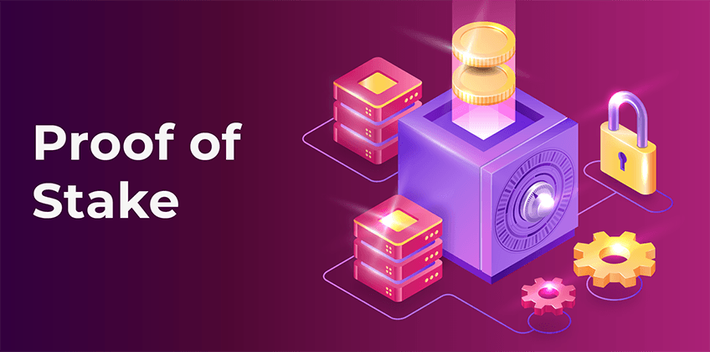
March 08, 2025
Implementing a Consensus Algorithm in Go: Proof of Stake (PoS)
How can cryptocurrencies like Ethereum be safe without using much resources? One of the most energy-efficient consensus techniques is Proof of Stake. How does PoS operate, and how can we use Go to implement it? This blogpost shows how to develop a basic Proof of Stake consensus algorithm in Go, which can power any blockchain network. Explore PoS and its role in decentralizing power while assuring security and scalability!
What is Proof of Stake (PoS)?
Blockchain networks agree on transaction authenticity and block generation via Proof of Stake. PoS chooses validators based on the amount of bitcoin they are prepared to "stake" or lock up as collateral, unlike Proof of Work (PoW), where miners compete to solve complicated cryptographic riddles.
PoS's main benefit is energy efficiency, no computationally demanding mining. Instead, validators construct new blocks according to their stake, with greater stakes having a better chance. This approach is greener and pays validators to perform honestly.
Setting Up the Go Environment
Let's set up Go before writing code. Download and install Go from its official website. Create a Go workspace and PoS project folder after installation.
Run this terminal command to verify Go installation:
go version
If configured properly, you should see Go version information. You can now code!
Key Components of the PoS Algorithm
PoS algorithms include many key components:
- Validators: Those who verify transactions and produce new blocks.
- Staking: Validators secure a certain quantity of cryptocurrency as collateral.
- Blocks: Transaction-holding units added to the blockchain.
- Transactions: Data for blockchain transactions need validation and storage.
The amount of cryptocurrency staked determines which validators verify the following block. Higher stakes increase their possibility of selection. This makes PoS fairer and scalable.
Coding the Basic PoS Algorithm
Here comes the fun, coding! Below is a simple Go PoS algorithm. The code provides validator setup, basic staking, and block construction.
package main
import (
"fmt"
"math/rand"
"time"
)
type Validator struct {
Name string
Stake int
}
type Block struct {
Transactions []string
Validator string
}
func main() {
// Create a list of validators
validators := []Validator{
{Name: "Validator1", Stake: 100},
{Name: "Validator2", Stake: 200},
{Name: "Validator3", Stake: 300},
}
// Choose a validator to create the next block
selectedValidator := selectValidator(validators)
// Create a new block
block := Block{
Transactions: []string{"Tx1", "Tx2", "Tx3"},
Validator: selectedValidator.Name,
}
// Print the block details
fmt.Println("New Block Created by:", block.Validator)
fmt.Println("Transactions:", block.Transactions)
}
// selectValidator chooses a validator based on their stake
func selectValidator(validators []Validator) Validator {
totalStake := 0
for _, v := range validators {
totalStake += v.Stake
}
// Select a random number between 0 and totalStake
rand.Seed(time.Now().UnixNano())
randomValue := rand.Intn(totalStake)
// Determine which validator is selected
var currentStake int
for _, v := range validators {
currentStake += v.Stake
if currentStake > randomValue {
return v
}
}
return Validator{}
}
Each of three validators with different stake levels can create new blocks in this code. A block includes transactions and the validator who produced it. The selectValidator method employs weighted random selection to verify and produce the next block. Validators with bigger stakes have a better probability.
Testing and Running the PoS Algorithm
Run the Go file to test our implementation:
Go run pos.
The output will display the validator used to produce a new block and its transactions. This creates a simple but effective PoS method that rewards validators by stake.
Conclusion
Implementing a Proof of Stake consensus algorithm in Go helps explain how blockchain networks accomplish decentralization and security without energy usage. Add more advanced validator selection algorithms, handle double-spending, and perform transaction verification to this basic version. Explore PoS and how to use it to blockchain applications.
109 views