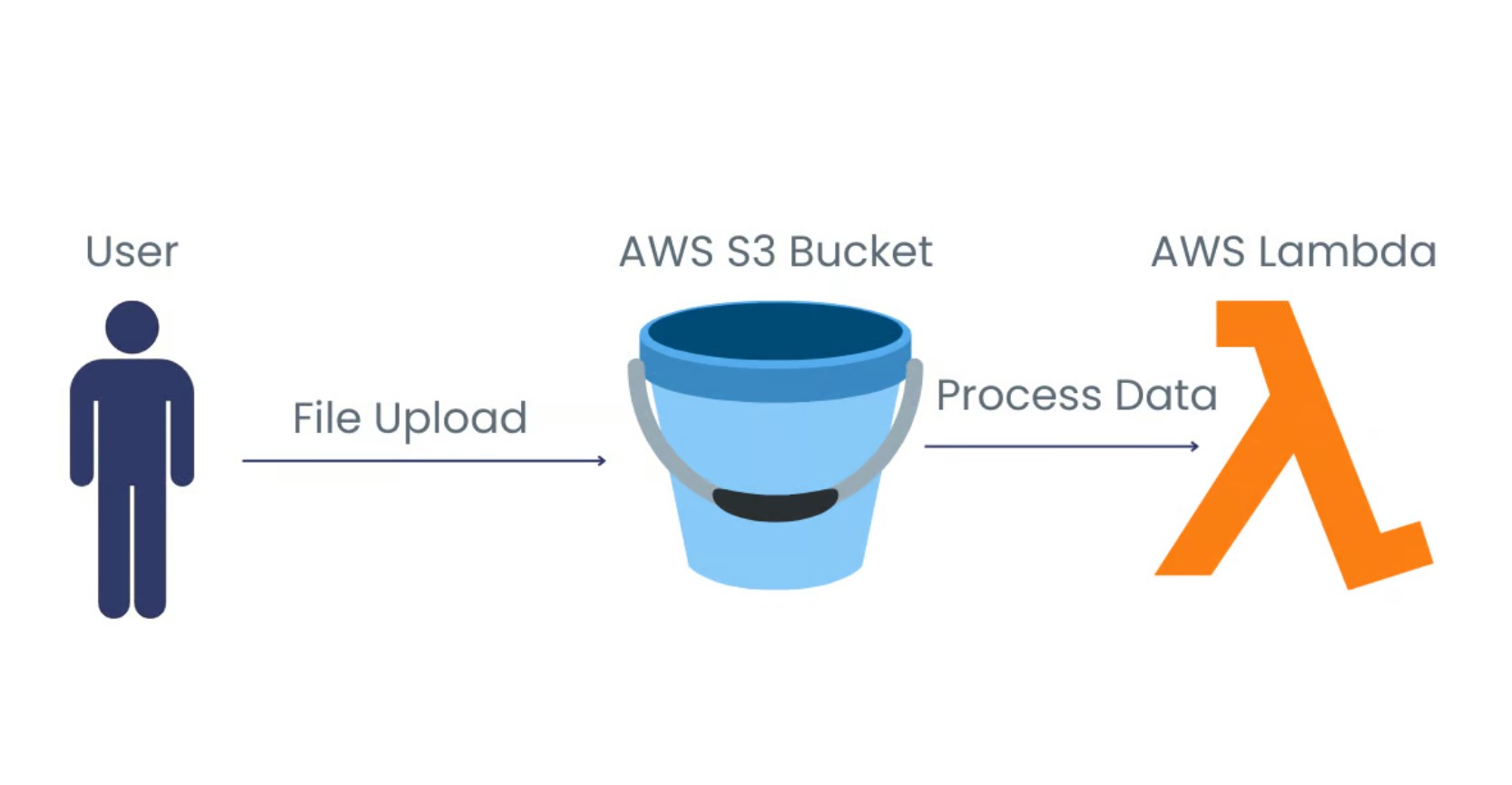
March 31, 2025
Mastering Event-Driven File Processing with AWS Lambda and S3
Have you tried automating file processing without server management? Event-driven architectures handle real-time events modernly and scalablely. AWS Lambda with S3 can create a serverless system that responds rapidly to file uploads. The uploading of a file might start a process that looks at, analyzes, or changes the file without the user's input. Using AWS Lambda, S3, Python, and Boto3, this article will show you how to build an event-driven file processor.
Prerequisites
Make sure you have an AWS account, basic Python and AWS knowledge, and the AWS CLI and Boto3 library in Python before beginning.
Step 1: Setting Up Your S3 Bucket
First, create an S3 bucket. Log in to AWS Management Console, choose S3, and click "Create Bucket." Name your bucket and choose an AWS region. After creating the bucket, activate event notifications. Add a s3:ObjectCreated event to the bucket settings' "Event Notifications" section. Let your Lambda function be the destination, we will setup it later.
Lambda function access requires bucket permissions. Make sure Lambda can read bucket files in the bucket policy.
Step 2: Configuring the Lambda Function
Open AWS Lambda console and create a new function to setup the Lambda function. Click "Author from scratch" and name your function. Use Python as the runtime. Attach an IAM role with S3 and CloudWatch Logs policies after building the function. The AmazonS3ReadOnlyAccess policy allows basic read rights.
A basic Lambda function structure:
import json
import boto3
def lambda_handler(event, context):
print("Event received:", json.dumps(event))
# Add your processing logic here
return {
'statusCode': 200,
'body': json.dumps('File processed successfully!')
}
This function will house the file processing logic we will create later.
Step 3: Linking S3 to Lambda
Set up the S3 console's bucket for connecting it to Lambda. Now you've to configure a Lambda function notification for file uploads under "Event Notifications". Set Lambda as the destination for the s3:ObjectCreated event type. In this setup, any bucket file upload triggers the function.
Step 4: Writing the Lambda Function Code
Enhance Lambda to handle uploaded files. An S3 file retrieval and logging function is below:
import json
import boto3
def lambda_handler(event, context):
s3 = boto3.client('s3')
# Extract bucket name and file key from the event
bucket_name = event['Records'][0]['s3']['bucket']['name']
file_key = event['Records'][0]['s3']['object']['key']
print(f"Processing file {file_key} from bucket {bucket_name}")
# Retrieve the file from S3
response = s3.get_object(Bucket=bucket_name, Key=file_key)
file_content = response['Body'].read().decode('utf-8')
# Example: Print the file content
print(f"File content: {file_content}")
return {
'statusCode': 200,
'body': json.dumps('File processed successfully!')
}
This function fetches S3 file content and reports it to CloudWatch. Replace content processing logic with file transformation or service sending. To monitor function performance and resolve difficulties, logging and error handling are necessary.
Testing the Workflow
Upload a file to S3 to test your setup. Use the AWS Management Console or CLI. To upload a file via CLI, use:
aws s3 cp testfile.txt s3://your-bucket-name/
The Lambda function's CloudWatch logs should show that it handled the file correctly after uploading. The logs should cover file content and actions.
Benefits of This Approach
This serverless design scales automatically to meet rising demands. Since you only pay for Lambda function calculate time, it is cost-effective. This configuration is versatile enough for image processing, log analysis, and data intake pipelines.
Conclusion
Using AWS Lambda and S3, you created an event-driven file processing system in this article. File uploads trigger automatic and scalable procedures using this structure. After building the system, you may add AWS services like DynamoDB or SNS. Start experimenting with this configuration to maximize serverless computing.
171 views