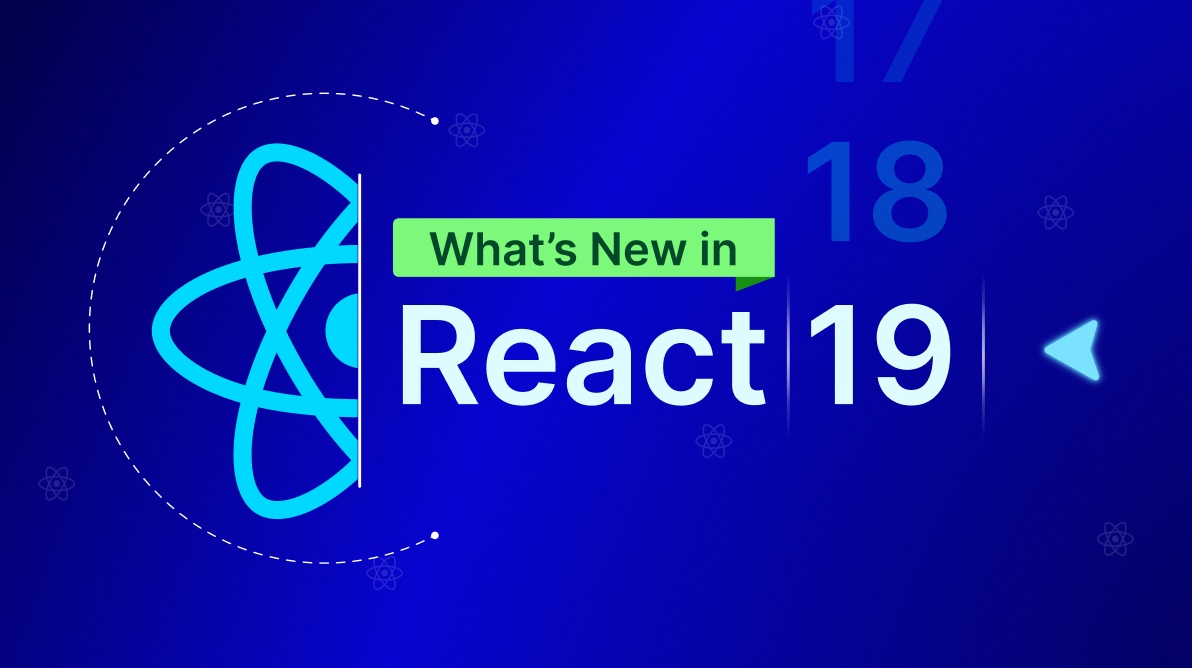
December 10, 2024
What's New in React 19: Actions
React 19 introduces Actions as an enhancement for managing async operations in transitions, making handling data mutations like form submissions or API requests more intuitive and efficient. Here is a deeper dive into Actions and how they simplify the developer experience:
Before React 19 (Manual Pending and Error Handling)
Previously, handling pending states, errors, and updates required managing everything manually with useState. This approach was verbose, repetitive, and error-prone, especially for larger apps:
function UpdateName() {
const [name, setName] = useState("");
const [error, setError] = useState(null);
const [isPending, setIsPending] = useState(false);
const handleSubmit = async () => {
setIsPending(true);
try {
await updateName(name);
redirect("/path");
} catch (err) {
setError(err.message);
} finally {
setIsPending(false);
}
};
return (
<div>
<input value={name} onChange={(e) => setName(e.target.value)} />
<button onClick={handleSubmit} disabled={isPending}>
Update
</button>
{error && <p>{error}</p>}
</div>
);
}
React 19: Introducing Actions
React 19 leverages Actions to handle async operations with less boilerplate. By combining the power of useTransition and async transitions, Actions automatically manage the pending state for you.
Using Actions for Simplified Transitions
With useTransition, you no longer need to manage isPending or error handling manually. React handles these aspects while keeping the UI interactive during background tasks:
function UpdateName() {
const [name, setName] = useState("");
const [error, setError] = useState(null);
const [isPending, startTransition] = useTransition();
const handleSubmit = () => {
startTransition(async () => {
try {
await updateName(name);
redirect("/path");
} catch (err) {
setError(err.message);
}
});
};
return (
<div>
<input value={name} onChange={(e) => setName(e.target.value)} />
<button onClick={handleSubmit} disabled={isPending}>
Update
</button>
{error && <p>{error}</p>}
</div>
);
}
What Makes Actions Powerful?
