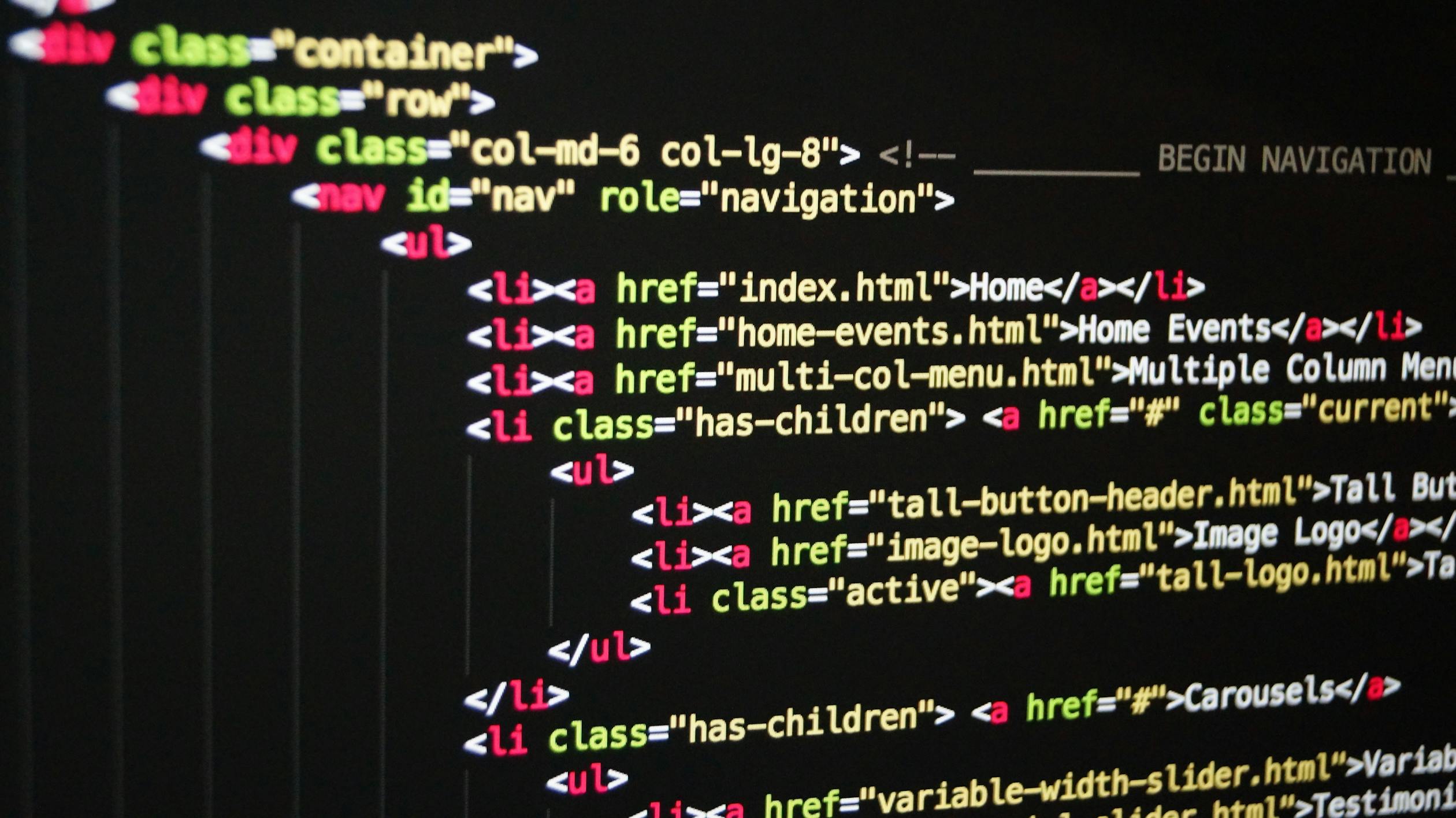
November 28, 2024
Exploring XOR and XNOR: Concepts, Applications, and Use Cases in Software Development
Bitwise operations are frequently overlooked but effective tools for problem-solving in software development. Two such operations, XOR (Exclusive OR) and XNOR (Exclusive NOR), are frequently employed in fields such as algorithms, data validation, and cryptography. XOR and XNOR will be introduced in this post along with their ideas and real-world applications.
Understanding XOR (Exclusive OR)
XOR is a binary operation that takes two input bits and returns 1 if the bits are different and 0 if they are the same. For example:
0 XOR 0
=0
1 XOR 1
=0
0 XOR 1
=1
1 XOR 0
=1
In JavaScript, the XOR operation is represented by the ^
operator. When applied to integers, it performs a bitwise XOR on each corresponding bit.
