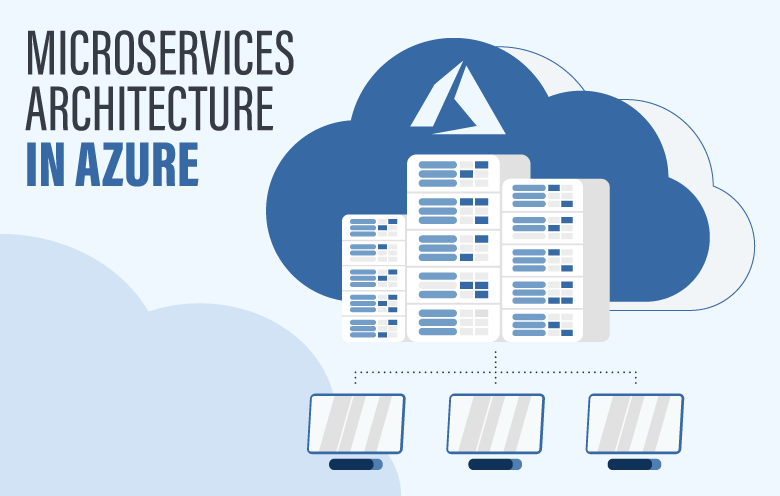
April 01, 2025
Designing Microservices with Azure Functions: A Step-by-Step Guide
How can new applications manage millions of users while being fast and reliable? The solution is microservices, a unique scalable and maintainable software strategy. Microservices provide flexibility by breaking applications into smaller, independent parts.
This tutorial uses Azure Functions and Event Grid to develop microservices. This will help you build loosely coupled microservices for a fictitious e-commerce site, whether you are new to serverless architecture or scaling your project.
Understanding Azure Functions and Azure Event Grid
A serverless computing service, Azure functions, runs short code functions without infrastructure. It excels in data processing, system integration, and event-driven applications. Functions scale automatically to manage different loads, are cost-effective since you only pay for the compute resources you use, and support C#, Python, and JavaScript.
Azure Event Grid is a controlled event routing solution that optimizes application communication. It routes events from Azure resources and bespoke apps to Azure Functions or Logic Apps for event-driven architectures. Event Grid delivers events efficiently with high throughput and low latency.
You can make flexible, decoupled microservices that respond quickly to changes in your application by mixing Azure Functions and Event Grid.
Use Case: E-Commerce Platform
Imagine running an e-commerce order processing system. As orders come in, the system must update inventory, confirm payments, and notify shippers. Traditional monolithic architectures are hard to expand and maintain. Microservices tackle these issues by splitting process into distinct components that interact via events.
This article will develop a microservice for order processing using Azure Functions for logic and Azure Event Grid for event routing.
Step-by-Step Tutorial
Setting Up the Environment
Start by installing Azure CLI and Visual Studio Code. Create an Azure account. The following CLI command creates a new Azure resource group:
az group create --name ECommerceResources --location eastus
Building the Order Processing Function
Start an Azure Function project in Visual Studio Code. Install Azure Functions in the editor. Create a new project, choose a runtime (e.g., JavaScript), and add a ProcessOrder method to handle order data. A sample function is shown below:
module.exports = async function (context, eventGridEvent) {
const order = eventGridEvent.data;
context.log(`Processing order ID: ${order.id}`);
// Simulate processing logic
context.log(`Order ${order.id} processed successfully.`);
};
Configuring Azure Event Grid
For order events, make a new Event Grid topic in the Azure portal. Note the topic's endpoint and key. This Azure CLI command subscribes the function to the Event Grid topic:
az eventgrid event-subscription create \
--name OrderEvents \
--source-resource-id /subscriptions/{subscription-id}/resourceGroups/ECommerceResources/providers/Microsoft.EventGrid/topics/OrderTopic \
--endpoint {function-endpoint}
Publish an event to the Event Grid to test the setup.
Deploying the Function
Visual Studio Code deploys the function to Azure. Make a Function App and add the ProcessOrder function to it. Publishing an order event and testing the function's execution tests the deployment.
Testing and Validation
Send an example Event Grid event to simulate order creation:
az eventgrid event publish \
--topic-endpoint https://{topic-name}.eventgrid.azure.net/api/events \
--sas-key {access-key} \
--event \
'{"id":"1","subject":"Order","eventType":"OrderCreated","data":{"id":"12345"},"eventTime":"2024-12-31T12:34:56Z","dataVersion":"1.0"}'
Verify that Azure Monitor logs show the function handled the event.
Best Practices for Microservices Design
Focus on one task to keep functions lightweight for success. Retry and observe failures to design for failure. Improve speed and scalability with Azure Monitor and auto-scaling.
Conclusion
Microservices created using Azure Functions and Event Grid are highly scalable and flexible. This tutorial taught you how to build an e-commerce order processing microservice. Try new use cases and read Azure's advanced feature documentation. These tools provide unlimited options!
254 views