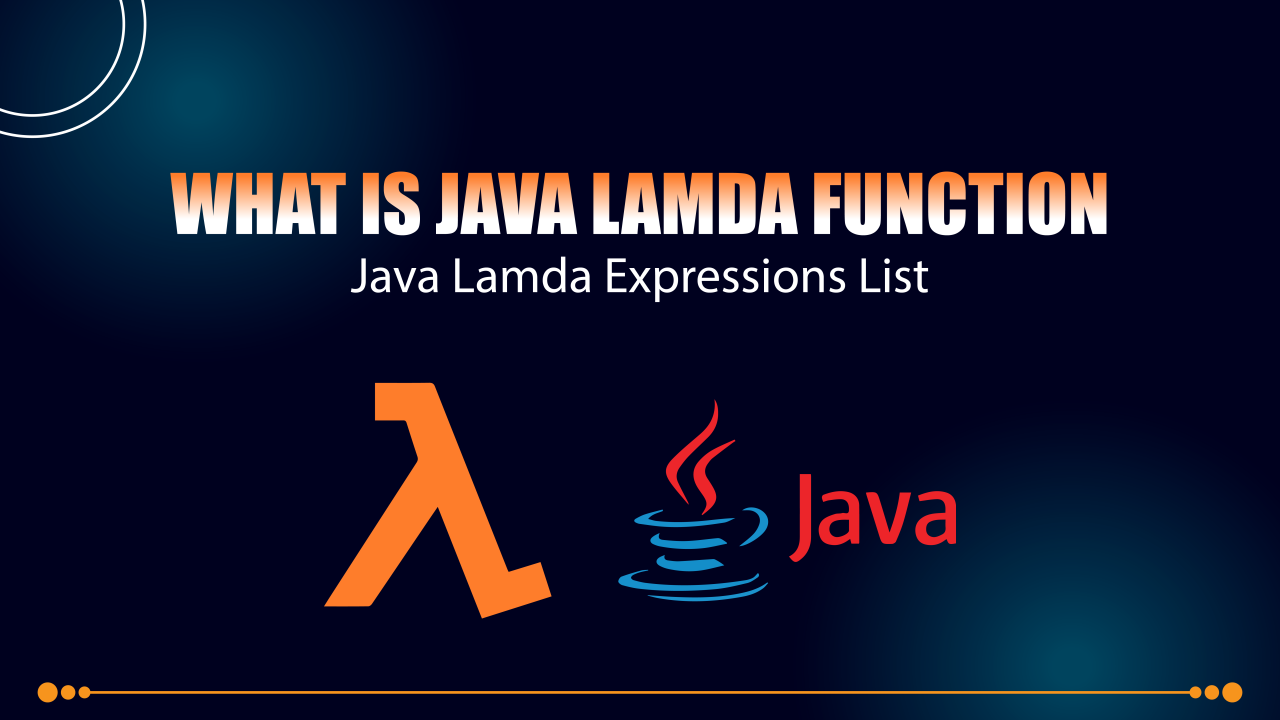
February 04, 2025
Demystifying Lambdas in Java: Writing Cleaner and More Concise Code
Have you ever thought; there has to be an easier way; while seeing repeated code? Java users, particularly before Java 8, have experienced verbose anonymous classes. Though strong, they clutter code and make readability difficult. Lambda expressions are Java's excellent solution for simplified code.
Lambdas, introduced in Java 8, changed functional programming by providing a clear syntax for behavior expression. This blogpost will explain lambdas step-by-step to help you adopt this trendy feature and improve your code.
What Are Lambda Expressions?
A lambda expression is a shorthand for an anonymous function. Think of it as an unnamed method you can apply directly or as an argument.
The syntax is simple:
- (parameters) -> expression
- (parameters) -> { statements; }
For example:
(x) -> x * 2
This function doubles the value of parameter x. Lambdas provide shorter code and behavior flexibility.
Benefits of Using Lambdas
Why do you need to know about lambdas? Here are some strong reasons:
- Conciseness: Replaces verbose unnamed classes, minimizing boilerplate code.
- Improved Readability: Lambdas enable cleaner, simpler to understand code.
- Functional Programming: Lambdas enable functional changes of collections using Java's Stream API.
Lambdas make code current, manageable, and expressive.
Basic Syntax and Examples
Let's explore the syntax with examples:
Single-Parameter Lambda:
x -> x * x;
A function that squares its input.
Multi-Parameter Lambda:
(x, y) -> x + y;
Adds two numbers together.
No-Parameter Lambda:
() -> System.out.println("Hello Lambda!");
Outputs a message to the console.
Comparison: Anonymous Class vs. Lambda
Anonymous Class:
Runnable r = new Runnable() {
public void run() {
System.out.println("Running...");
}
};
Lambda Equivalent:
Runnable r = () -> System.out.println("Running...");
Notice how much cleaner and concise the lambda version is!
Key Functional Interfaces in Java for Lambdas
Functional interfaces with one abstract method and lambda expressions work well together. Java offers some useful ones:
Predicate:
Predicate<Integer> isEven = x -> x % 2 == 0;
Tests whether a number is even.
Function:
Function<String, Integer> length = str -> str.length();
Returns the length of a string.
Consumer:
Consumer<String> print = x -> System.out.println(x);
Prints a string to the console.
Supplier:
Supplier<Double> random = () -> Math.random();
Supplies a random number.
Real-World Use Cases for Lambdas
Lambdas shine in practical scenarios:
Stream API:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4);
numbers.stream().filter(x -> x % 2 == 0).forEach(System.out::println);
Filters and prints even numbers.
Event Handling:
button.setOnAction(e -> System.out.println("Button clicked!"));
Thread Simplification:
new Thread(() -> System.out.println("Thread running")).start();
Lambdas simplify and express coding.
Common Pitfalls and Best Practices
Lambdas are simple and powerful, but its misuse can lead to problems.
- Avoid Overcomplicated Lambdas: Long lambdas might be harder to comprehend than the original code. It is better to use named methods.
// Complex lambda (Avoid)
list.sort((a, b) -> a.length() == b.length() ? a.compareTo(b) : Integer.compare(a.length(), b.length()));
// Simplified with named method
list.sort(this::compareByLengthAndAlphabet);
- Prioritize Readability: Brevity should not compromise readability. Use descriptive variable names and logic breakdown.
- Be Cautious with Side Effects: Avoid modifying shared resources using lambdas in streams or concurrent processes.
- Debugging Tips: Debug complicated lambdas using informative logging or breakpoints.
Conclusion
Lambdas have modernized functional programming in Java by making code more expressive. They reduce boilerplate code, improve readability, and speed processes via functional interfaces and the Stream API. Lambdas help you create clearer code when filtering collections, managing events, or streamlining threads.
As you explore lambdas, you will see their adaptability and efficiency in tackling real-world situations. Take use of this functionality to improve your development process and remain ahead in Java!
490 views