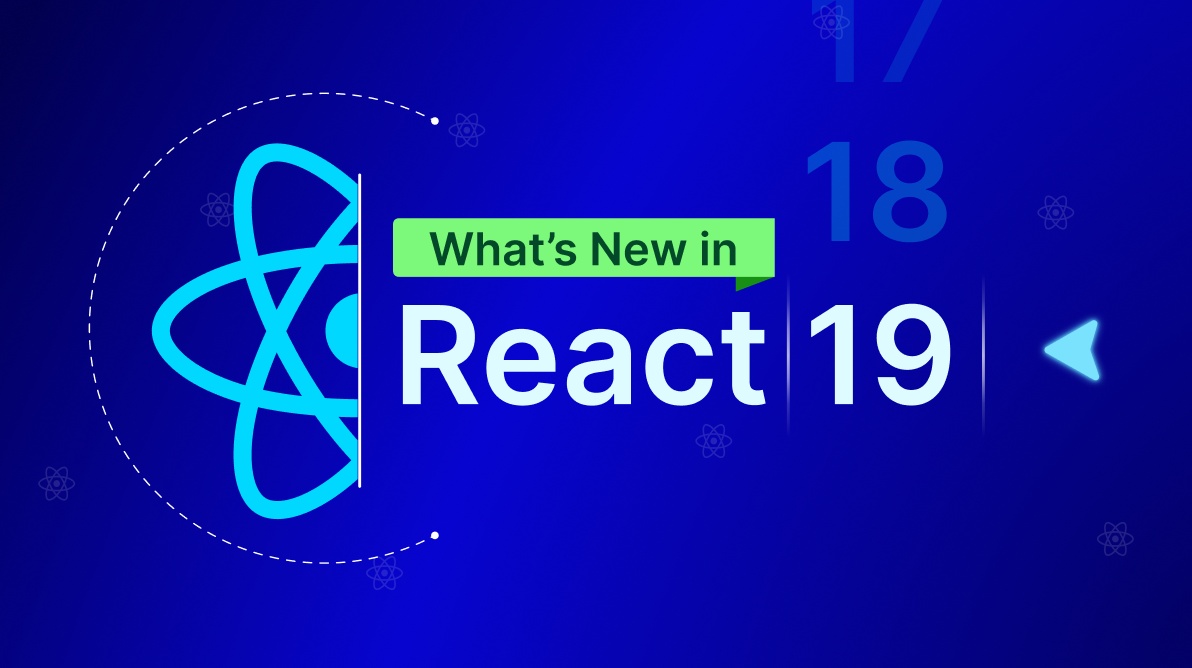
December 11, 2024
React 19 Updates: Resource Preloading, Hydration, Error Reporting, and Custom Elements
1. Resource Preloading
React 19 introduces APIs like prefetchDNS, preconnect, preload, and preinit to optimize resource loading and improve page performance.
Examples:
import { prefetchDNS, preconnect, preload, preinit } from 'react-dom'
function MyComponent() {
preinit('https://.../path/to/some/script.js', {as: 'script' }) // loads and executes this script eagerly
preload('https://.../path/to/font.woff', { as: 'font' }) // preloads this font
preload('https://.../path/to/stylesheet.css', { as: 'style' }) // preloads this stylesheet
prefetchDNS('https://...') // when you may not actually request anything from this host
preconnect('https://...') // when you will request something but aren't sure what
}
<!-- the above would result in the following DOM/HTML -->
<html>
<head>
<!-- links/scripts are prioritized by their utility to early loading, not call order -->
<link rel="prefetch-dns" href="https://...">
<link rel="preconnect" href="https://...">
<link rel="preload" as="font" href="https://.../path/to/font.woff">
<link rel="preload" as="style" href="https://.../path/to/stylesheet.css">
<script async="" src="https://.../path/to/some/script.js"></script>
</head>
<body>
...
</body>
</html>
These APIs improve:
Initial Page Loads: Faster discovery of resources like fonts and scripts.
Anticipated Navigation: Preloading resources on hover or click for smoother transitions.
2. Compatibility with Third-Party Scripts and Extensions
React 19 improves hydration by gracefully handling unexpected changes in the DOM introduced by third-party scripts or browser extensions:
Skipping Unexpected Tags: Elements like <script> or <style> added by third-party scripts no longer cause hydration mismatches.
Preserving Stylesheets: Styles injected by extensions remain intact even if React re-renders the document.
This ensures more robust hydration in environments with external script or extension interference.
3. Enhanced Error Reporting
React 19 refines error handling by:
Removing Duplication: Logs a single error instead of multiple messages for the same issue.
Providing More Context: Consolidates error details, showing the component tree and recovery attempt in one message.
New Error Boundary Callbacks:
onCaughtError: Called when an error is caught by an Error Boundary.
onUncaughtError: Triggered when an error isnât caught by any Error Boundary.
onRecoverableError: Called for errors automatically recovered by React.
These improvements simplify debugging and recovery in applications.
4. Full Support for Custom Elements
Custom Elements are now fully supported in React 19, with smarter handling of attributes and properties:
Server-Side Rendering (SSR):
Primitive values (e.g., strings, numbers, true) render as attributes.
Non-primitive values (objects, false) are omitted.
Client-Side Rendering (CSR):
Props matching a property on the Custom Element instance are assigned as properties.
Others are treated as attributes.
Example:
<my-custom-element some-prop="value" anotherProp={{ key: 'value' }}>
</my-custom-element>
This change makes React more compatible with modern web standards and ensures seamless integration with custom elements.
Conclusion
React 19 focuses on:
Optimizing Performance: Through preloading and resource prioritization.
Improving Robustness: Enhanced hydration handling and error reporting.
Expanding Compatibility: Better support for Custom Elements.
For more information, see the official React docs
630 views