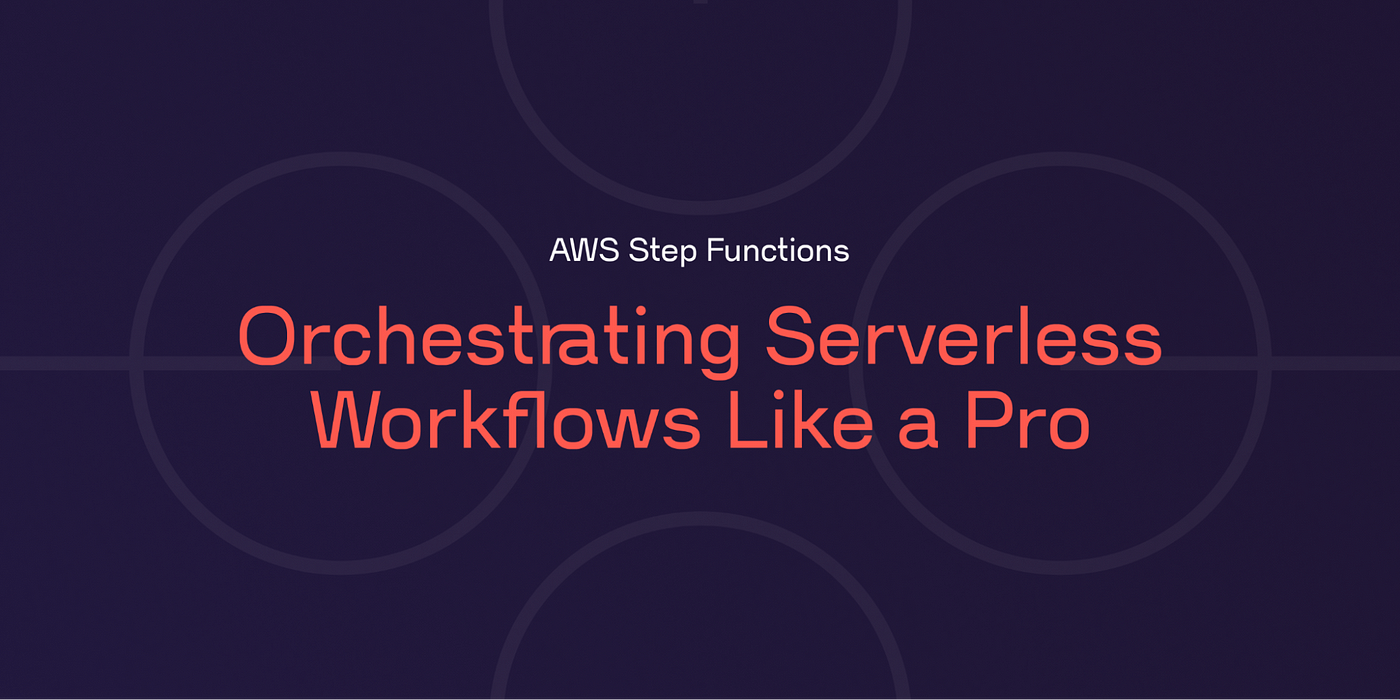
April 02, 2025
Orchestrating Serverless Workflows with AWS Step Functions: A Hands-on Tutorial with AWS CDK
Ever struggled to manage complex workflows across several serverless services? As cloud-native apps get more complex, task orchestration becomes crucial. AWS Step Functions is a robust and efficient tool for managing and automating workflows across AWS services. This article will teach you how to coordinate AWS Lambda functions and establish a scalable workflow using AWS Step Functions and AWS CDK to build a basic order processing system.
Understanding AWS Step Functions
Fully managed AWS Step Functions lets you integrate Lambda, DynamoDB, and S3 into a process. State machines describe tasks, transitions, and decision points, so you build workflows instead of controlling each task. Without service call overhead, complicated processes are simpler to implement.
Visual process design, AWS connection, automated retries, and extensive error handling are Step Functions' main features. It simplifies serverless architecture orchestration, letting you concentrate on application logic rather than infrastructure.
Why Use AWS Step Functions?
Managing many activities in a serverless environment may be difficult, particularly for long-running processes or systems that interface with several services. Easy process creation and automation using AWS Step Functions makes this simpler. Visual workflow design using Step Functions helps understanding and troubleshooting.
