tools before we begin. You will not need anything lavish. You just need:
- A text editor (e.g., VS Code or Notepad++
- A web browser (any current one would suffice).
- A local server is optional but useful for testing.
Start with a simple HTML layout. This is your gallery's framework. Start with this simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dynamic Photo Gallery</title>
</head>
<body>
<div id="photo-gallery"></div>
</body>
</html>
Creating the XML File
Making your XML file is now the fun part. You can think of this file as the center of your photo gallery. It is where you will keep all the details about your photos, like names, file paths, and comments.
<gallery>
<photo title="Sunset" src="sunset.jpg" description="A beautiful sunset." />
<photo title="Mountain" src="mountain.jpg" description="A majestic mountain." />
<photo title="Beach" src="beach.jpg" description="Relaxing waves and golden sand." />
</gallery>
That is pretty cool, right? It is easy to add or change photos with this layout because you do not have to touch the HTML. Save this as gallery.xml.
Fetching XML Data Using JavaScript
Let's make your photos come to life now. It only takes a few lines of JavaScript to get data from an XML file and show it on the screen.
Here's how you can do it using the Fetch API:
fetch('gallery.xml')
.then(response => response.text())
.then(xmlText => {
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlText, "application/xml");
const photos = xmlDoc.getElementsByTagName("photo");
let galleryHTML = '';
for (let photo of photos) {
const title = photo.getAttribute("title");
const src = photo.getAttribute("src");
const description = photo.getAttribute("description");
galleryHTML += `
<div class="photo">
<img src="${src}" alt="${title}">
<h3>${title}</h3>
<p>${description}</p>
</div>`;
}
document.getElementById("photo-gallery").innerHTML = galleryHTML;
});
Your gallery really comes to life with this script. Your XML file holds all the information, so changing things in your gallery is as simple as changing words in a file.
Styling and Enhancing the Gallery
Not only does a gallery need to work, but it also needs to look good! You can edit your gallery and make it look good by using CSS. Here's a simple CSS snippet to get you started:
#photo-gallery {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 20px;
}
.photo img {
width: 100%;
border-radius: 10px;
}
.photo h3 {
margin: 10px 0 5px;
}
Adding JavaScript tools like Lightbox to your gallery can also make it better by letting you add dynamic features like scrolling or rotating views.
Testing and Debugging Tips
The fact is that things may not work exactly the first time, and that is fine!
- Make sure there are no grammar mistakes in your XML file. Not having a > can break everything.
- Use browser developer tools to address JavaScript issues.
- If cross-origin issues persist, use local servers like XAMPP or WAMP.
Conclusion
You did it! You have created a dynamic photo gallery with XML. Your gallery is not only adaptable, but it is also simple to keep up to date.
If you want to try something new, you could add groups to your XML file or add an API that changes your photos in real time. With XML, there are a lot of options. Go ahead and show off your new photo gallery skills to everyone!
27 views