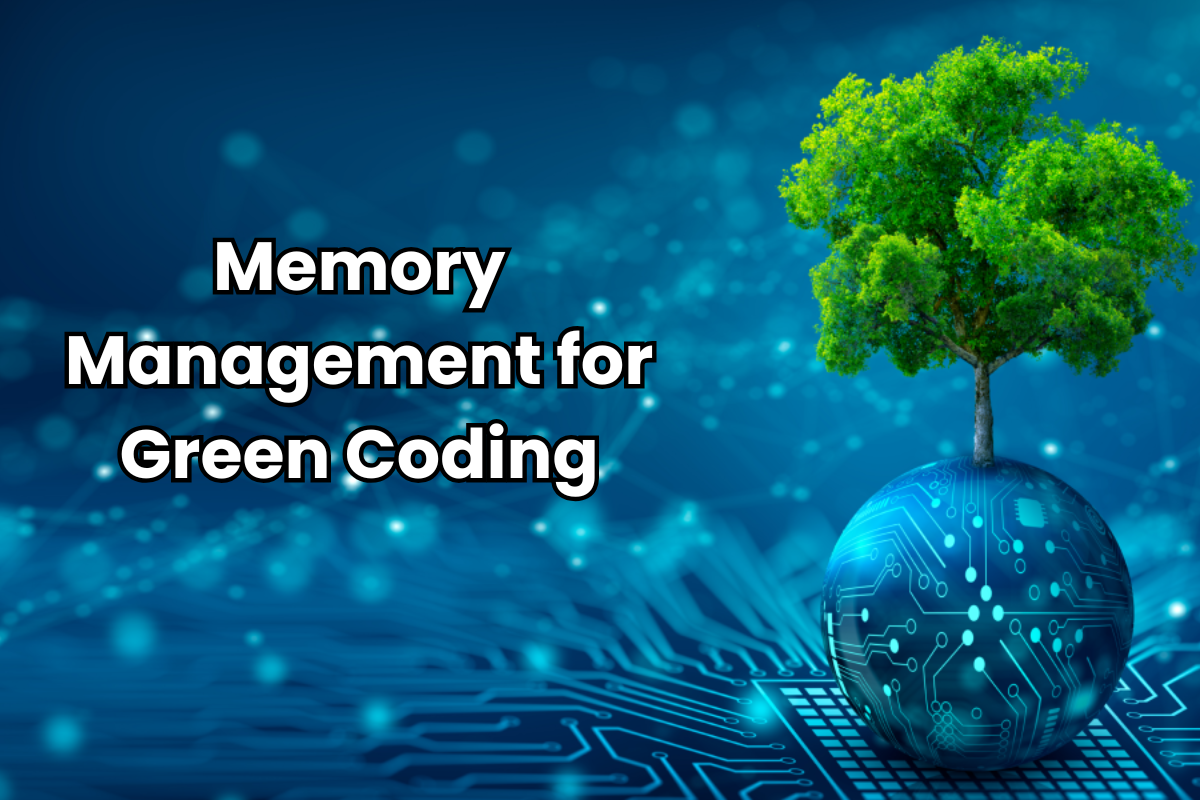
March 21, 2025
Memory Management for Green Coding: Reducing Wasteful Memory Usage
Have you considered your code's environmental impact? Programmers prioritize functionality and speed, but energy economy is also important. Every inefficient line of code wastes energy, particularly in huge systems. Memory management is crucial to effective, eco-friendly software. This tutorial will help you build memory-efficient C programs for greener coding.
Understanding Memory in Programming
Starting with the fundamentals. Programming uses stack and heap memory. The stack allocates static memory, local variables, while the heap allocates dynamic memory at runtime.
However, poor memory management may cause fragmentation, memory leaks, and overallocation. As your system works to manage wasteful memory, these issues impede your program and use more energy. As a code optimizer, I know that improved memory management improves performance and the environment.
Best Practices for Memory-Efficient Code
Let's get practical. How to develop memory-efficient code? Strategies that worked for me:
1. Minimizing Allocation Overhead
Use stack memory wherever feasible. It automatically deallocates and is quicker. As an example:
void example() {
int localVar = 10; // Stored on the stack
}
Use local variables instead of dynamically allocating RAM for tiny variables.
2. Avoiding Memory Leaks
Remember to clear memory after use. C requires matching every malloc or calloc with a free.
int *ptr = (int *)malloc(sizeof(int));
if (ptr != NULL) {
*ptr = 42;
free(ptr); // Prevents memory leaks
}
Deallocating memory seems simple, but people frequently forget, particularly in complex systems.
3. Optimizing Data Structures
It can make a big difference to pick the right data structure. For example, when the collection is small, arrays use less memory than linked lists.
int arr[5] = {1, 2, 3, 4, 5}; // More compact than a linked list
Look at your use case and choose the structure that has the least amount of additional overhead.
4. Pooling Memory
Do not allocate fresh memory blocks for repeated activities. Memory pooling saves time and costs in high-frequency activities like gaming and simulations.
Profiling and Debugging Tools for Memory Management
Debugging and profiling are as vital as writing efficient code. I use Valgrind to find memory leaks and inefficiencies. As an example:
valgrind --leak-check=full ./my_program
This simple command finds C program leaks. Other great memory-bug utilities include AddressSanitizer and gdb. You may optimize code performance and energy efficiency by profiling it often.
Real-World Impact: Memory-Efficient Software Saves Energy
Did you realize that wasteful memory consumption in one single program can waste millions of devices' energy? Companies have saved energy and money by using memory-efficient techniques. Optimizing server software for memory management reduces data center power consumption, benefiting business and the environment. Developers' memory optimizations help achieve sustainability objectives.
Conclusion
Today's sustainable civilization requires memory-efficient programming. By knowing memory usage, eliminating waste, and using the right tools you can create high-performing and eco-friendly software. So, what's next? Explore your projects, profile memory utilization, and optimize.
72 views