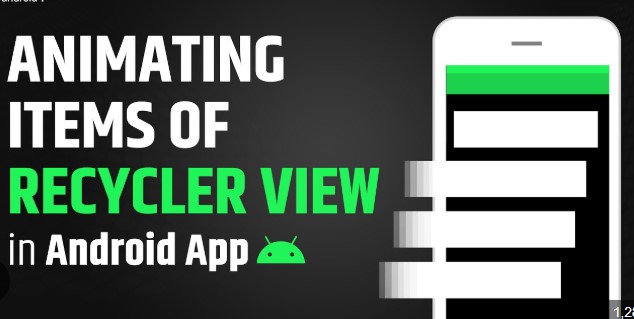
January 24, 2025
Animating RecyclerView Items: A Beginner’s Guide
To make your app's list items stand out, have you considered adding smooth animations? You can make a basic Android app appealing and easy to use by animating RecyclerView objects. Add swipe and fade animations to your RecyclerView to improve its appearance and function with this tutorial.
Understanding RecyclerView
RecyclerView is a flexible Android list view. Reusing view components and allowing movement simplifies large datasets. It shows enormous data sets nicely, and animations help.
- To use RecyclerView, you will need three major things:
- The RecyclerView itself, which holds the list.
- Adapter, which gives the RecyclerView info.
- ViewHolders hold links to the views of each item in the list.
After these basics, you may add animations to your app to make it more engaging.
Setting Up the RecyclerView
Before we start animating, let us quickly put up a RecyclerView in your project. How to:
Add the RecyclerView dependency to your build.gradle file:
implementation 'androidx.recyclerview:recyclerview:1.2.1'
Create a RecyclerView layout in your XML file:
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
Create an Adapter class: Set up the adapter and view holder to fill out your RecyclerView.
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.MyViewHolder> {
private List<String> dataList;
public MyAdapter(List<String> data) {
this.dataList = data;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(android.R.layout.simple_list_item_1, parent, false);
return new MyViewHolder(view);
}
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
holder.textView.setText(dataList.get(position));
}
@Override
public int getItemCount() {
return dataList.size();
}
class MyViewHolder extends RecyclerView.ViewHolder {
TextView textView;
MyViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(android.R.id.text1);
}
}
}
Adding Swipe Animations
In current mobile applications, swipe actions are widespread. Swipe-to-delete and edit increase list user interaction.
Add swipe animations to RecyclerView using ItemTouchHelper. Swipe to add functionality:
Create an ItemTouchHelper Callback:
ItemTouchHelper.SimpleCallback swipeCallback = new ItemTouchHelper.SimpleCallback(0, ItemTouchHelper.LEFT | ItemTouchHelper.RIGHT) {
@Override
public boolean onMove(RecyclerView recyclerView, RecyclerView.ViewHolder viewHolder, RecyclerView.ViewHolder target) {
return false;
}
@Override
public void onSwiped(RecyclerView.ViewHolder viewHolder, int direction) {
int position = viewHolder.getAdapterPosition();
// Handle swipe action (e.g., delete item)
dataList.remove(position);
adapter.notifyItemRemoved(position);
}
};
Attach the ItemTouchHelper to the RecyclerView:
ItemTouchHelper itemTouchHelper = new ItemTouchHelper(swipeCallback);
itemTouchHelper.attachToRecyclerView(recyclerView);
Adding Fade Animations
Fade animations help RecyclerView elements appear and vanish gracefully. ObjectAnimator or AlphaAnimation may fade list items when added, removed, or interacted with.
Add a fade-in effect to RecyclerView items:
Use ObjectAnimator to fade in:
ObjectAnimator fadeIn = ObjectAnimator.ofFloat(holder.itemView, "alpha", 0f, 1f);
fadeIn.setDuration(300); // Duration in milliseconds
fadeIn.start();
Apply fade-out animation when removing an item:
ObjectAnimator fadeOut = ObjectAnimator.ofFloat(holder.itemView, "alpha", 1f, 0f);
fadeOut.setDuration(300);
fadeOut.addListener(new AnimatorListenerAdapter() {
@Override
public void onAnimationEnd(Animator animation) {
super.onAnimationEnd(animation);
// Remove item from the list after fade-out animation
dataList.remove(position);
adapter.notifyItemRemoved(position);
}
});
fadeOut.start();
Conclusion
An animated RecyclerView item helps your app seem better and improves user experience. This tutorial discusses utilizing ItemTouchHelper and ObjectAnimator to animate RecyclerView items swipe and fade. After learning the fundamentals, try scaling or rotation animations to improve your app's interaction.
Once you start utilizing animations in Android development, you will realize how they may make your app more engaging and enjoyable.
234 views