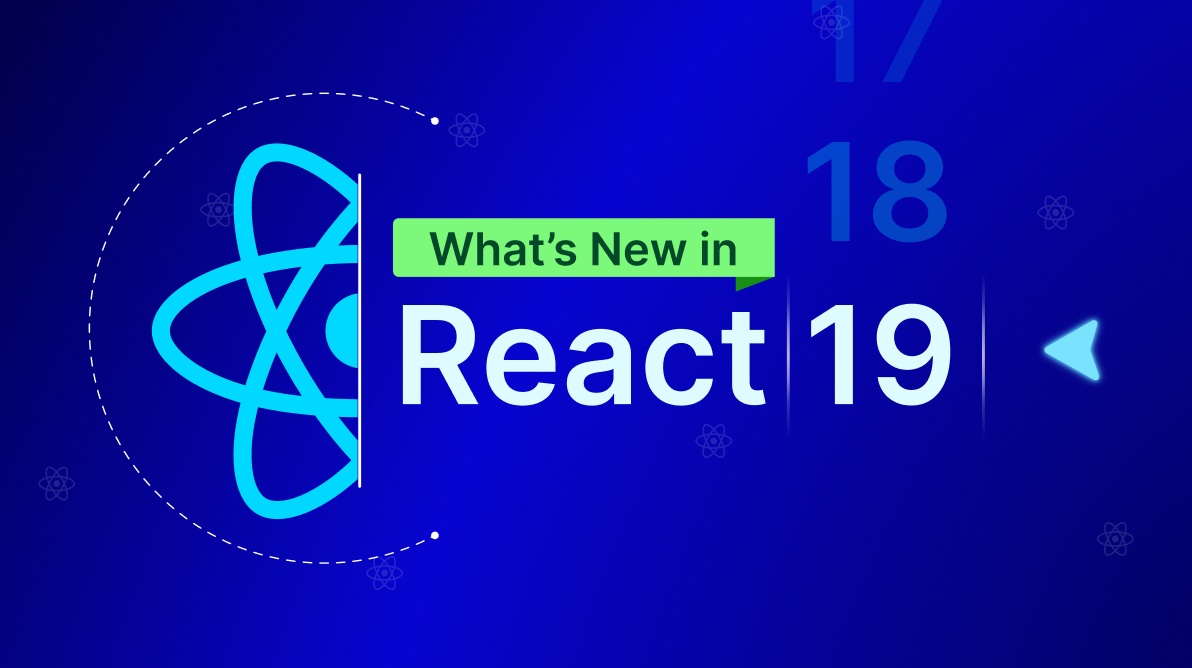
December 11, 2024
React 19 Updates: Metadata, Stylesheets, and Async Scripts
1. Native Support for Document Metadata
React 19 simplifies how document metadata (e.g., <title>, <link>, <meta>) is managed within components. These tags can now be rendered directly in components, and React will hoist them to the <head> section.
Example:
function BlogPost({ post }) {
return (
<article>
<h1>{post.title}</h1>
<title>{post.title}</title>
<meta name="author" content="Josh" />
<meta name="keywords" content={post.keywords} />
<link rel="author" href="https://twitter.com/joshcstory/" />
<p>E = mcò...</p>
</article>
);
}
Key Benefits:
Automatic Hoisting: Metadata tags are automatically moved to the <head> section, making it compatible with both client-side rendering (CSR) and server-side rendering (SSR).
Ease of Use: No need for additional libraries like react-helmet for simple metadata handling.
Streaming Support: Works seamlessly with Reacts Streaming SSR and Server Components.
Note:
Libraries like react-helmet are still useful for advanced use cases, such as route-specific metadata overriding.
2. Built-in Stylesheet Management
React 19 introduces native support for stylesheets, improving their integration with concurrent rendering and streaming SSR.
Example:
function ComponentOne() {
return (
<Suspense fallback="loading...">
<link rel="stylesheet" href="foo.css" precedence="default" />
<link rel="stylesheet" href="bar.css" precedence="high" />
<article className="foo-class bar-class">
{/* Content */}
</article>
</Suspense>
);
}
Key Features:
Precedence Management: Stylesheets are inserted into the DOM in the correct order based on their precedence.
Streaming SSR: Ensures stylesheets are included in the <head> early, preventing visual flashes.
Deduplication: React ensures a stylesheet is only loaded once, even if referenced multiple times.
Suspense Integration: Content dependent on stylesheets is not rendered until the stylesheets are loaded.
Use Case:
Stylesheets can now be colocated with the components that depend on them, improving modularity and load efficiency.
3. Support for Async Scripts
React 19 enhances the handling of <script> tags, particularly for async scripts, allowing them to be colocated with the components that use them.
Example:
function MyComponent() {
return (
<div>
<script async={true} src="my-script.js"></script>
Hello World
</div>
);
}
Key Features:
Deduplication: React ensures that async scripts are only executed once, even if rendered multiple times.
Priority Handling: During SSR, async scripts are included in the <head> but are prioritized behind critical resources like stylesheets.
Streaming-Friendly: Works seamlessly with Reacts streaming architecture.
Summary
React 19 introduces significant enhancements to better handle document metadata, stylesheets, and scripts:
Document Metadata: Simplifies <title>, <meta>, and <link> management directly in components.
Stylesheets: Adds precedence and deduplication to stylesheet management, improving performance and modularity.
Async Scripts: Ensures scripts are deduplicated and integrated efficiently with SSR and CSR.
398 views