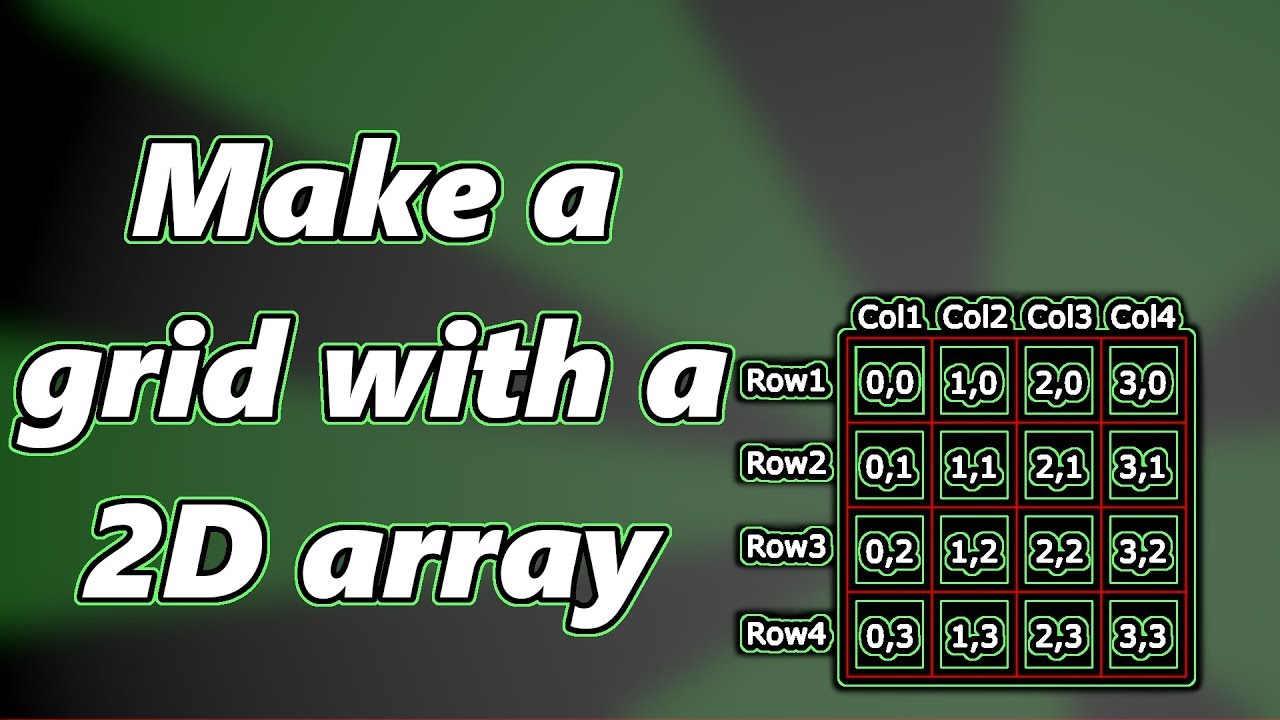
February 19, 2025
Implementing Two-Dimensional Arrays for Game Boards and Grids
Have you ever noticed how the games like Tic-Tac-Toe, Minesweeper, and Chess monitor movements and positions? This is because these games use 2D arrays which is a basic yet powerful data structure. The developers can manipulate rows and columns, monitor player movements, and change game states using these grids. You can construct interactive, grid-based games using 2D arrays, regardless of your coding skills. I will use Tic-Tac-Toe and Minesweeper to show how to design and maintain game boards using 2D arrays.
Basics of Two-Dimensional Arrays
Lists or arrays make up a 2D array. A 2D array has row and column indexes for each entry. Here's a simple Python 3x3 example:
grid = [
[0, 0, 0],
[0, 0, 0],
[0, 0, 0]
]
Grid[0][0] is the top-left cell and grid[2][2] is the bottom-right cell. For rows and columns, index starts at 0. This layout is ideal for grid-based games.
Example 1: Creating a Tic-Tac-Toe Board
The traditional 3 x 3 grid game Tic-Tac-Toe involves alternately putting X and O until one player obtains three in a row. Try a simple Tic-Tac-Toe board.
Steps to Implement:
- Initialize a 3x3 grid with empty cells ('-').
- Display the board.
- Make moves and update the board.
Hereââ¬â¢s a simple implementation in Python:
def create_board():
return [['-' for _ in range(3)] for _ in range(3)]
def display_board(board):
for row in board:
print(" | ".join(row))
print()
board = create_board()
display_board(board)
The function create_board() starts a 3x3 board with empty cells marked with a "-." and display_board(board) prints each row, which makes it easy to see what is going on.
To make a move, update a specific cell:
board[0][0] = 'X' # Player X makes a move in the top-left cell
display_board(board)
This sets the stage for a simple but functional Tic-Tac-Toe game.
Example 2: Implementing a Simple Minesweeper Grid
Another grid-based game, Minesweeper, requires avoiding hidden mines in cells. Let's make a simple Minesweeper grid.
Steps to Implement:
- Define the grid size and the number of mines.
- Initialize the grid with empty cells ('.').
- Randomly place mines on the grid.
Here's a practical example in Python:
import random
def create_minesweeper_board(size, num_mines):
board = [['.' for _ in range(size)] for _ in range(size)]
mines_placed = 0
while mines_placed < num_mines:
row = random.randint(0, size - 1)
col = random.randint(0, size - 1)
if board[row][col] != 'M':
board[row][col] = 'M'
mines_placed += 1
return board
def display_board(board):
for row in board:
print(" ".join(row))
print()
# Create a 5x5 board with 5 mines
board = create_minesweeper_board(5, 5)
display_board(board)
The create_minesweeper_board() function creates a grid of size size x size and randomly puts num_mines. Mine ('M') placement refers to cells without existing mines. The display_board() function shows placement.
Adding logic to compute nearby mine counts makes this a functioning Minesweeper game.
Common Operations for 2D Game Boards
In grid-based games, common operations are required:
- Check Cell Neighbors: Use offsets to inspect neighboring cells in Minesweeper:
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
Reset the Board: Clear the grid for a new round by reinitializing it.
Validate Moves: Ensure player moves are within the grid bounds:
if 0 <= row < len(board) and 0 <= col < len(board[0]):
# Valid move
Conclusion
Many grid-based games use two-dimensional arrays. Mastering them lets you design basic games like Tic-Tac-Toe and more complicated ones like Minesweeper. Ready to level up? Implement your own grid-based game for executing your ideas!
425 views