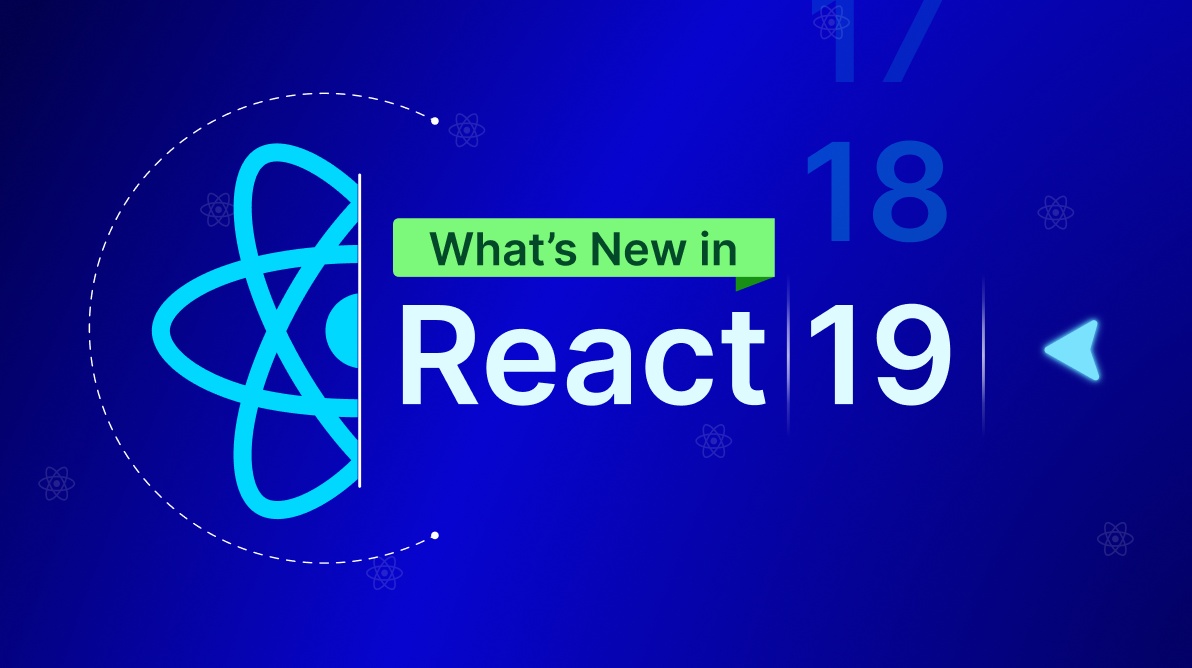
December 10, 2024
What’s New in React — New Use Api, New React DOM Static APIs
React 19 introduces several powerful features, including the new use API and enhanced static rendering APIs for better resource management and static site generation. Here is a breakdown of these updates:
New API: use
The use API allows you to consume asynchronous resources or context directly in the render phase, enabling more flexible handling of data and context within components.
Features of use
Reading Promises:
You can pass a promise to use, and React will suspend rendering until the promise resolves.
Ideal for working with suspense-compatible libraries where promises are pre-cached.
Example:
import { use } from 'react';
function Comments({ commentsPromise }) {
const comments = use(commentsPromise);
return comments.map(comment => <p key={comment.id}>{comment.text}</p>);
}
function Page({ commentsPromise }) {
return (
<Suspense fallback={<div>Loading comments...</div>}>
<Comments commentsPromise={commentsPromise} />
</Suspense>
);
}
Reading Context Conditionally:
Unlike useContext, the use API can be used conditionally.
This is useful for cases with early returns or conditional logic.
Example:
import { use } from 'react';
import ThemeContext from './ThemeContext';
function Heading({ children }) {
if (!children) return null;
const theme = use(ThemeContext);
return <h1 style={{ color: theme.color }}>{children}</h1>;
}
Error Handling:
React issues warnings if you pass promises created in render (e.g., from async calls in the render function). To resolve this, use suspense-compatible libraries that cache promises.
Limitations
You cannot pass promises created within render. Instead, use cached promises from suspense-powered libraries.
It can only be used in the render phase, similar to hooks, but unlike hooks, it can be used conditionally.
New React DOM Static APIs
React 19 introduces prerender and prerenderToNodeStream in react-dom/static for static site generation (SSG). These APIs are designed for environments like Node.js or Web Streams.
Features
Data Loading Before Rendering:
The prerender API waits for all data to load before returning the static HTML.
Unlike existing server-side rendering (SSR) APIs, prerender focuses on complete static generation rather than streaming content.
Web Stream Example:
import { use } from 'react';
import ThemeContext from './ThemeContext';
function Heading({ children }) {
if (!children) return null;
const theme = use(ThemeContext);
return <h1 style={{ color: theme.color }}>{children}</h1>;
}
Advantages:
Prepares fully-rendered HTML for static delivery.
Integrates seamlessly with modern web streaming environments.
Comparison to SSR:
Prerender: Focuses on generating complete HTML before returning.
Streaming APIs: Stream content as it becomes available.
Key Benefits
use API: Simplifies consuming asynchronous data and context in render with suspense.
Static Rendering APIs: Provides new options for static site generation, supporting modern deployment needs with improved performance and flexibility.
545 views