Automatic Pending State:
No need to manually toggle a loading or pending state.
React handles when to show and hide the pending indicator during async operations.
Error Handling:
Errors are caught in the async transition and can be displayed as needed without complicating the component logic.
Optimistic UI Updates:
Actions work seamlessly with optimistic UI updates (e.g., updating the UI instantly while awaiting server confirmation).
Benefits for Developers
Reduced Boilerplate: Actions drastically reduce the lines of code needed to manage async operations.
Responsive UI: The app remains interactive even during long-running tasks, improving user experience.
Easier Debugging: Transitions provide a clear lifecycle for handling pending states and errors.
When to Use Actions?
- Form submissions
- API mutations (e.g., updating or deleting data)
- Tasks requiring optimistic updates
Actions are part of Reacts broader effort to streamline complex tasks and improve user experiences by reducing common pitfalls in async operations. To try Actions, make sure your project uses React 19 and the latest react-dom package.
Key Features of Actions in React 19
Pending State: Actions automatically track and manage the pending state, starting it at the beginning of an async request and resetting it when the request completes. This eliminates the need for manual state management with useState.
Optimistic Updates: Optimistic UI updates are handled using the new useOptimistic hook, which allows users to see instant feedback (e.g., UI changes) while waiting for the backend response.
Error Handling: When an error occurs, Actions automatically revert optimistic updates to their original state and provide easy error handling, such as displaying error boundaries.
Form Enhancements:
<form> elements now support an action prop that directly references a function, making form submissions easier to manage.
Forms automatically reset after submission, reducing boilerplate code.
New Hooks:
useActionState: Simplifies managing the state and lifecycle of an Action, including pending states, errors, and results.
useFormStatus: Handles common form specific cases, such as managing errors or checking if a form submission is pending.
Example Using <form> Actions and useActionState
This example showcases how the useActionState hook and <form> Actions streamline handling form submissions:
function ChangeName({ name, setName }) {
const [error, submitAction, isPending] = useActionState(
async (previousState, formData) => {
const error = await updateName(formData.get("name"));
if (error) {
return error;
}
redirect("/path");
return null;
},
null
);
return (
<form action={submitAction}>
<input type="text" name="name" />
<button type="submit" disabled={isPending}>Update</button>
{error && <p>{error}</p>}
</form>
);
}
How useActionState Works
Action Function: The first argument to useActionState is an async function that defines the Action. It accepts the previous state and any additional data (e.g., form input).
Return Values:
- error: The result of the Action, typically used to handle errors.
- submitAction: A wrapped version of the Action to call when needed.
- isPending: A boolean that tracks the pending state of the Action.
useFormStatus
React 19 introduces a new hook called useFormStatus, which makes it easier to access information about the status of a form without having to drill props down to child components. This is particularly useful in design systems where components like buttons or inputs might need to interact with a form's state.
How it Works:
The useFormStatus hook provides information about the current status of the form it is within, specifically whether the form submission is "pending." This is often used to disable a submit button while the form is submitting, preventing multiple submissions.
Example Usage:
import { useFormStatus } from 'react-dom';
function DesignButton() {
const { pending } = useFormStatus();
return <button type="submit" disabled={pending} />;
}
pending: This value indicates whether the form is currently in a pending state, typically during submission or when asynchronous actions are being processed.
Usage: The button in this example will be disabled when the form is in a pending state, which is commonly used to prevent multiple submissions while the form is still processing.
Optimistic Updates with useOptimistic
For cases where you want to provide instant feedback before the server response, you can use useOptimistic:
const [state, setOptimistic] = useOptimistic(initialState, reducer);
function handleUpdate(newValue) {
setOptimistic({ type: "update", payload: newValue });
updateOnServer(newValue).catch(() => {
setOptimistic({ type: "revert" });
});
}
Here, useOptimistic helps manage the local state update while awaiting confirmation from the server, rolling back if necessary.
Advantages of Actions in React 19
Less Boilerplate: Automatically managed states and errors reduce repetitive code.
Improved Developer Experience: Simplified API for handling async tasks.
Enhanced User Experience: Responsive UI with seamless transitions and error handling.
React 19 introduction of Actions, combined with useActionState, marks a significant step toward making React apps more intuitive and efficient. This new approach minimizes common pitfalls and enables developers to build richer user experiences with less effort.
602 views