XOR Use Cases in JavaScript
1. Basic XOR Encryption and Decryption
XOR can provide a simple, symmetric encryption scheme. XORing data with a key scrambles the data, while XORing the encrypted data with the same key restores it to the original.
function xorEncryptDecrypt(data, key) {
return data.split('').map(char => String.fromCharCode(char.charCodeAt(0) ^ key)).join('');
}
const text = "XOR example";
const key = 23;
const encrypted = xorEncryptDecrypt(text, key);
const decrypted = xorEncryptDecrypt(encrypted, key);
console.log("Encrypted:", encrypted); // Encrypted text (scrambled)
console.log("Decrypted:", decrypted); // Original text: "XOR example"
In this example:
xorEncryptDecrypt
function takes adata
string and akey
.- It XORs each characterâs code with the key to scramble the text.
- Calling
xorEncryptDecrypt
again with the same key decrypts the scrambled text back to its original form.
2. Finding the Unique Element in an Array
If an array has elements where each element appears twice, except one unique element, XOR can be used to find this unique element efficiently.
function findUniqueNumber(arr) {
return arr.reduce((acc, num) => acc ^ num, 0);
}
const numbers = [2, 3, 2, 4, 3];
console.log("Unique number:", findUniqueNumber(numbers)); // Output: 4
Explanation:
- XORing a number with itself results in
0
, and XORing with0
has no effect. - By XORing all elements, pairs cancel out, leaving only the unique number.
3. Swapping Variables Without a Temporary Variable
XOR enables swapping values of two variables without needing a temporary variable. This trick can optimize memory usage.
let a = 5;
let b = 7;
a = a ^ b; // Step 1: a becomes 5 XOR 7
b = a ^ b; // Step 2: b becomes (5 XOR 7) XOR 7 => 5
a = a ^ b; // Step 3: a becomes (5 XOR 7) XOR 5 => 7
console.log("a:", a); // Output: 7
console.log("b:", b); // Output: 5
This example:
- Uses XOR operations in sequence to swap the values.
- Avoids any intermediate storage, a useful approach in memory-limited environments.
4. Using XOR as a Fast Equality Checker
If you XOR two numbers and get 0
, they are equal. This trick can be helpful when comparing binary dat
function isEqualBinary(a, b) {
return (a ^ b) === 0;
}
console.log(isEqualBinary(5, 5)); // Output: true
console.log(isEqualBinary(5, 3)); // Output: false
Understanding XNOR (Exclusive NOR)
XNOR is the complement of XOR, returning 1 if both bits are the same and 0 if they are different:
0 XNOR 0
=1
1 XNOR 1
=1
0 XNOR 1
=0
1 XNOR 0
=0
JavaScript doesnât have a direct XNOR operator, but we can simulate it by XORing two values and then applying the NOT operator (~
) to flip the result.
XNOR Use Cases in JavaScript
1. XNOR as an Equality Check
XNOR is often used to check if two binary values are equal. In JavaScript, you can simulate this by checking if the XOR of two values, inverted, gives 1
.
function isEqualXNOR(a, b) {
return ~(a ^ b) === -1; // -1 in two's complement is equivalent to all bits being 1
}
console.log(isEqualXNOR(5, 5)); // Output: true
console.log(isEqualXNOR(5, 3)); // Output: false
2. Using XNOR for Bit Comparison
In some data processing scenarios, you may need to compare bitwise similarity. XNOR gives you a way to do this at the binary level.
function bitwiseSimilarity(a, b) {
const xnorResult = ~(a ^ b) >>> 0;
return xnorResult.toString(2);
}
console.log(bitwiseSimilarity(10, 10)); // Output: '11111111111111111111111111111111' (all bits match)
console.log(bitwiseSimilarity(10, 7)); // Output: '11111111111111111111111111111000' (only 3 least bits differ)
Summary
- XOR (
^
): A bitwise operation that results in1
if bits differ and0
if they are the same. XOR has uses in simple encryption, finding unique elements, swapping values, and checking equality. - XNOR: The complement of XOR, returning
1
if bits are the same and0
if they differ. In JavaScript, XNOR can be implemented with~(a ^ b)
.
Both XOR and XNOR play essential roles in binary operations, allowing for creative, optimized solutions in various algorithms. These examples illustrate how bitwise operations can enhance performance and enable unique solutions to common programming problems in JavaScript.
678 views