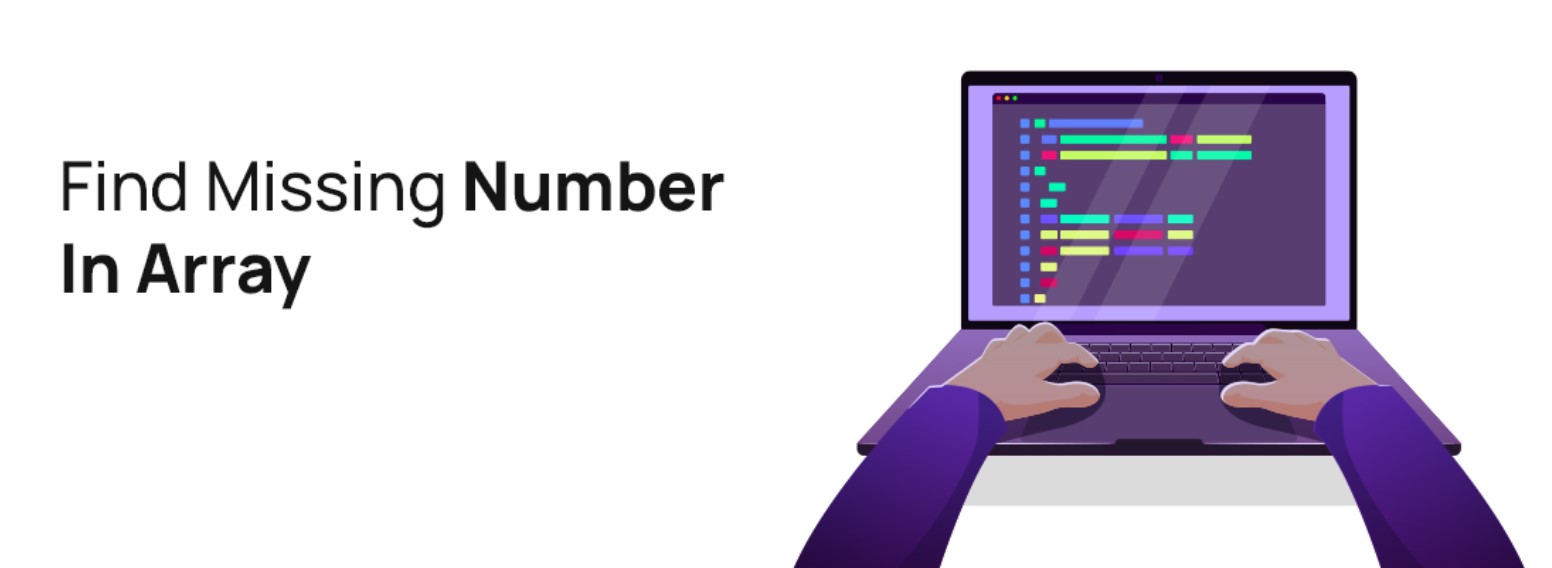
February 20, 2025
Finding the Missing Number in an Array: Coding Techniques and Best Practices
Ever confused by missing data in a list of numbers? One missing number might throw off your calculations when debugging a program or solving a coding problem. This "missing number" challenge is common in competitive coding and programming interviews. When time complexity is included, tackling this issue efficiently can make or break a task.
This blog post will discuss linear-time strategies for finding the missing number in an array. Let's get started.
Understanding the Problem Statement
The typical problem statement is to discover the missing number in an array of N-1 unique numbers from 1 to N.
For example, the array [1, 2, 4, 5] lacks 3.
The dataset may start at 0 or be negative. The approach is the same, but you must know the limitations before solving it.
1. Brute Force Approach
For the brute force method, you check each number in the range from 1 to N to see if it is in the array. Missing numbers are returned.
Code Example
def find_missing_brute(nums, n):
for i in range(1, n + 1):
if i not in nums:
return i
Time Complexity
The approach is inefficient for big datasets since it checks for each integer in the range in the array (O(N^2)).
Why It's Not Ideal
Nested loops make this method inefficient as datasets grow.
2. Sorting Approach
After sorting the array, verify each element's expected value. Identify the missing number otherwise.
Code Example
def find_missing_sort(nums):
nums.sort()
for i in range(len(nums)):
if nums[i] != i + 1:
return i + 1
Time Complexity
Sorting requires O(N log N) time, making it less efficient than linear solutions.
Why It's Not Ideal
Sorting works, but it is inefficient for discovering one missing number.
3. Using Hashing
Create a hash set of the array numbers, then iterate across the range to find the missing number.
Code Example
def find_missing_hash(nums, n):
num_set = set(nums)
for i in range(1, n + 1):
if i not in num_set:
return i
Time Complexity
Adding items to the hash set and looking to see if they are in the set take O(N) time.
Space Complexity
O(N): Hash set needs more space.
Best Use Case
Best for time efficiency and increased space.
4. XOR Method
Using XOR (exclusive OR), cancel identical integers to leave the missing number.
Code Example
def find_missing_xor(nums, n):
xor_all = 0
xor_nums = 0
for i in range(1, n + 1):
xor_all ^= i
for num in nums:
xor_nums ^= num
return xor_all ^ xor_nums
Time Complexity
O(N): One single pass through the numbers.
Space Complexity
O(1): No space required.
Why It Works
Only the missing number remains after XORing similar numbers.
5. Sum Formula Method
For the first N natural integers, use arithmetic sum formula: Sum = N * (N+1)/2. Take this number and subtract the array's real sum to get the missing number.
Code Example
def find_missing_sum(nums, n):
expected_sum = n * (n + 1) // 2
actual_sum = sum(nums)
return expected_sum - actual_sum
Time Complexity
One single pass across the array calculates the total in O(N).
Space Complexity
The space complexity is O(1), requiring no additional space.
Limitations
Modern languages handle big sums effectively, although integer overflow could occur for excessively large sums.
Conclusion
Finding the missing number in an array is a frequent yet important coding interview and real-world topic. Hashing, XOR, and sum formulas are some of the methods you can use to solve it quickly and correctly. These strategies and when to utilize them will allow you to confront this difficulty in any coding situation. Try these strategies to improve your problem-solving!
392 views