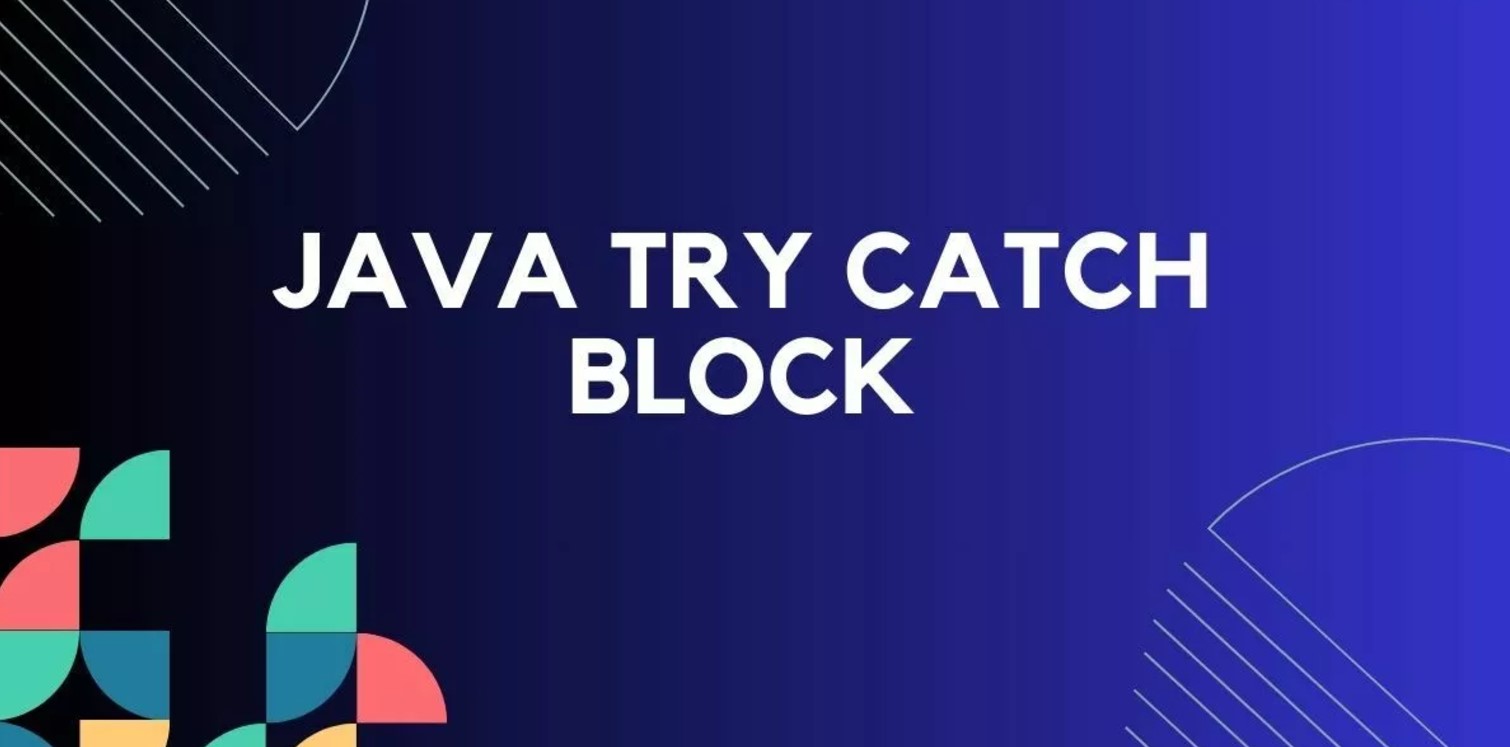
February 06, 2025
Understanding Try-Catch Blocks: Writing Robust Java Programs
How do Java apps handle unexpected errors? Have you ever had an unhandled exception crash your program? Then you are not alone. Exception handling is essential for developing trustworthy Java applications. The try-catch block is a key error-handling feature that prevents applications from crashing. To create fault-tolerant code, we will cover try-catch blocks, recommended practices, and error logging in this article.
What is a Try-Catch Block?
Java exceptions interrupt program execution. Java uses the try-catch block to handle exceptions and avoid crashes.
A try block wraps exception-prone code. In the catch block, you can declare how to handle exceptions. The fundamental syntax is:
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
The catch block executes when the try block throws an exception. Skip the catch block if no exception occurs. This makes try-catch blocks effective for avoiding crashes and controlling mistakes.
Types of Exceptions in Java
The Java exception model includes checked and unchecked exceptions.
- Checked Exceptions are compiler-mandated. You must catch checked exceptions in a try-catch block or specify them in the method signature using throws. Example checked exceptions are IOException and SQLException.
- Unchecked Exceptions needs no handling or declaration. These exceptions frequently result from programming errors like accessing null objects or dividing by zero. Example: NullPointerException and ArithmeticException.
Understanding the difference between these two categories helps determine which exceptions must be handled directly and which implicitly or disregarded. To minimize computer crashes, handle unchecked exceptions carefully.
Handling Multiple Exceptions
Java try blocks can handle multiple exceptions. You can handle each exception type independently with several catch blocks. As an example:
try {
// Some risky code
} catch (IOException e) {
// Handle IOException
} catch (SQLException e) {
// Handle SQLException
}
A multi-catch block can handle multiple exceptions in one catch block. This helps when numerous exception handling logic is similar:
try {
// Some risky code
} catch (IOException | SQLException e) {
// Handle both IOExceptions and SQLExceptions
}
This makes code more simple and maintainable, particularly for several exceptions with identical handling processes.
Best Practices for Using Try-Catch Blocks
Try-catch blocks need best practices for clear, efficient error-handling code.
- Limit the scope of try-catch blocks: Only surround exception-prone code. Try blocks can conceal flaws and make debugging difficult, therefore avoid them for big code portions.
- Catch specific exceptions: Target particular exceptions like IOException and SQLException instead of Exception. You can handle problems more accurately and offer better error messages.
- Use the finally block for cleanup: Cleanup code for file or database closures should go in the finally section. This block runs regardless of exceptions.
try {
// Code that might throw exception
} catch (IOException e) {
// Handle exception
} finally {
// Code to release resources
}
Following these recommended practices will make your code more resilient, readable, and manageable.
Logging Errors
Java exception handling requires good logging. It lets you monitor mistakes, troubleshoot issues, and enhance application reliability.
Java's built-in java.util.logging package and third-party libraries like Log4j and SLF4J can record failures. To find the cause of an exception, record both the error message and stack trace.
Example of logging an exception with java.util.logging:
catch (IOException e) {
logger.severe("IOException occurred: " + e.getMessage());
e.printStackTrace(); // Optionally, log the stack trace for debugging
}
Conclusion
Java developers must use try-catch blocks to handle exceptions to build fault-tolerant apps. Know try-catch blocks, exceptions, and best practices to prevent crashes and improve user experience. Error handling involves thorough logging to record and evaluate problems. Use these Java development methods to create more stable, professional, and long-lasting apps.
778 views