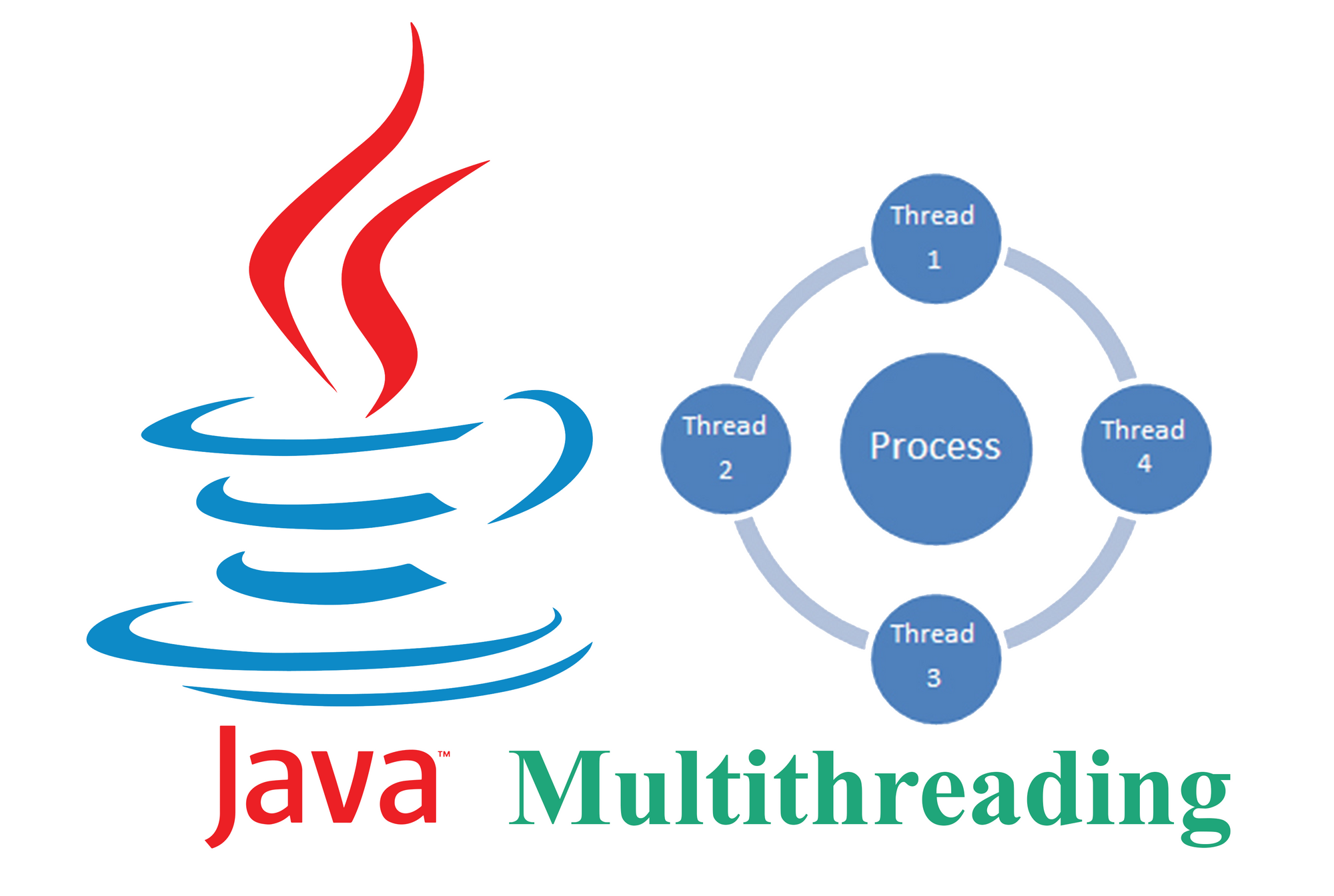
February 05, 2025
Starting with Multithreading: Creating and Managing Threads in Java
How effectively do your favorite applications multitask? Smooth performance often comes from multithreading. For responsive, efficient apps, we will examine Java thread generation and management. Proper multithreading speeds up app performance. Start with Java thread creation, management, and synchronization.
Understanding Threads in Java
Before managing threads, we must define them. Java processes have threads, the smallest execution units. A thread lets your application receive input, process data, and handle user requests concurrently. Multithreading boosts application speed by optimizing CPU use.
Creating a Thread in Java
Extending the Thread class or implementing Runnable are the main approaches to construct a thread in Java. Watch how this works.
- Extending the Thread Class: When you extend the Thread class, you override run() to specify the thread's job.
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running!");
}
}
public class Main {
public static void main(String[] args) {
MyThread t = new MyThread();
t.start(); // Starting the thread
}
}
- Implementing the Runnable Interface: Another option is to implement Runnable and create the run() function. This method is best for classes that inherit from others.
class MyRunnable implements Runnable {
public void run() {
System.out.println("Runnable thread is running!");
}
}
public class Main {
public static void main(String[] args) {
Thread t = new Thread(new MyRunnable());
t.start();
}
}
Managing Threads in Java
Once a thread starts, controlling it is vital. Java has several thread-controlling methods for starting, pausing, and stopping threads.
Thread Lifecycle
Java threads can be New, Runnable, Blocked, Waiting, or Terminated. How can you manage a thread's behavior at various phases using start(), sleep(), join(), and interrupt()? Let's see:
- start(): This function starts the thread and executes run().
- sleep(): Pauses the thread for milliseconds.
- join(): Waits for another thread to finish.
The following example uses join() and sleep():
class ThreadExample extends Thread {
public void run() {
try {
Thread.sleep(1000); // Sleep for 1 second
System.out.println("Thread awake and finished!");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
ThreadExample t = new ThreadExample();
t.start();
t.join(); // Wait for thread to finish
}
}
Synchronization in Multithreading
Thread safety is crucial when many threads access shareable variables or data structures. Threads can conflict without synchronization, resulting in inconsistent results.
Why Synchronization?
Thread interference may cause data inconsistency and exceptions. To avoid this, Java controls thread access to shared resources via synchronization.
Synchronized Methods
The synchronized keyword in Java lets you restrict access to a critical area of code to one thread. An example of method synchronization:
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
Synchronized increment() ensures that only one thread may change the counter value.
Thread Synchronization Example
This example shows thread synchronization. We will increment a shared counter using many threads. Result may be erroneous without synchronization owing to thread interference. Synchronizing the increment function updates the counter securely.
class CounterThread extends Thread {
private Counter counter;
public CounterThread(Counter counter) {
this.counter = counter;
}
public void run() {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread t1 = new CounterThread(counter);
Thread t2 = new CounterThread(counter);
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Final count: " + counter.getCount());
}
}
CounterThread increments the number 1000 times, and synchronized assures a correct count.
Deadlocks and Avoiding Them
Deadlocks happen when two or more threads can not get resources because they are waiting too long. Avoid deadlocks by locking resources in a consistent sequence and without holding multiple locks.
Conclusion
This article covered Java multithreading fundamentals, from thread creation and management to thread safety via synchronization. You can create fast and dependable Java multithreaded programs by knowing thread lifecycle management and synchronization.
Learn more about thread pools and concurrent collections to boost your multithreading abilities. Today's programming requires multithreading to design quicker, more responsive apps for real-world use cases.
299 views