Built-in error handling and retry logic make Step Functions a reliable alternative for complex workflows in addition to visual workflow management. You do not need to provide infrastructure since it scales automatically depending on workload. You simply pay for what you use with most serverless systems, making it cost-effective for today's cloud apps.
Setting Up Your Environment
Prepare your work environment before starting the tutorial. First, install and setup AWS CLI and Node.js for AWS CDK.
Install AWS CDK globally on your system with this command:
npm install -g aws-cdk
Next, create a new CDK project with:
cdk init app --language=typescript
To deploy resources to your AWS account, setup your AWS CLI with proper credentials using aws configure.
Tutorial: Building an Order Processing Workflow
After setting up the infrastructure, let's develop a basic order processing system using AWS Step Functions and CDK.
1. Define the Lambda Functions
This example creates three Lambda functions:
- Validate Order: Verifies order data.
- Charge Payment: Handles order payments.
- Confirmation: Sends an email to the consumer.
Define these Lambda functions in CDK code:
import * as lambda from 'aws-cdk-lib/aws-lambda';
const validateOrder = new lambda.Function(this, 'ValidateOrder', {
runtime: lambda.Runtime.NODEJS_14_X,
handler: 'validateOrder.handler',
code: lambda.Code.fromAsset('lambda/validateOrder'),
});
const chargePayment = new lambda.Function(this, 'ChargePayment', {
runtime: lambda.Runtime.NODEJS_14_X,
handler: 'chargePayment.handler',
code: lambda.Code.fromAsset('lambda/chargePayment'),
});
const sendConfirmation = new lambda.Function(this, 'SendConfirmation', {
runtime: lambda.Runtime.NODEJS_14_X,
handler: 'sendConfirmation.handler',
code: lambda.Code.fromAsset('lambda/sendConfirmation'),
});
2. Create the Step Functions State Machine
Next, we will design a Step Functions state machine to organize Lambda functions. The state machine does each job sequentially, from order validation to payment processing to confirmation.
How to define the state machine using AWS CDK:
import * as stepfunctions from 'aws-cdk-lib/aws-stepfunctions';
import * as tasks from 'aws-cdk-lib/aws-stepfunctions-tasks';
const validateOrderTask = new tasks.LambdaInvoke(this, 'ValidateOrder', {
lambdaFunction: validateOrder,
outputPath: '$.Payload',
});
const chargePaymentTask = new tasks.LambdaInvoke(this, 'ChargePayment', {
lambdaFunction: chargePayment,
outputPath: '$.Payload',
});
const sendConfirmationTask = new tasks.LambdaInvoke(this, 'SendConfirmation', {
lambdaFunction: sendConfirmation,
outputPath: '$.Payload',
});
const definition = validateOrderTask
.next(chargePaymentTask)
.next(sendConfirmationTask);
const stateMachine = new stepfunctions.StateMachine(this, 'OrderProcessingStateMachine', {
definition,
});
3. Deploy and Test the Workflow
After creating Lambda functions and state machines, deploy them using AWS CDK. Deploy your stack using this command:
cdk deploy
Trigger the state machine via the AWS Console or SDK to test the workflow. When activated, the workflow validates, charges, and emails customers to confirm orders.
Error Handling and Best Practices
To ensure reliability, accept mistakes graciously while developing workflows. Catchers and retries in AWS Step Functions allow you to automatically retry failed processes.
You may add a failure status for order validation errors:
const validateOrderTask = new tasks.LambdaInvoke(this, 'ValidateOrder', {
lambdaFunction: validateOrder,
resultPath: '$.validateOrderResult',
catch: [new stepfunctions.Fail(this, 'OrderValidationFailed')],
});
Set timeouts and arrange the process to manage retries. Best practices will strengthen your process and reduce failure.
Conclusion
Managing complicated activities across various AWS services is simpler with AWS Step Functions' serverless workflow solution. With Step Functions, AWS Lambda, and AWS CDK, you can automate order processing easily. The service's visual workflow architecture, error management, and scalability keep your applications credible and cost-effective as they develop. This tutorial is your first step toward learning serverless orchestration. Now, explore more advanced capabilities to improve your workflows.
65 views