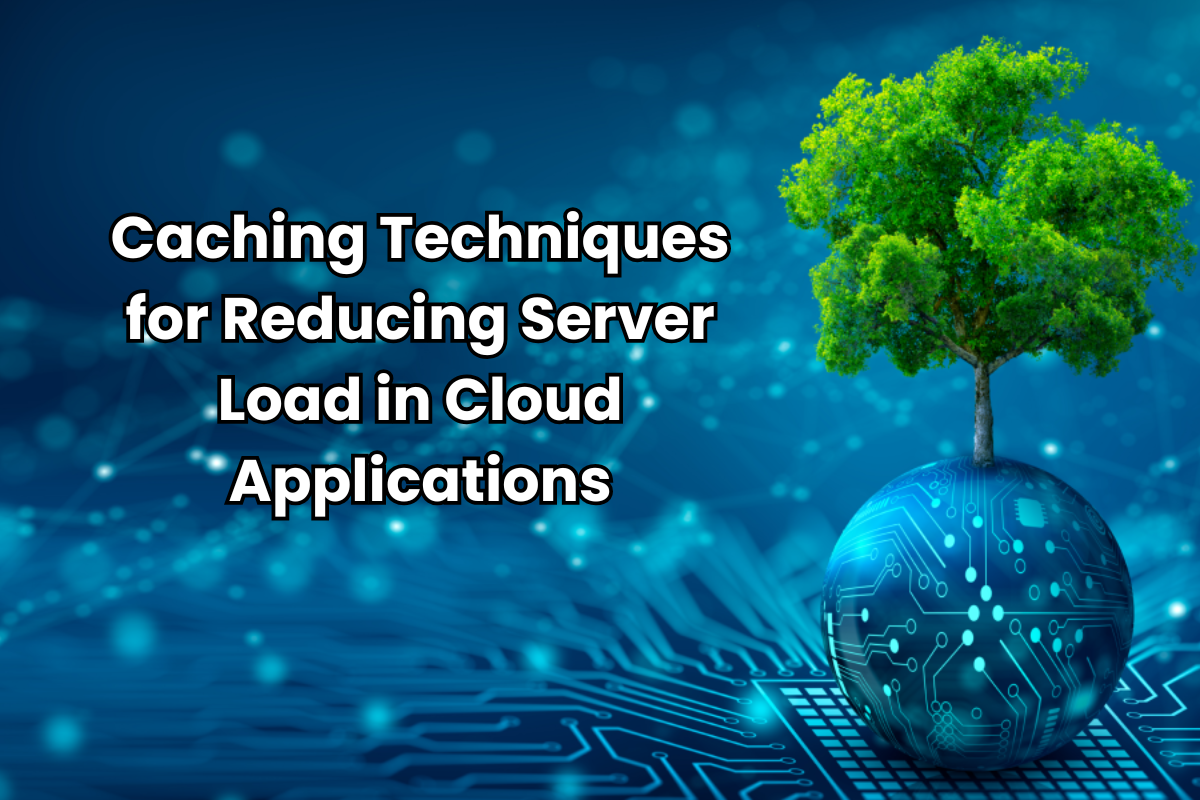
March 26, 2025
Caching Techniques for Reducing Server Load in Cloud Applications
Why do certain cloud apps remain quick and responsive under heavy load, while others crawl? The solution is frequently caching. Server load and latency may drastically increase cloud computing expenses and lower user experience. But what if you could significantly decrease server load and enhance performance with a few easy techniques? This blog post explains how caching can lower your cloud footprint and how to apply it in Node.js.
Why Caching Matters in Cloud Applications?
Cloud applications struggle with scale, latency, and operating expenses. Each time a user requests, the server may need to retrieve data from a database or compute, increasing response time and load. High traffic or complicated data processing might make this costly.
The real game-changer is caching. Caching minimizes the requirement to retrieve or compute frequently requested data by temporarily keeping it in memory. This speeds answers, reduces server strain, and most crucially, lowers expenses. It is a must-have approach for developers since it optimizes performance and cloud consumption easily.
Types of Caching Techniques
Caching methods vary by use case. Let's review the most popular:
- In-Memory Caching: Storage of data in memory for quick retrieval is one of the fastest caching methods. For in-memory caching, tools like Redis and Memcached are often used to make data that is often asked available without having to access the database.
- Database Query Caching: Caching database queries can reduce database load if your application uses them frequently. Data that seldom changes benefits from this.
- HTTP Response Caching: CDNs and browsers can cache static resources like images, CSS, and JavaScript. This speeds website load times and decreases server burden.
- Object Caching: Cache API replies and complicated data structures to prevent recalculating or obtaining them from the database. It is ideal for regularly used but stable data.
Implementing Caching in Node.js
Let's build Node.js caching. We will use Redis as our cache to keep things easy. This is ideal for Redis, an in-memory data storage.
This example shows how to cache API responses. Here's the procedure:
Set up a Node.js application
Run these commands to build a Node.js app:
mkdir node-cache-example
cd node-cache-example
npm init -y
npm install express redis
Install Redis and set up the Redis client
Use the redis Node.js client to communicate with Redis. Read the steps on their website to install Redis if you have not already.
Code Example:
const express = require('express');
const redis = require('redis');
const app = express();
const client = redis.createClient();
// Simulating a function that fetches data from a database
async function fetchDataFromDatabase() {
return { message: 'This is some fresh data from the database!' };
}
app.get('/data', async (req, res) => {
const key = 'dataKey';
client.get(key, async (err, cachedData) => {
if (cachedData) {
return res.json(JSON.parse(cachedData)); // Serve cached data if available
}
const freshData = await fetchDataFromDatabase();
client.setex(key, 3600, JSON.stringify(freshData)); // Cache the data for 1 hour (3600 seconds)
res.json(freshData); // Serve fresh data
});
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code initially checks whether the data is in the cache (client.get). We avoid the database call and provide it directly. If not cached, we request new data, save it in cache (client.setex), and provide it to the user. Cache data expires after 1 hour (3600 seconds) and must be reretrieved.
- Why It Works: Redis keeps the response in memory, making it quicker to retrieve than accessing the database. It maintains the application scalable and decreases database load.
Best Practices for Effective Caching
Caching is robust, but use it carefully. Let's see some recommended practices:
- Use appropriate TTL (Time-to-Live): Cache data should expire after a reasonable period to provide consumers new stuff without continuously hitting your database.
- Cache the right data: Focus on regularly accessible, static data. Avoid caching sensitive or frequently changed data to avoid stale or inconsistent results.
- Monitor your cache: Optimize performance by monitoring cache hits and misses using Redis Insights.
- Handle cache invalidation: When data changes, clean your cache. This prevents users from obtaining outdated facts.
Conclusion
Caching is one of the best ways to reduce server load and optimize resource use in cloud applications. Redis can cache data in memory to increase speed, save costs, and improve user experience. As seen with Node.js, caching is easy and can boost performance and scalability. Try caching your cloud apps now and experience the difference!
47 views