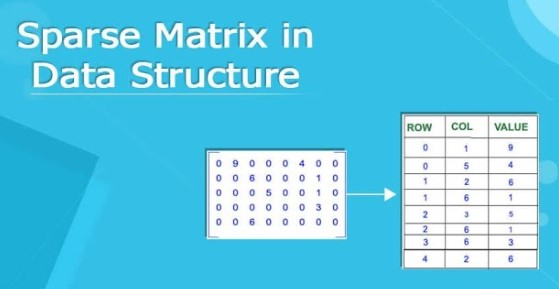
February 18, 2025
Sparse Arrays: Storing and Accessing Large, Sparse Data Efficiently
Have you worked with a dataset containing mostly 0 values? Imagine placing a huge matrix or array in memory and finding 90% empty or filled with zero. Traditional arrays waste memory dealing with this. This inefficiency can slow machine learning, image processing, and geographic data and use too much memory. Thankfully, sparse arrays help. Optimizing how we store and retrieve empty elements can boost program efficiency.
What are Sparse Arrays?
Most sparse array elements are empty or have default values like zero. Unlike dense arrays, where most items contain useful data. Sparse arrays do not explicitly store empty or default elements, conserving memory.
Consider a dense array like this:
dense = [0, 0, 5, 0, 0, 0, 3, 0, 0]
This scenario has just two relevant values (5 and 3). A sparse array representation stores just non-zero values and their places, making it more efficient.
Memory Inefficiencies of Dense Arrays
Dense arrays store all elements regardless of importance. This wastes memory in large zero- or placeholder-heavy databases.
A dense array would allocate memory for every 100 million elements in a 10,000 x 10,000 matrix with 90% zeros! This inefficiency is even worse when dealing with systems that do not have a lot of resources or real-time apps.
Storing Sparse Arrays Efficiently
Sparse arrays concentrate on non-zero elements to save memory. The most prevalent storage formats:
1. Coordinate List (COO) Format:
The Coordinate List (COO) format stores non-zero values with their index or coordinates. This format is easiest to understand.
sparse = [(2, 5), (6, 3)]
This represents the values 5 at index 2 and 3 at index 6.
2. Compressed Sparse Row (CSR) and Compressed Sparse Column (CSC) Formats:
These advanced formats are efficient for big matrices. They store data to speed up calculations, notably linear algebra.
CSR compresses rows, and CSC columns. Non-zero values and row/column changes are stored in both.
When to Use Each Format:
- COO is suitable for basic datasets or dynamic array creation.
- CSR/CSC excel on huge datasets with matrix operations like multiplication or equation solving.
Accessing Data in Sparse Arrays
Effective data storage and access are equally critical. Memory savings and retrieval performance trade off in sparse arrays.
1. Accessing Data in COO Format:
To access a COO element, search the stored list for the index. Default value is zero if not found.
def get_value(sparse, index):
for i, val in sparse:
if i == index:
return val
return 0
sparse = [(2, 5), (6, 3)]
print(get_value(sparse, 2)) # Output: 5
2. CSR/CSC Formats:
Matrix operations and sequential access are quicker with these formats. Although dense arrays are faster, random access is slower.
Libraries and Tools for Sparse Arrays
Many programming languages provide sparse array libraries, making them simpler to use in practical applications.
Python:
- SciPy (scipy.sparse): Supports COO, CSR, and CSC formats.
- NumPy: Designed for dense arrays, can communicate with sparse formats via SciPy.
Example of using scipy.sparse to create a CSR matrix:
from scipy.sparse import csr_matrix
matrix = csr_matrix(([5, 3], ([0, 2], [2, 1])), shape=(3, 3))
print(matrix)
C++:
- Eigen Library: Supports sparse matrices and operations efficiently.
Benefits and Trade-offs of Sparse Arrays
Benefits:
- Memory Efficiency: Minimizes memory use by preventing empty storage.
- Performance Gains: Reduce calculation time for huge datasets by considering only non-zero elements.
Trade-offs:
- Complexity: Data access is more challenging than with simple dense arrays.
- Slower Random Access: For example, retrieving individual items in COO format can be slower than in dense arrays.
Conclusion
Datasets containing mostly empty or default values need sparse arrays. Memory optimization allows them to store and compute enormous amounts of data efficiently. Their memory savings and efficiency make them essential for modern uses like machine learning and scientific computing, despite their complexity. Sparse arrays are efficient!
182 views